libuav original
Dependents: UAVCAN UAVCAN_Subscriber
storage_backend.hpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_PROTOCOL_DYNAMIC_NODE_ID_SERVER_STORAGE_BACKEND_HPP_INCLUDED 00006 #define UAVCAN_PROTOCOL_DYNAMIC_NODE_ID_SERVER_STORAGE_BACKEND_HPP_INCLUDED 00007 00008 #include <uavcan/build_config.hpp> 00009 #include <uavcan/marshal/types.hpp> 00010 00011 namespace uavcan 00012 { 00013 namespace dynamic_node_id_server 00014 { 00015 /** 00016 * This interface is used by the server to read and write stable storage. 00017 * The storage is represented as a key-value container, where keys and values are ASCII strings up to 32 00018 * characters long, not including the termination byte. Fixed block size allows for absolutely straightforward 00019 * and efficient implementation of storage backends, e.g. based on text files. 00020 * Keys and values may contain only non-whitespace, non-formatting printable characters. 00021 */ 00022 class UAVCAN_EXPORT IStorageBackend 00023 { 00024 public: 00025 /** 00026 * Maximum length of keys and values. One pair takes twice as much space. 00027 */ 00028 enum { MaxStringLength = 32 }; 00029 00030 /** 00031 * It is guaranteed that the server will never require more than this number of key/value pairs. 00032 * Total storage space needed is (MaxKeyValuePairs * MaxStringLength * 2), not including storage overhead. 00033 */ 00034 enum { MaxKeyValuePairs = 512 }; 00035 00036 /** 00037 * This type is used to exchange data chunks with the backend. 00038 * It doesn't use any dynamic memory; please refer to the Array<> class for details. 00039 */ 00040 typedef MakeString<MaxStringLength>::Type String; 00041 00042 /** 00043 * Read one value from the storage. 00044 * If such key does not exist, or if read failed, an empty string will be returned. 00045 * This method should not block for more than 50 ms. 00046 */ 00047 virtual String get(const String& key) const = 0; 00048 00049 /** 00050 * Create or update value for the given key. Empty value should be regarded as a request to delete the key. 00051 * This method should not block for more than 50 ms. 00052 * Failures will be ignored. 00053 */ 00054 virtual void set(const String& key, const String& value) = 0; 00055 00056 virtual ~IStorageBackend() { } 00057 }; 00058 00059 } 00060 } 00061 00062 #endif // Include guard
Generated on Tue Jul 12 2022 17:17:34 by
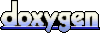