libuav original
Dependents: UAVCAN UAVCAN_Subscriber
HeapBasedPoolAllocator< BlockSize, RaiiSynchronizer > Class Template Reference
A special-purpose implementation of a pool allocator that keeps the pool in the heap using malloc()/free(). More...
#include <heap_based_pool_allocator.hpp>
Inherits uavcan::IPoolAllocator, and uavcan::Noncopyable.
Public Member Functions | |
HeapBasedPoolAllocator (uint16_t block_capacity_soft_limit, uint16_t block_capacity_hard_limit=0) | |
The allocator initializes with empty reserve, so first allocations will be served from heap. | |
~HeapBasedPoolAllocator () | |
The destructor de-allocates all blocks that are currently in the reserve. | |
virtual void * | allocate (std::size_t size) |
Takes a block from the reserve, unless it's empty. | |
virtual void | deallocate (const void *ptr) |
Puts the block back to reserve. | |
virtual uint16_t | getBlockCapacity () const |
The soft limit. | |
uint16_t | getBlockCapacityHardLimit () const |
The hard limit. | |
void | shrink () |
Frees all blocks that are not in use at the moment. | |
uint16_t | getNumAllocatedBlocks () const |
Number of blocks that are currently in use by the application. | |
uint16_t | getNumReservedBlocks () const |
Number of blocks that are acquired from the heap. |
Detailed Description
template<std::size_t BlockSize, typename RaiiSynchronizer = char>
class uavcan::HeapBasedPoolAllocator< BlockSize, RaiiSynchronizer >
A special-purpose implementation of a pool allocator that keeps the pool in the heap using malloc()/free().
The pool grows dynamically, ad-hoc, thus using as little memory as possible.
Allocated blocks will not be freed back automatically, but there are two ways to force their deallocation:
- Call shrink() - this method frees all blocks that are unused at the moment.
- Destroy the object - the desctructor calls shrink().
The pool can be limited in growth with hard and soft limits. The soft limit defines the value that will be reported via IPoolAllocator::getBlockCapacity(). The hard limit defines the maximum number of blocks that can be allocated from heap. Typically, the hard limit should be equal or greater than the soft limit.
The allocator can be made thread-safe (optional) by means of providing a RAII-lock type via the second template argument. The allocator uses the lock only to access the shared state, therefore critical sections are only a few cycles long, which implies that it should be acceptable to use hardware IRQ disabling instead of a mutex for performance reasons. For example, an IRQ-based RAII-lock type can be implemented as follows: struct RaiiSynchronizer { RaiiSynchronizer() { __disable_irq(); } ~RaiiSynchronizer() { __enable_irq(); } };
Definition at line 40 of file heap_based_pool_allocator.hpp.
Constructor & Destructor Documentation
HeapBasedPoolAllocator | ( | uint16_t | block_capacity_soft_limit, |
uint16_t | block_capacity_hard_limit = 0 |
||
) |
The allocator initializes with empty reserve, so first allocations will be served from heap.
- Parameters:
-
block_capacity_soft_limit Block capacity that will be reported via getBlockCapacity(). block_capacity_hard_limit Real block capacity limit; the number of allocated blocks will never exceed this value. Hard limit should be higher than soft limit. Default value is two times the soft limit.
Definition at line 70 of file heap_based_pool_allocator.hpp.
The destructor de-allocates all blocks that are currently in the reserve.
BLOCKS THAT ARE CURRENTLY HELD BY THE APPLICATION WILL LEAK.
Definition at line 85 of file heap_based_pool_allocator.hpp.
Member Function Documentation
virtual void* allocate | ( | std::size_t | size ) | [virtual] |
Takes a block from the reserve, unless it's empty.
In the latter case, allocates a new block in the heap.
Implements IPoolAllocator.
Definition at line 100 of file heap_based_pool_allocator.hpp.
virtual void deallocate | ( | const void * | ptr ) | [virtual] |
Puts the block back to reserve.
The block will not be free()d automatically; see shrink().
Implements IPoolAllocator.
Definition at line 143 of file heap_based_pool_allocator.hpp.
virtual uint16_t getBlockCapacity | ( | ) | const [virtual] |
The soft limit.
Implements IPoolAllocator.
Definition at line 161 of file heap_based_pool_allocator.hpp.
uint16_t getBlockCapacityHardLimit | ( | ) | const |
The hard limit.
Definition at line 166 of file heap_based_pool_allocator.hpp.
uint16_t getNumAllocatedBlocks | ( | ) | const |
Number of blocks that are currently in use by the application.
Definition at line 200 of file heap_based_pool_allocator.hpp.
uint16_t getNumReservedBlocks | ( | ) | const |
Number of blocks that are acquired from the heap.
Definition at line 210 of file heap_based_pool_allocator.hpp.
void shrink | ( | ) |
Frees all blocks that are not in use at the moment.
Post-condition is getNumAllocatedBlocks() == getNumReservedBlocks().
Definition at line 172 of file heap_based_pool_allocator.hpp.
Generated on Tue Jul 12 2022 17:17:37 by
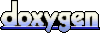