libuav original
Dependents: UAVCAN UAVCAN_Subscriber
Multiset< T > Class Template Reference
Slow but memory efficient unordered multiset. More...
#include <multiset.hpp>
Inherits uavcan::Noncopyable.
Public Member Functions | |
T * | emplace () |
Creates one item in-place and returns a pointer to it. | |
template<typename Predicate > | |
void | removeAllWhere (Predicate predicate) |
Removes entries where the predicate returns true. | |
template<typename Predicate > | |
T * | find (Predicate predicate) |
Returns first entry where the predicate returns true. | |
template<typename Operator > | |
void | forEach (Operator oper) |
Calls Operator for each item of the set. | |
T * | getByIndex (unsigned index) |
Returns an item located at the specified position from the beginning. | |
bool | isEmpty () const |
Complexity is O(1). | |
unsigned | getSize () const |
Counts number of items stored. |
Detailed Description
template<typename T>
class uavcan::Multiset< T >
Slow but memory efficient unordered multiset.
Unlike Map<>, this container does not move objects, so they don't have to be copyable.
Items will be allocated in the node's memory pool.
Definition at line 29 of file multiset.hpp.
Member Function Documentation
T* emplace | ( | ) |
Creates one item in-place and returns a pointer to it.
If creation fails due to lack of memory, UAVCAN_NULLPTR will be returned. Complexity is O(N).
Definition at line 205 of file multiset.hpp.
T * find | ( | Predicate | predicate ) |
Returns first entry where the predicate returns true.
Predicate prototype: bool (const T& item)
Definition at line 437 of file multiset.hpp.
void forEach | ( | Operator | oper ) |
Calls Operator for each item of the set.
Operator prototype: void (T& item) void (const T& item) - const overload
Definition at line 294 of file multiset.hpp.
T* getByIndex | ( | unsigned | index ) |
Returns an item located at the specified position from the beginning.
Note that addition and removal operations invalidate indices. If index is greater than or equal the number of items, null pointer will be returned. Complexity is O(N).
Definition at line 313 of file multiset.hpp.
unsigned getSize | ( | ) | const |
Counts number of items stored.
Best case complexity is O(N).
Definition at line 461 of file multiset.hpp.
bool isEmpty | ( | ) | const |
Complexity is O(1).
Definition at line 327 of file multiset.hpp.
void removeAllWhere | ( | Predicate | predicate ) |
Removes entries where the predicate returns true.
Predicate prototype: bool (T& item)
Definition at line 262 of file multiset.hpp.
Generated on Tue Jul 12 2022 17:17:37 by
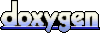