libuav original
Dependents: UAVCAN UAVCAN_Subscriber
file_server.cpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <gtest/gtest.h> 00006 #include <uavcan/protocol/file_server.hpp> 00007 #include "helpers.hpp" 00008 00009 00010 class TestFileServerBackend : public uavcan::IFileServerBackend 00011 { 00012 public: 00013 static const std::string file_name; 00014 static const std::string file_data; 00015 00016 virtual int16_t getInfo(const Path& path, uint64_t& out_size, EntryType& out_type) 00017 { 00018 if (path == file_name) 00019 { 00020 out_size = uint16_t(file_data.length()); 00021 out_type.flags |= EntryType::FLAG_FILE; 00022 out_type.flags |= EntryType::FLAG_READABLE; 00023 return 0; 00024 } 00025 else 00026 { 00027 return Error::NOT_FOUND; 00028 } 00029 } 00030 00031 virtual int16_t read(const Path& path, const uint64_t offset, uint8_t* out_buffer, uint16_t& inout_size) 00032 { 00033 if (path == file_name) 00034 { 00035 if (offset < file_data.length()) 00036 { 00037 inout_size = uint16_t(file_data.length() - offset); 00038 std::memcpy(out_buffer, file_data.c_str() + offset, inout_size); 00039 } 00040 else 00041 { 00042 inout_size = 0; 00043 } 00044 return 0; 00045 } 00046 else 00047 { 00048 return Error::NOT_FOUND; 00049 } 00050 } 00051 }; 00052 00053 const std::string TestFileServerBackend::file_name = "test"; 00054 const std::string TestFileServerBackend::file_data = "123456789"; 00055 00056 TEST(BasicFileServer, Basic) 00057 { 00058 using namespace uavcan::protocol::file; 00059 00060 uavcan::GlobalDataTypeRegistry::instance().reset(); 00061 uavcan::DefaultDataTypeRegistrator<GetInfo> _reg1; 00062 uavcan::DefaultDataTypeRegistrator<Read> _reg2; 00063 00064 InterlinkedTestNodesWithSysClock nodes; 00065 00066 TestFileServerBackend backend; 00067 00068 uavcan::BasicFileServer serv(nodes.a, backend); 00069 std::cout << "sizeof(uavcan::BasicFileServer): " << sizeof(uavcan::BasicFileServer) << std::endl; 00070 00071 ASSERT_LE(0, serv.start()); 00072 00073 /* 00074 * GetInfo, existing file 00075 */ 00076 { 00077 ServiceClientWithCollector<GetInfo> get_info(nodes.b); 00078 00079 GetInfo::Request get_info_req; 00080 get_info_req.path.path = "test"; 00081 00082 ASSERT_LE(0, get_info.call(1, get_info_req)); 00083 nodes.spinBoth(uavcan::MonotonicDuration::fromMSec(10)); 00084 00085 ASSERT_TRUE(get_info.collector.result.get()); 00086 ASSERT_TRUE(get_info.collector.result->isSuccessful()); 00087 00088 ASSERT_EQ(0, get_info.collector.result->getResponse().error.value); 00089 ASSERT_EQ(9, get_info.collector.result->getResponse().size); 00090 ASSERT_EQ(EntryType::FLAG_FILE | EntryType::FLAG_READABLE, 00091 get_info.collector.result->getResponse().entry_type.flags); 00092 } 00093 00094 /* 00095 * Read, existing file 00096 */ 00097 { 00098 ServiceClientWithCollector<Read> read(nodes.b); 00099 00100 Read::Request read_req; 00101 read_req.path.path = "test"; 00102 00103 ASSERT_LE(0, read.call(1, read_req)); 00104 nodes.spinBoth(uavcan::MonotonicDuration::fromMSec(10)); 00105 00106 ASSERT_TRUE(read.collector.result.get()); 00107 ASSERT_TRUE(read.collector.result->isSuccessful()); 00108 00109 ASSERT_EQ("123456789", read.collector.result->getResponse().data); 00110 ASSERT_EQ(0, read.collector.result->getResponse().error.value); 00111 } 00112 } 00113 00114 00115 TEST(FileServer, Basic) 00116 { 00117 using namespace uavcan::protocol::file; 00118 00119 uavcan::GlobalDataTypeRegistry::instance().reset(); 00120 uavcan::DefaultDataTypeRegistrator<GetInfo> _reg1; 00121 uavcan::DefaultDataTypeRegistrator<Read> _reg2; 00122 uavcan::DefaultDataTypeRegistrator<Write> _reg3; 00123 uavcan::DefaultDataTypeRegistrator<Delete> _reg4; 00124 uavcan::DefaultDataTypeRegistrator<GetDirectoryEntryInfo> _reg5; 00125 00126 InterlinkedTestNodesWithSysClock nodes; 00127 00128 TestFileServerBackend backend; 00129 00130 uavcan::FileServer serv(nodes.a, backend); 00131 std::cout << "sizeof(uavcan::FileServer): " << sizeof(uavcan::FileServer) << std::endl; 00132 00133 ASSERT_LE(0, serv.start()); 00134 00135 // TODO TEST 00136 }
Generated on Tue Jul 12 2022 17:17:31 by
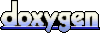