libuav original
Dependents: UAVCAN UAVCAN_Subscriber
publisher.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_NODE_PUBLISHER_HPP_INCLUDED 00006 #define UAVCAN_NODE_PUBLISHER_HPP_INCLUDED 00007 00008 #include <uavcan/node/generic_publisher.hpp> 00009 00010 namespace uavcan 00011 { 00012 /** 00013 * Use this class to publish messages to the bus (broadcast, unicast, or both). 00014 * 00015 * @tparam DataType_ Message data type 00016 */ 00017 template <typename DataType_> 00018 class UAVCAN_EXPORT Publisher : protected GenericPublisher<DataType_, DataType_> 00019 { 00020 typedef GenericPublisher<DataType_, DataType_> BaseType; 00021 00022 public: 00023 typedef DataType_ DataType; ///< Message data type 00024 00025 /** 00026 * @param node Node instance this publisher will be registered with. 00027 * 00028 * @param tx_timeout If CAN frames of this message are not delivered to the bus 00029 * in this amount of time, they will be discarded. Default value 00030 * is good enough for rather high-frequency, high-priority messages. 00031 * 00032 * @param max_transfer_interval Maximum expected transfer interval. It's absolutely safe 00033 * to leave default value here. It just defines how soon the 00034 * Transfer ID tracking objects associated with this message type 00035 * will be garbage collected by the library if it's no longer 00036 * being published. 00037 */ 00038 explicit Publisher(INode& node, MonotonicDuration tx_timeout = getDefaultTxTimeout(), 00039 MonotonicDuration max_transfer_interval = TransferSender::getDefaultMaxTransferInterval()) 00040 : BaseType (node, tx_timeout, max_transfer_interval) 00041 { 00042 #if UAVCAN_DEBUG 00043 UAVCAN_ASSERT(getTxTimeout() == tx_timeout); // Making sure default values are OK 00044 #endif 00045 StaticAssert<DataTypeKind(DataType::DataTypeKind) == DataTypeKindMessage>::check(); 00046 } 00047 00048 /** 00049 * Broadcast the message. 00050 * Returns negative error code. 00051 */ 00052 int broadcast(const DataType& message) 00053 { 00054 return BaseType::publish(message, TransferTypeMessageBroadcast, NodeID::Broadcast); 00055 } 00056 00057 /** 00058 * Warning: You probably don't want to use this method; it's for advanced use cases like 00059 * e.g. network time synchronization. Use the overloaded method with fewer arguments instead. 00060 * This overload allows to explicitly specify Transfer ID. 00061 * Returns negative error code. 00062 */ 00063 int broadcast(const DataType& message, TransferID tid) 00064 { 00065 return BaseType::publish(message, TransferTypeMessageBroadcast, NodeID::Broadcast, tid); 00066 } 00067 00068 static MonotonicDuration getDefaultTxTimeout() { return MonotonicDuration::fromMSec(100); } 00069 00070 /** 00071 * Init method can be called prior first publication, but it's not necessary 00072 * because the publisher can be automatically initialized ad-hoc. 00073 */ 00074 using BaseType::init; 00075 00076 using BaseType::allowAnonymousTransfers; 00077 using BaseType::getTransferSender; 00078 using BaseType::getMinTxTimeout; 00079 using BaseType::getMaxTxTimeout; 00080 using BaseType::getTxTimeout; 00081 using BaseType::setTxTimeout; 00082 using BaseType::getPriority; 00083 using BaseType::setPriority; 00084 using BaseType::getNode; 00085 }; 00086 00087 } 00088 00089 #endif // UAVCAN_NODE_PUBLISHER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:33 by
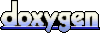