libuav original
Dependents: UAVCAN UAVCAN_Subscriber
SocketCanIface Class Reference
Single SocketCAN socket interface. More...
#include <socketcan.hpp>
Inherits uavcan::ICanIface.
Inherited by SocketCanDriver::IfaceWrapper.
Public Member Functions | |
SocketCanIface (const SystemClock &clock, int socket_fd, int max_frames_in_socket_tx_queue=2) | |
Takes ownership of socket's file descriptor. | |
virtual | ~SocketCanIface () |
Socket file descriptor will be closed. | |
std::int16_t | send (const uavcan::CanFrame &frame, const uavcan::MonotonicTime tx_deadline, const uavcan::CanIOFlags flags) override |
Assumes that the socket is writeable. | |
std::int16_t | receive (uavcan::CanFrame &out_frame, uavcan::MonotonicTime &out_ts_monotonic, uavcan::UtcTime &out_ts_utc, uavcan::CanIOFlags &out_flags) override |
Will read the socket only if RX queue is empty. | |
void | poll (bool read, bool write) |
Performs socket read/write. | |
std::uint16_t | getNumFilters () const override |
Number of available hardware filters. | |
std::uint64_t | getErrorCount () const override |
Returns total number of errors of each kind detected since the object was created. | |
const | decltype (errors_)&getErrors() const |
Returns number of errors of each kind in a map. | |
virtual int16_t | configureFilters (const CanFilterConfig *filter_configs, uint16_t num_configs)=0 |
Configure the hardware CAN filters. | |
Static Public Member Functions | |
static int | openSocket (const std::string &iface_name) |
Open and configure a CAN socket on iface specified by name. | |
Static Public Attributes | |
static constexpr unsigned | NumFilters = 8 |
SocketCAN emulates the CAN filters in software, so the number of filters is virtually unlimited. |
Detailed Description
Single SocketCAN socket interface.
SocketCAN socket adapter maintains TX and RX queues in user space. At any moment socket's buffer contains no more than 'max_frames_in_socket_tx_queue_' TX frames, rest is waiting in the user space TX queue; when the socket produces loopback for the previously sent TX frame the next frame from the user space TX queue will be sent into the socket.
This approach allows to properly maintain TX timeouts (http://stackoverflow.com/questions/19633015/). TX timestamping is implemented by means of reading RX timestamps of loopback frames (see "TX timestamping" on linux-can mailing list, http://permalink.gmane.org/gmane.linux.can/5322).
Note that if max_frames_in_socket_tx_queue_ is greater than one, frame reordering may occur (depending on the unrderlying logic).
This class is too complex and needs to be refactored later. At least, basic socket IO and configuration should be extracted into a different class.
Definition at line 60 of file socketcan.hpp.
Constructor & Destructor Documentation
SocketCanIface | ( | const SystemClock & | clock, |
int | socket_fd, | ||
int | max_frames_in_socket_tx_queue = 2 |
||
) |
Takes ownership of socket's file descriptor.
max_frames_in_socket_tx_queue See a note in the class comment.
Definition at line 372 of file socketcan.hpp.
virtual ~SocketCanIface | ( | ) | [virtual] |
Socket file descriptor will be closed.
Definition at line 383 of file socketcan.hpp.
Member Function Documentation
virtual int16_t configureFilters | ( | const CanFilterConfig * | filter_configs, |
uint16_t | num_configs | ||
) | [pure virtual, inherited] |
Configure the hardware CAN filters.
- Returns:
- 0 = success, negative for error.
Implemented in CanDriver.
const decltype | ( | errors_ | ) | const |
Returns number of errors of each kind in a map.
Definition at line 509 of file socketcan.hpp.
std::uint64_t getErrorCount | ( | ) | const [override, virtual] |
Returns total number of errors of each kind detected since the object was created.
Implements ICanIface.
Definition at line 499 of file socketcan.hpp.
std::uint16_t getNumFilters | ( | ) | const [override, virtual] |
Number of available hardware filters.
Implements ICanIface.
Definition at line 493 of file socketcan.hpp.
static int openSocket | ( | const std::string & | iface_name ) | [static] |
Open and configure a CAN socket on iface specified by name.
- Parameters:
-
iface_name String containing iface name, e.g. "can0", "vcan1", "slcan0"
- Returns:
- Socket descriptor or negative number on error.
Definition at line 518 of file socketcan.hpp.
void poll | ( | bool | read, |
bool | write | ||
) |
Performs socket read/write.
- Parameters:
-
read Socket is readable write Socket is writeable
Definition at line 433 of file socketcan.hpp.
std::int16_t receive | ( | uavcan::CanFrame & | out_frame, |
uavcan::MonotonicTime & | out_ts_monotonic, | ||
uavcan::UtcTime & | out_ts_utc, | ||
uavcan::CanIOFlags & | out_flags | ||
) | [override, virtual] |
Will read the socket only if RX queue is empty.
Normally, poll() needs to be executed first.
Implements ICanIface.
Definition at line 406 of file socketcan.hpp.
std::int16_t send | ( | const uavcan::CanFrame & | frame, |
const uavcan::MonotonicTime | tx_deadline, | ||
const uavcan::CanIOFlags | flags | ||
) | [override, virtual] |
Assumes that the socket is writeable.
Implements ICanIface.
Definition at line 392 of file socketcan.hpp.
Field Documentation
constexpr unsigned NumFilters = 8 [static] |
SocketCAN emulates the CAN filters in software, so the number of filters is virtually unlimited.
This method returns a constant value.
Definition at line 492 of file socketcan.hpp.
Generated on Tue Jul 12 2022 17:17:38 by
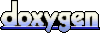