Library for communicating with a Wii classic controller using the I2C bus.
Dependents: WiiClassicControllerTest
WiiClassicControllerWithCalibration Class Reference
Derived from WiiClassicController but with calibrated analogue inputs. More...
#include <WiiClassicControllerWithCalibration.h>
Inherits WiiClassicController.
Public Types | |
enum | AnaIn |
Enumeration of all the analogue inputs on the Wii classic controller. More... | |
Public Member Functions | |
WiiClassicControllerWithCalibration (PinName sda, PinName scl) | |
Constructor. | |
virtual | ~WiiClassicControllerWithCalibration () |
Destructor. | |
virtual bool | Read (void) |
Read from the controller. | |
void | SetScaling (AnaIn input, float m, float c) |
Set scaling for a particular analogue input. | |
void | GetScaling (AnaIn input, float *m, float *c) const |
Get scaling for a particular analogue input. | |
float | GetCalLJoyX (void) const |
Get calibrated left joystick X reading. | |
float | GetCalLJoyY (void) const |
Get calibrated left joystick Y reading. | |
float | GetCalRJoyX (void) const |
Get calibrated right joystick X reading. | |
float | GetCalRJoyY (void) const |
Get calibrated right joystick Y reading. | |
float | GetCalLeftTrigger (void) const |
Get calibrated left trigger reading. | |
float | GetCalRightTrigger (void) const |
Get calibrated right trigger reading. | |
bool | IsCalibrating (void) const |
Get indication that calibration is in progress. | |
void | StartCalibrating (void) |
Start calibrating. | |
void | StopCalibrating (void) |
Stop calibrating. | |
UInt8 | GetLJoyX (void) const |
Read left joystick X axis. | |
UInt8 | GetLJoyY (void) const |
Read left joystick Y axis. | |
UInt8 | GetRJoyX (void) const |
Read right joystick X axis. | |
UInt8 | GetRJoyY (void) const |
Read right joystick Y axis. | |
bool | GetButtonX (void) const |
Read X button. | |
bool | GetButtonY (void) const |
Read Y button. | |
bool | GetButtonA (void) const |
Read A button. | |
bool | GetButtonB (void) const |
Read B button. | |
bool | GetButtonLT (void) const |
Read left trigger button. | |
bool | GetButtonRT (void) const |
Read right trigger button. | |
UInt8 | GetLeftTrigger (void) const |
Read left trigger reading. | |
UInt8 | GetRightTrigger (void) const |
Read right trigger reading. | |
bool | GetButtonZL (void) const |
Read ZL button. | |
bool | GetButtonZR (void) const |
Read ZR button. | |
bool | GetButtonSelect (void) const |
Read select (or minus) button. | |
bool | GetButtonHome (void) const |
Read home button. | |
bool | GetButtonStart (void) const |
Read start (or plus) button. | |
bool | GetButtonUp (void) const |
Read up button. | |
bool | GetButtonDown (void) const |
Read down button. | |
bool | GetButtonLeft (void) const |
Read left button. | |
bool | GetButtonRight (void) const |
Read right button. | |
UInt8 | GetReadBufSize (void) const |
Get size of read buffer. | |
UInt8 * | GetReadBuf (void) |
Get address of read buffer. |
Detailed Description
Derived from WiiClassicController but with calibrated analogue inputs.
Definition at line 15 of file WiiClassicControllerWithCalibration.h.
Member Enumeration Documentation
enum AnaIn |
Enumeration of all the analogue inputs on the Wii classic controller.
Definition at line 20 of file WiiClassicControllerWithCalibration.h.
Constructor & Destructor Documentation
WiiClassicControllerWithCalibration | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor.
- Parameters:
-
sda pin to use for SDA. scl pin to use for SCL.
Definition at line 14 of file WiiClassicControllerWithCalibration.cpp.
~WiiClassicControllerWithCalibration | ( | ) | [virtual] |
Destructor.
Definition at line 33 of file WiiClassicControllerWithCalibration.cpp.
Member Function Documentation
bool GetButtonA | ( | void | ) | const [inherited] |
Read A button.
- Returns:
- true if button is pressed.
Definition at line 89 of file WiiClassicController.h.
bool GetButtonB | ( | void | ) | const [inherited] |
Read B button.
- Returns:
- true if button is pressed.
Definition at line 96 of file WiiClassicController.h.
bool GetButtonDown | ( | void | ) | const [inherited] |
Read down button.
- Returns:
- true if button is pressed.
Definition at line 176 of file WiiClassicController.h.
bool GetButtonHome | ( | void | ) | const [inherited] |
Read home button.
- Returns:
- true if button is pressed.
Definition at line 155 of file WiiClassicController.h.
bool GetButtonLeft | ( | void | ) | const [inherited] |
Read left button.
- Returns:
- true if button is pressed.
Definition at line 183 of file WiiClassicController.h.
bool GetButtonLT | ( | void | ) | const [inherited] |
Read left trigger button.
- Returns:
- true if button is pressed.
Definition at line 103 of file WiiClassicController.h.
bool GetButtonRight | ( | void | ) | const [inherited] |
Read right button.
- Returns:
- true if button is pressed.
Definition at line 190 of file WiiClassicController.h.
bool GetButtonRT | ( | void | ) | const [inherited] |
Read right trigger button.
- Returns:
- true if button is pressed.
Definition at line 110 of file WiiClassicController.h.
bool GetButtonSelect | ( | void | ) | const [inherited] |
Read select (or minus) button.
- Returns:
- true if button is pressed.
