libuav original
Dependents: UAVCAN UAVCAN_Subscriber
Logger Class Reference
Node logging convenience class. More...
#include <logger.hpp>
Public Member Functions | |
int | init (const TransferPriority priority=TransferPriority::Lowest) |
Initializes the logger, does not perform any network activity. | |
int | log (const protocol::debug::LogMessage &message) |
Logs one message. | |
LogLevel | getLevel () const |
Severity filter for UAVCAN broadcasting. | |
ILogSink * | getExternalSink () const |
External log sink allows the application to install a hook on the logger output. | |
MonotonicDuration | getTxTimeout () const |
Log message broadcast transmission timeout. | |
template<typename... Args> | |
int | log (LogLevel level, const char *source, const char *format, Args...args) UAVCAN_NOEXCEPT |
Helper methods for various severity levels and with formatting support. | |
Static Public Member Functions | |
static LogLevel | getLogLevelAboveAll () |
This value is higher than any valid severity value. |
Detailed Description
Node logging convenience class.
This class is based on the standard UAVCAN message type for logging - uavcan.protocol.debug.LogMessage.
Provides logging methods of different severity; implements two sinks for the log messages:
- Broadcast via the UAVCAN bus;
- Sink into the application via ILogSink.
For each sink an individual severity threshold filter can be configured.
Definition at line 58 of file logger.hpp.
Member Function Documentation
ILogSink* getExternalSink | ( | ) | const |
External log sink allows the application to install a hook on the logger output.
This can be used for application-wide logging. Null pointer means that there's no log sink (can be used to remove it). By default there's no log sink.
Definition at line 147 of file logger.hpp.
LogLevel getLevel | ( | ) | const |
Severity filter for UAVCAN broadcasting.
Log message will be broadcasted via the UAVCAN network only if its severity is >= getLevel(). This does not affect the external sink. Default level is ERROR.
Definition at line 138 of file logger.hpp.
static LogLevel getLogLevelAboveAll | ( | ) | [static] |
This value is higher than any valid severity value.
Use it to completely suppress the output.
Definition at line 67 of file logger.hpp.
MonotonicDuration getTxTimeout | ( | ) | const |
Log message broadcast transmission timeout.
The default value should be acceptable for any use case.
Definition at line 154 of file logger.hpp.
int init | ( | const TransferPriority | priority = TransferPriority::Lowest ) |
Initializes the logger, does not perform any network activity.
Must be called once before use. Returns negative error code.
Definition at line 97 of file logger.hpp.
int log | ( | LogLevel | level, |
const char * | source, | ||
const char * | format, | ||
Args... | args | ||
) |
Helper methods for various severity levels and with formatting support.
These methods build a formatted log message and pass it into the method log().
Format string usage is a bit unusual: use "%*" for any argument type, use "%%" to print a percent character. No other formating options are supported. Insufficient/extra arguments are ignored.
Example format string: "What do you get if you %* %* by %*? %*. Extra arguments: %* %* %%" ...with the following arguments: "multiply", 6, 9.0F 4.2e1 ...will likely produce this (floating point representation is platform dependent): "What do you get if you multiply 6 by 9.000000? 42.000000. Extra arguments: %* %* %"
Formatting is not supported in C++03 mode.
Definition at line 257 of file logger.hpp.
int log | ( | const protocol::debug::LogMessage & | message ) |
Logs one message.
Please consider using helper methods instead of this one.
The message will be broadcasted via the UAVCAN bus if the severity level of the message is >= severity level of the logger.
The message will be reported into the external log sink if the external sink is installed and the severity level of the message is >= severity level of the external sink.
Returns negative error code.
Definition at line 118 of file logger.hpp.
Generated on Tue Jul 12 2022 17:17:37 by
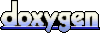