
Based on Alfredo Guerrero's WiiClassicControllerReader although I had to do additional decoding of packet. KL25Z talking to Wii classic controller.
Dependencies: CommonTypes WiiClassicControllerLib mbed
main.cpp
00001 /* 00002 * SOURCE FILE : main.cpp 00003 * 00004 * Test program to test out WiiClassicController class. 00005 * 00006 */ 00007 00008 #include "mbed.h" 00009 #include "WiiClassicControllerWithCalibration.h" 00010 00011 // Define this to dump packet in hex. 00012 #undef DUMP_PACKET 00013 00014 #define LOOP_DELAY (float)0.025 // seconds 00015 00016 // global declarations 00017 Serial pc(USBTX, USBRX); 00018 00019 /* Read from the Wii classic controller and display results on serial port output. 00020 * @param ctrlr Controller to read from. 00021 * @param portname Name of port you are reading from. 00022 */ 00023 static void ReadAndReport( WiiClassicControllerWithCalibration *ctrlr, const char *portname ) { 00024 static int counter = 0; 00025 if( ctrlr->Read() ) { 00026 00027 // Display updated every tenth time. 00028 if( counter == 0 ) { 00029 00030 pc.printf( "%c[H", 27 ); // VT100 home cursor 00031 pc.printf("%s\r\n", portname); 00032 00033 #ifdef DUMP_PACKET 00034 int bufSize = ctrlr->GetReadBufSize(); 00035 UInt8 *bufPtr = ctrlr->GetReadBuf(); 00036 for (int i = 0; i < bufSize; i++) { 00037 pc.printf("%x ", bufPtr[i]); 00038 } 00039 pc.printf("\r\n"); 00040 #endif 00041 00042 pc.printf("A=%u\r\n", ctrlr->GetButtonA()); 00043 pc.printf("B=%u\r\n", ctrlr->GetButtonB()); 00044 pc.printf("X=%u\r\n", ctrlr->GetButtonX()); 00045 pc.printf("Y=%u\r\n", ctrlr->GetButtonY()); 00046 pc.printf("ZL=%u\r\n", ctrlr->GetButtonZL()); 00047 pc.printf("ZR=%u\r\n", ctrlr->GetButtonZR()); 00048 pc.printf("Up=%u\r\n", ctrlr->GetButtonUp()); 00049 pc.printf("Down=%u\r\n", ctrlr->GetButtonDown()); 00050 pc.printf("Left=%u\r\n", ctrlr->GetButtonLeft()); 00051 pc.printf("Right=%u\r\n", ctrlr->GetButtonRight()); 00052 pc.printf("Home=%u\r\n", ctrlr->GetButtonHome()); 00053 pc.printf("Select=%u\r\n", ctrlr->GetButtonSelect()); 00054 pc.printf("Start=%u\r\n", ctrlr->GetButtonStart()); 00055 pc.printf("LeftTrigger=%2u (%6.3f)\r\n", ctrlr->GetLeftTrigger(), ctrlr->GetCalLeftTrigger() ); 00056 pc.printf("ButtonLT=%u\r\n", ctrlr->GetButtonLT()); 00057 pc.printf("RightTrigger=%2u (%6.3f)\r\n", ctrlr->GetRightTrigger(), ctrlr->GetCalRightTrigger() ); 00058 pc.printf("ButtonRT=%u\r\n", ctrlr->GetButtonRT()); 00059 pc.printf("LJoyX=%2u (%6.3f)\r\n", ctrlr->GetLJoyX(), ctrlr->GetCalLJoyX() ); 00060 pc.printf("LJoyY=%2u (%6.3f)\r\n", ctrlr->GetLJoyY(), ctrlr->GetCalLJoyY() ); 00061 pc.printf("RJoyX=%2u (%6.3f)\r\n", ctrlr->GetRJoyX(), ctrlr->GetCalRJoyX() ); 00062 pc.printf("RJoyY=%2u (%6.3f)\r\n", ctrlr->GetRJoyY(), ctrlr->GetCalRJoyY() ); 00063 if( ctrlr->IsCalibrating() ) { 00064 pc.puts( "Wiggle joysticks and triggers. Press HOME button to end calibration." ); 00065 } 00066 else { 00067 pc.puts( "Press A and B buttons simultaneously to start calibration." ); 00068 } 00069 00070 } 00071 00072 // Update counter. 00073 // Display is updated every so often. 00074 counter++; 00075 counter %= 10; 00076 00077 } 00078 else { 00079 pc.puts( "READ FAILURE\r\n" ); 00080 } 00081 } 00082 00083 /** Clear the screen. 00084 */ 00085 static void ClearScreen( void ) { 00086 pc.printf( "%c[2J", 27 ); // VT100 clear screen 00087 } 00088 00089 /** Main program. 00090 */ 00091 int main() { 00092 WiiClassicControllerWithCalibration ctrlrA( PTE0, PTE1 ); 00093 pc.baud( 115200 ); 00094 pc.format( 8, Serial::None, 1 ); 00095 ClearScreen(); 00096 while (true) { 00097 ReadAndReport( &ctrlrA, "PORT A" ); 00098 // If both A and B buttons are pressed and not already calibrating 00099 // then start calibrating and clear the screen. 00100 if( ctrlrA.GetButtonA() && ctrlrA.GetButtonB() && ! ctrlrA.IsCalibrating() ) { 00101 ctrlrA.StartCalibrating(); 00102 ClearScreen(); 00103 } 00104 // If HOME buttons is pressed and doing a calibration 00105 // then stop calibrating and clear the screen. 00106 if( ctrlrA.GetButtonHome() && ctrlrA.IsCalibrating() ) { 00107 ctrlrA.StopCalibrating(); 00108 ClearScreen(); 00109 } 00110 wait(LOOP_DELAY); 00111 } 00112 // Never gets here. 00113 // return EXIT_SUCCESS; 00114 }
Generated on Mon Jul 18 2022 08:21:07 by
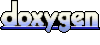