libuav original
Dependents: UAVCAN UAVCAN_Subscriber
Map< Key, Value > Class Template Reference
Slow but memory efficient KV container. More...
#include <map.hpp>
Inherits uavcan::Noncopyable.
Public Member Functions | |
Value * | access (const Key &key) |
Returns null pointer if there's no such entry. | |
Value * | insert (const Key &key, const Value &value) |
If entry with the same key already exists, it will be replaced. | |
void | remove (const Key &key) |
Does nothing if there's no such entry. | |
template<typename Predicate > | |
void | removeAllWhere (Predicate predicate) |
Removes entries where the predicate returns true. | |
template<typename Predicate > | |
const Key * | find (Predicate predicate) const |
Returns first entry where the predicate returns true. | |
void | clear () |
Removes all items. | |
KVPair * | getByIndex (unsigned index) |
Returns a key-value pair located at the specified position from the beginning. | |
bool | isEmpty () const |
Complexity is O(1). | |
unsigned | getSize () const |
Complexity is O(N). |
Detailed Description
template<typename Key, typename Value>
class uavcan::Map< Key, Value >
Slow but memory efficient KV container.
KV pairs will be allocated in the node's memory pool.
Please be aware that this container does not perform any speed optimizations to minimize memory footprint, so the complexity of most operations is O(N).
Type requirements: Both key and value must be copyable, assignable and default constructible. Key must implement a comparison operator. Key's default constructor must initialize the object into invalid state. Size of Key + Value + padding must not exceed MemPoolBlockSize.
Definition at line 33 of file map.hpp.
Member Function Documentation
Value * access | ( | const Key & | key ) |
const Key * find | ( | Predicate | predicate ) | const |
Map< Key, Value >::KVPair * getByIndex | ( | unsigned | index ) |
Value * insert | ( | const Key & | key, |
const Value & | value | ||
) |
void remove | ( | const Key & | key ) |
Generated on Tue Jul 12 2022 17:17:37 by
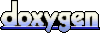