libuav original
Dependents: UAVCAN UAVCAN_Subscriber
dsdl_uavcan_compilability.cpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <gtest/gtest.h> 00006 00007 #include <uavcan/helpers/ostream.hpp> 00008 #include <uavcan/transport/transfer_buffer.hpp> 00009 00010 #include <uavcan/Timestamp.hpp> 00011 #include <uavcan/protocol/param/GetSet.hpp> 00012 #include <uavcan/protocol/GetTransportStats.hpp> 00013 #include <uavcan/protocol/Panic.hpp> 00014 #include <uavcan/protocol/RestartNode.hpp> 00015 #include <uavcan/protocol/GlobalTimeSync.hpp> 00016 #include <uavcan/protocol/DataTypeKind.hpp> 00017 #include <uavcan/protocol/GetDataTypeInfo.hpp> 00018 #include <uavcan/protocol/NodeStatus.hpp> 00019 #include <uavcan/protocol/GetNodeInfo.hpp> 00020 #include <uavcan/protocol/debug/LogMessage.hpp> 00021 #include <uavcan/protocol/debug/KeyValue.hpp> 00022 00023 #include <root_ns_a/Deep.hpp> 00024 #include <root_ns_a/UnionTest.hpp> 00025 00026 template <typename T> 00027 static bool validateYaml(const T& obj, const std::string& reference) 00028 { 00029 uavcan::OStream::instance() << "Validating YAML:\n" << obj << "\n" << uavcan::OStream::endl; 00030 00031 std::ostringstream os; 00032 os << obj; 00033 if (os.str() == reference) 00034 { 00035 return true; 00036 } 00037 else 00038 { 00039 std::cout << "INVALID YAML:\n" 00040 << "EXPECTED:\n" 00041 << "===\n" 00042 << reference 00043 << "\n===\n" 00044 << "ACTUAL:\n" 00045 << "\n===\n" 00046 << os.str() 00047 << "\n===\n" << std::endl; 00048 return false; 00049 } 00050 } 00051 00052 TEST(Dsdl, Streaming) 00053 { 00054 EXPECT_TRUE(validateYaml(uavcan::protocol::GetNodeInfo::Response(), 00055 "status: \n" 00056 " uptime_sec: 0\n" 00057 " health: 0\n" 00058 " mode: 0\n" 00059 " sub_mode: 0\n" 00060 " vendor_specific_status_code: 0\n" 00061 "software_version: \n" 00062 " major: 0\n" 00063 " minor: 0\n" 00064 " optional_field_flags: 0\n" 00065 " vcs_commit: 0\n" 00066 " image_crc: 0\n" 00067 "hardware_version: \n" 00068 " major: 0\n" 00069 " minor: 0\n" 00070 " unique_id: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]\n" 00071 " certificate_of_authenticity: \"\"\n" 00072 "name: \"\"")); 00073 00074 root_ns_a::Deep ps; 00075 ps.a.resize(1); 00076 EXPECT_TRUE(validateYaml(ps, 00077 "c: 0\n" 00078 "str: \"\"\n" 00079 "a: \n" 00080 " - \n" 00081 " scalar: 0\n" 00082 " vector: \n" 00083 " - \n" 00084 " vector: [0, 0]\n" 00085 " bools: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]\n" 00086 " - \n" 00087 " vector: [0, 0]\n" 00088 " bools: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]\n" 00089 "b: \n" 00090 " - \n" 00091 " vector: [0, 0]\n" 00092 " bools: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]\n" 00093 " - \n" 00094 " vector: [0, 0]\n" 00095 " bools: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]")); 00096 } 00097 00098 00099 template <typename T> 00100 static bool encodeDecodeValidate(const T& obj, const std::string& reference_bit_string) 00101 { 00102 uavcan::StaticTransferBuffer<256> buf; 00103 00104 { 00105 uavcan::BitStream bits(buf); 00106 uavcan::ScalarCodec codec(bits); 00107 /* 00108 * Coding 00109 */ 00110 if (0 > T::encode(obj, codec)) 00111 { 00112 std::cout << "Failed to encode" << std::endl; 00113 return false; 00114 } 00115 00116 /* 00117 * Validating the encoded bitstream 00118 */ 00119 const std::string result = bits.toString(); 00120 if (result != reference_bit_string) 00121 { 00122 std::cout << "ENCODED VALUE DOESN'T MATCH THE REFERENCE:\nEXPECTED:\n" 00123 << reference_bit_string << "\nACTUAL:\n" 00124 << result << std::endl; 00125 return false; 00126 } 00127 } 00128 00129 /* 00130 * Decoding back and comparing 00131 */ 00132 uavcan::BitStream bits(buf); 00133 uavcan::ScalarCodec codec(bits); 00134 00135 T decoded; 00136 00137 if (0 > T::decode(decoded, codec)) 00138 { 00139 std::cout << "Failed to decode" << std::endl; 00140 return false; 00141 } 00142 00143 if (!decoded.isClose(obj)) 00144 { 00145 std::cout << "DECODED OBJECT DOESN'T MATCH THE REFERENCE:\nEXPECTED:\n" 00146 << obj << "\nACTUAL:\n" 00147 << decoded << std::endl; 00148 return false; 00149 } 00150 return true; 00151 } 00152 00153 00154 TEST(Dsdl, Union) 