
Read only one key pressed
Dependencies: Hotboards_keypad mbed
main.cpp
00001 00002 #include "mbed.h" 00003 #include "Hotboards_keypad.h" 00004 00005 // Defines the keys array with it's respective number of rows & cols, 00006 // and with the value of each key 00007 char keys[ 4 ][ 4 ] = 00008 { 00009 { '1' , '2' , '3' , 'A' }, 00010 { '4' , '5' , '6' , 'B' }, 00011 { '7' , '8' , '9' , 'C' }, 00012 { '*' , '0' , '#' , 'D' } 00013 }; 00014 00015 // Defines the pins connected to the rows 00016 DigitalInOut rowPins[ 4 ] = { PA_6 , PA_7 , PB_6 , PC_7 }; 00017 // Defines the pins connected to the cols 00018 DigitalInOut colPins[ 4 ] = { PA_8 , PB_10 , PB_4 , PB_5 }; 00019 00020 // Creates a new keyboard with the values entered before 00021 Keypad kpd( makeKeymap( keys ) , rowPins , colPins , 4 , 4 ); 00022 00023 // Configures the serial port 00024 Serial pc( USBTX , USBRX ); 00025 00026 int main() 00027 { 00028 pc.printf( "Press any key: " ); 00029 while(1) 00030 { 00031 // Poll the keypad to look for any activation 00032 char key = kpd.getKey( ); 00033 00034 // If any key was pressed 00035 if( key ) 00036 { 00037 // Display the key pressed on serial port 00038 pc.printf( "%c" , key ); 00039 pc.printf( "\n\r" ); 00040 } 00041 } 00042 }
Generated on Mon Jul 18 2022 18:23:51 by
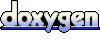