Definition at line 148 of file WiiClassicController.h.
bool GetButtonStart | ( | void | ) | const [inherited] |
Read start (or plus) button.
- Returns:
- true if button is pressed.
Definition at line 162 of file WiiClassicController.h.
bool GetButtonUp | ( | void | ) | const [inherited] |
Read up button.
- Returns:
- true if button is pressed.
Definition at line 169 of file WiiClassicController.h.
bool GetButtonX | ( | void | ) | const [inherited] |
Read X button.
- Returns:
- true if button is pressed.
Definition at line 75 of file WiiClassicController.h.
bool GetButtonY | ( | void | ) | const [inherited] |
Read Y button.
- Returns:
- true if button is pressed.
Definition at line 82 of file WiiClassicController.h.
bool GetButtonZL | ( | void | ) | const [inherited] |
Read ZL button.
- Returns:
- true if button is pressed.
Definition at line 134 of file WiiClassicController.h.
bool GetButtonZR | ( | void | ) | const [inherited] |
Read ZR button.
- Returns:
- true if button is pressed.
Definition at line 141 of file WiiClassicController.h.
float GetCalLeftTrigger | ( | void | ) | const |
Get calibrated left trigger reading.
- Returns:
- a reading between 0 and +1.
Definition at line 113 of file WiiClassicControllerWithCalibration.cpp.
float GetCalLJoyX | ( | void | ) | const |
Get calibrated left joystick X reading.
- Returns:
- a reading between -1 and +1.
Definition at line 85 of file WiiClassicControllerWithCalibration.cpp.
float GetCalLJoyY | ( | void | ) | const |
Get calibrated left joystick Y reading.
- Returns:
- a reading between -1 and +1.
Definition at line 92 of file WiiClassicControllerWithCalibration.cpp.
float GetCalRightTrigger | ( | void | ) | const |
Get calibrated right trigger reading.
- Returns:
- a reading between 0 and +1.
Definition at line 120 of file WiiClassicControllerWithCalibration.cpp.
float GetCalRJoyX | ( | void | ) | const |
Get calibrated right joystick X reading.
- Returns:
- a reading between -1 and +1.
Definition at line 99 of file WiiClassicControllerWithCalibration.cpp.
float GetCalRJoyY | ( | void | ) | const |
Get calibrated right joystick Y reading.
- Returns:
- a reading between -1 and +1.
Definition at line 106 of file WiiClassicControllerWithCalibration.cpp.
UInt8 GetLeftTrigger | ( | void | ) | const [inherited] |
Read left trigger reading.
- Returns:
- reading as a number between 0 and 31.
Definition at line 117 of file WiiClassicController.h.
UInt8 GetLJoyX | ( | void | ) | const [inherited] |
Read left joystick X axis.
- Returns:
- joystick reading as number between 0 and 63.
Definition at line 43 of file WiiClassicController.h.
UInt8 GetLJoyY | ( | void | ) | const [inherited] |
Read left joystick Y axis.
- Returns:
- joystick reading as number between 0 and 63.
Definition at line 50 of file WiiClassicController.h.
UInt8* GetReadBuf | ( | void | ) | [inherited] |
Get address of read buffer.
- Returns:
- pointer to read buffer.
Definition at line 204 of file WiiClassicController.h.
UInt8 GetReadBufSize | ( | void | ) | const [inherited] |
Get size of read buffer.
- Returns:
- size of read buffer.
Definition at line 197 of file WiiClassicController.h.
UInt8 GetRightTrigger | ( | void | ) | const [inherited] |
Read right trigger reading.
- Returns:
- reading as a number between 0 and 31.
Definition at line 127 of file WiiClassicController.h.
UInt8 GetRJoyX | ( | void | ) | const [inherited] |
Read right joystick X axis.
- Returns:
- joystick reading as number between 0 and 31.
Definition at line 57 of file WiiClassicController.h.
UInt8 GetRJoyY | ( | void | ) | const [inherited] |
Read right joystick Y axis.
- Returns:
- joystick reading as number between 0 and 31.
Definition at line 68 of file WiiClassicController.h.
void GetScaling | ( | AnaIn | input, |
float * | m, | ||
float * | c | ||
) | const |
Get scaling for a particular analogue input.
- Parameters:
-
input channel to read. m scale (multiplier) for this analogue input return here. c offset for this analogue input returned here.
Definition at line 54 of file WiiClassicControllerWithCalibration.cpp.
bool IsCalibrating | ( | void | ) | const |
Get indication that calibration is in progress.
- Returns:
- true if calibration is in progress.
Definition at line 94 of file WiiClassicControllerWithCalibration.h.
bool Read | ( | void | ) | [virtual] |
Read from the controller.
This override will also update calibration information when calibration is in progress.
- Returns:
- true on success, false on failure.
Reimplemented from WiiClassicController.
Definition at line 67 of file WiiClassicControllerWithCalibration.cpp.
void SetScaling | ( | AnaIn | input, |
float | m, | ||
float | c | ||
) |
Set scaling for a particular analogue input.
- Parameters:
-
input channel to change. m scale (multiplier) for this analogue input. c offset for this analogue input.
Definition at line 41 of file WiiClassicControllerWithCalibration.cpp.
void StartCalibrating | ( | void | ) |
Start calibrating.
Every call to Read method updates calibration until StopCalibrating is called.
Definition at line 151 of file WiiClassicControllerWithCalibration.cpp.
void StopCalibrating | ( | void | ) |
Stop calibrating.
Definition at line 192 of file WiiClassicControllerWithCalibration.cpp.
Generated on Tue Jul 12 2022 20:58:08 by
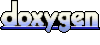