00155 { 00156 using root_ns_a::UnionTest; 00157 using root_ns_a::NestedInUnion; 00158 00159 ASSERT_EQ(3, UnionTest::MinBitLen); 00160 ASSERT_EQ(16, UnionTest::MaxBitLen); 00161 ASSERT_EQ(13, NestedInUnion::MinBitLen); 00162 ASSERT_EQ(13, NestedInUnion::MaxBitLen); 00163 00164 UnionTest s; 00165 00166 EXPECT_TRUE(validateYaml(s, "z: ")); 00167 encodeDecodeValidate(s, "00000000"); 00168 00169 s.to<UnionTest::Tag::a>() = 16; 00170 EXPECT_TRUE(validateYaml(s, "a: 16")); 00171 EXPECT_TRUE(encodeDecodeValidate(s, "00110000")); 00172 00173 s.to<UnionTest::Tag::b>() = 31; 00174 EXPECT_TRUE(validateYaml(s, "b: 31")); 00175 EXPECT_TRUE(encodeDecodeValidate(s, "01011111")); 00176 00177 s.to<UnionTest::Tag::c>() = 256; 00178 EXPECT_TRUE(validateYaml(s, "c: 256")); 00179 EXPECT_TRUE(encodeDecodeValidate(s, "01100000 00000001")); 00180 00181 s.to<UnionTest::Tag::d>().push_back(true); 00182 s.to<UnionTest::Tag::d>().push_back(false); 00183 s.to<UnionTest::Tag::d>().push_back(true); 00184 s.to<UnionTest::Tag::d>().push_back(true); 00185 s.to<UnionTest::Tag::d>().push_back(false); 00186 s.to<UnionTest::Tag::d>().push_back(false); 00187 s.to<UnionTest::Tag::d>().push_back(true); 00188 s.to<UnionTest::Tag::d>().push_back(true); 00189 s.to<UnionTest::Tag::d>().push_back(true); 00190 ASSERT_EQ(9, s.to<UnionTest::Tag::d>().size()); 00191 EXPECT_TRUE(validateYaml(s, "d: [1, 0, 1, 1, 0, 0, 1, 1, 1]")); 00192 EXPECT_TRUE(encodeDecodeValidate(s, "10010011 01100111")); 00193 00194 s.to<UnionTest::Tag::e>().array[0] = 0; 00195 s.to<UnionTest::Tag::e>().array[1] = 1; 00196 s.to<UnionTest::Tag::e>().array[2] = 2; 00197 s.to<UnionTest::Tag::e>().array[3] = 3; 00198 EXPECT_TRUE(validateYaml(s, "e: \n array: [0, 1, 2, 3]")); 00199 EXPECT_TRUE(encodeDecodeValidate(s, "10100000 11011000")); 00200 } 00201 00202 00203 TEST(Dsdl, ParamGetSetRequestUnion) 00204 { 00205 uavcan::protocol::param::GetSet::Request req; 00206 00207 req.index = 8191; 00208 req.name = "123"; // 49, 50, 51 // 00110001, 00110010, 00110011 00209 EXPECT_TRUE(encodeDecodeValidate(req, "11111111 11111000 00110001 00110010 00110011")); 00210 00211 req.value.to<uavcan::protocol::param::Value::Tag::string_value>() = "abc"; // 01100001, 01100010, 01100011 00212 EXPECT_TRUE(encodeDecodeValidate(req, 00213 "11111111 11111100 " // Index, Union tag 00214 "00000011 " // Array length 00215 "01100001 01100010 01100011 " // Payload 00216 "00110001 00110010 00110011")); // Name 00217 00218 EXPECT_TRUE(validateYaml(req, 00219 "index: 8191\n" 00220 "value: \n" 00221 " string_value: \"abc\"\n" 00222 "name: \"123\"")); 00223 00224 req.value.to<uavcan::protocol::param::Value::Tag::integer_value>() = 1; 00225 EXPECT_TRUE(encodeDecodeValidate(req, 00226 "11111111 11111001 " // Index, Union tag 00227 "00000001 00000000 00000000 00000000 00000000 00000000 00000000 00000000 " // Payload 00228 "00110001 00110010 00110011")); // Name 00229 } 00230 00231 00232 TEST(Dsdl, ParamGetSetResponseUnion) 00233 { 00234 uavcan::protocol::param::GetSet::Response res; 00235 00236 res.value.to<uavcan::protocol::param::Value::Tag::string_value>() = "abc"; 00237 res.default_value.to<uavcan::protocol::param::Value::Tag::string_value>(); // Empty 00238 res.name = "123"; 00239 EXPECT_TRUE(encodeDecodeValidate(res, 00240 "00000100 " // Value union tag 00241 "00000011 " // Value array length 00242 "01100001 01100010 01100011 " // Value array payload 00243 "00000100 " // Default union tag 00244 "00000000 " // Default array length 00245 "00000000 " // Max value tag 00246 "00000000 " // Min value tag 00247 "00110001 00110010 00110011")); // Name 00248 00249 res.value.to<uavcan::protocol::param::Value::Tag::boolean_value>() = true; 00250 res.default_value.to<uavcan::protocol::param::Value::Tag::boolean_value>(); // False 00251 res.name = "123"; 00252 EXPECT_TRUE(encodeDecodeValidate(res, 00253 "00000011 " // Value union tag 00254 "00000001 " // Value 00255 "00000011 " // Default union tag 00256 "00000000 " // Default value 00257 "00000000 " // Max value tag 00258 "00000000 " // Min value tag 00259 "00110001 00110010 00110011")); // Name 00260 }
Generated on Tue Jul 12 2022 17:17:31 by
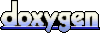