libuav original
Dependents: UAVCAN UAVCAN_Subscriber
allocation_request_manager.cpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <gtest/gtest.h> 00006 #include <uavcan/protocol/dynamic_node_id_server/allocation_request_manager.hpp> 00007 #include <uavcan/protocol/dynamic_node_id_client.hpp> 00008 #include "event_tracer.hpp" 00009 #include "../helpers.hpp" 00010 00011 00012 using uavcan::dynamic_node_id_server::UniqueID; 00013 00014 class AllocationRequestHandler : public uavcan::dynamic_node_id_server::IAllocationRequestHandler 00015 { 00016 std::vector<std::pair<UniqueID, uavcan::NodeID> > requests_; 00017 00018 public: 00019 bool can_followup; 00020 00021 AllocationRequestHandler() : can_followup(false) { } 00022 00023 virtual void handleAllocationRequest(const UniqueID& unique_id, uavcan::NodeID preferred_node_id) 00024 { 00025 requests_.push_back(std::pair<UniqueID, uavcan::NodeID>(unique_id, preferred_node_id)); 00026 } 00027 00028 virtual bool canPublishFollowupAllocationResponse() const 00029 { 00030 return can_followup; 00031 } 00032 00033 bool matchAndPopLastRequest(const UniqueID& unique_id, uavcan::NodeID preferred_node_id) 00034 { 00035 if (requests_.empty()) 00036 { 00037 std::cout << "No pending requests" << std::endl; 00038 return false; 00039 } 00040 00041 const std::pair<UniqueID, uavcan::NodeID> pair = requests_.at(requests_.size() - 1U); 00042 requests_.pop_back(); 00043 00044 if (pair.first != unique_id) 00045 { 00046 std::cout << "Unique ID mismatch" << std::endl; 00047 return false; 00048 } 00049 00050 if (pair.second != preferred_node_id) 00051 { 00052 std::cout << "Node ID mismatch (" << pair.second.get() << ", " << preferred_node_id.get() << ")" 00053 << std::endl; 00054 return false; 00055 } 00056 00057 return true; 00058 } 00059 00060 void reset() { requests_.clear(); } 00061 }; 00062 00063 00064 TEST(dynamic_node_id_server_AllocationRequestManager, Basic) 00065 { 00066 using namespace uavcan::protocol::dynamic_node_id; 00067 using namespace uavcan::protocol::dynamic_node_id::server; 00068 using namespace uavcan::dynamic_node_id_server; 00069 00070 uavcan::GlobalDataTypeRegistry::instance().reset(); 00071 uavcan::DefaultDataTypeRegistrator<Allocation> _reg1; 00072 00073 // Node A is Allocator, Node B is Allocatee 00074 InterlinkedTestNodesWithSysClock nodes(uavcan::NodeID(10), uavcan::NodeID::Broadcast); 00075 00076 uavcan::DynamicNodeIDClient client(nodes.b); 00077 00078 /* 00079 * Client initialization 00080 */ 00081 uavcan::protocol::HardwareVersion::FieldTypes::unique_id unique_id; 00082 for (uavcan::uint8_t i = 0; i < unique_id.size(); i++) 00083 { 00084 unique_id[i] = i; 00085 } 00086 const uavcan::NodeID PreferredNodeID = 42; 00087 ASSERT_LE(0, client.start(unique_id, PreferredNodeID)); 00088 00089 /* 00090 * Request manager initialization 00091 */ 00092 EventTracer tracer; 00093 AllocationRequestHandler handler; 00094 handler.can_followup = true; 00095 00096 AllocationRequestManager manager(nodes.a, tracer, handler); 00097 00098 ASSERT_LE(0, manager.init(uavcan::TransferPriority::OneHigherThanLowest)); 00099 00100 /* 00101 * Allocation 00102 */ 00103 nodes.spinBoth(uavcan::MonotonicDuration::fromMSec(2000)); 00104 00105 ASSERT_TRUE(handler.matchAndPopLastRequest(unique_id, PreferredNodeID)); 00106 00107 ASSERT_LE(0, manager.broadcastAllocationResponse(unique_id, PreferredNodeID)); 00108 00109 nodes.spinBoth(uavcan::MonotonicDuration::fromMSec(100)); 00110 00111 /* 00112 * Checking the client 00113 */ 00114 ASSERT_TRUE(client.isAllocationComplete()); 00115 00116 ASSERT_EQ(PreferredNodeID, client.getAllocatedNodeID()); 00117 }
Generated on Tue Jul 12 2022 17:17:30 by
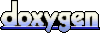