
Mandelbrot viewer for SPI TFT touchscreen
Dependencies: SPI_TFT TFT_fonts Touch_tft mbed
main.cpp
00001 /* Mandelbrot for mbed - April 2013 00002 * Uses Peter Drescher's library for Mikroelektronika's TFT-Proto screen, an inexpensive SPI-driven QVGA touch panel 00003 * Library: http://mbed.org/cookbook/SPI-driven-QVGA-TFT 00004 * Manufacturer's page for the screen: http://www.mikroe.com/add-on-boards/display/tft-proto/ 00005 * UK distributor: http://www.mcustore.com/tft-proto-board.html 00006 * Partly adapted from Kamil Ondrousek's code for another screen: http://mbed.org/users/JLS/code/Mandelbrot-2/ 00007 */ 00008 #include "mbed.h" 00009 #include "SPI_TFT.h" 00010 #include "Arial12x12.h" 00011 #include "touch_tft.h" 00012 00013 #define WIDTH 320 00014 #define HEIGHT 240 00015 00016 touch_tft tt(p18,p19,p16,p17,p11, p12, p13, p14, p15,"TFT"); // x+,x-,y+,y-,mosi, miso, sclk, cs, reset 00017 00018 void drawMandelbrot(float centrex, float centrey, float zoom, int maxIter) { 00019 float xmin = centrex - 1.0/zoom; 00020 float xmax = centrex + 1.0/zoom; 00021 float ymin = centrey - HEIGHT / (WIDTH * zoom); 00022 float ymax = centrey + HEIGHT / (WIDTH *zoom); 00023 00024 float dx = (xmax - xmin) / WIDTH; 00025 float dy = (ymax - ymin) / HEIGHT; 00026 00027 float y = ymin; 00028 00029 float c; 00030 unsigned int cr, cg, cb; 00031 00032 for (int j = 0; j < HEIGHT; j++) { 00033 float x = xmin; 00034 00035 for (int i = 0; i < WIDTH; i++) { 00036 float a = x; 00037 float b = y; 00038 int n = 0; 00039 00040 while (n < maxIter) { 00041 float aa = a * a; 00042 float bb = b * b; 00043 float twoab = 2.0 * a * b; 00044 00045 a = aa - bb + x; 00046 b = twoab + y; 00047 00048 if(aa + bb > 16.0) { 00049 break; 00050 } 00051 n++; 00052 } 00053 00054 if (n == maxIter) { 00055 //It's in the set - Black 00056 tt.pixel(i,j,Black); 00057 } else { 00058 //Not in the set - pick a colour 00059 c = 3.0 * (maxIter-n)/maxIter; 00060 cr = ((c < 1.0) ? 255 * ( 1.0 - c) : (c > 2.0) ? 255 * (c - 2.0) : 0); 00061 cg = ((c < 1.0) ? 255 * c : (c > 2.0) ? 0 : 255 * (2.0 - c)); 00062 cb = ((c < 1.0) ? 0 : (c > 2.0) ? 255 * (3.0 - c) : 255 * (c - 1.0)); 00063 tt.pixel(i,j, RGB(cr,cg,cb)); 00064 } 00065 x += dx; 00066 } 00067 y += dy; 00068 } 00069 } 00070 00071 int main() 00072 { 00073 //Setup screen 00074 tt.claim(stdout); // send stdout to the TFT display 00075 tt.background(Black); // set background to black 00076 tt.foreground(White); // set chars to white 00077 tt.cls(); // clear the screen 00078 tt.set_font((unsigned char*) Arial12x12); // select the font 00079 tt.set_orientation(1); 00080 tt.calibrate(); // calibrate the touch 00081 point p; 00082 00083 tt.locate(0,0); 00084 00085 //Setup base Mandelbrot 00086 float centrex = -0.5; 00087 float centrey = 0.0; 00088 float zoom=0.5; 00089 int maxiterations = 150; 00090 00091 while(true) { 00092 //Draw it 00093 drawMandelbrot(centrex, centrey, zoom, maxiterations); 00094 00095 //Wait for a touch 00096 p=tt.get_touch(); 00097 while(!tt.is_touched(p)) { 00098 p=tt.get_touch(); 00099 } 00100 //Set new centre and zoom 00101 p = tt.to_pixel(p); 00102 centrex += (2.0 * p.x - WIDTH) / (zoom * WIDTH); 00103 centrey += (2.0 * p.y - HEIGHT) / (zoom * WIDTH); 00104 zoom *= 4.0; 00105 tt.rect(p.x - WIDTH / 8.0, p.y - HEIGHT / 8.0, p.x + WIDTH / 8.0, p.y + HEIGHT / 8.0, Yellow); 00106 } 00107 }
Generated on Tue Jul 12 2022 21:25:15 by
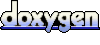