
A simple meter using Adafruit 2.8 TFT with touch v2
Dependencies: SPI_STMPE610 UniGraphic mbed vt100
meter.h
00001 #ifndef _METER_H_ 00002 #define _METER_H_ 00003 /** 00004 * meter class 00005 * A simple analog style meter 00006 **/ 00007 00008 class meter { 00009 public: 00010 /** 00011 * meter constructor 00012 * @param x right-top position of meter 00013 * @param y right-top position of meter 00014 * @param width width of meter 00015 * @param height height of meter 00016 * @param min minimum value of meter 00017 * @param max maximum value of meter 00018 */ 00019 meter(int x, int y, int width, int height, float min, float max) ; 00020 00021 /** 00022 * meter destructor 00023 */ 00024 ~meter(void) ; 00025 00026 /** 00027 * drawFrame draw meter frame/canvas 00028 */ 00029 void drawFrame(void) ; 00030 00031 /** 00032 * drawScale draw measuring mark 00033 */ 00034 void drawScale(void) ; 00035 00036 /** 00037 * drawHand draw meter hand at value position 00038 * @param value value to plot the hand 00039 */ 00040 void drawHand(float value) ; 00041 00042 /** 00043 * drawValue draw textual value in the lower part of the meter 00044 * @param value value to display (voltage assumed) 00045 */ 00046 void drawValue(float value) ; 00047 00048 /** 00049 * draw draw full set of the meter 00050 * @param value value to display and put hand 00051 */ 00052 void draw(float value) ; 00053 00054 /** 00055 * update draw only hand and value text 00056 */ 00057 void update(float value) ; 00058 00059 private: 00060 int _x ; 00061 int _y ; 00062 int _w ; 00063 int _h ; 00064 int _center_x ; 00065 int _center_y ; 00066 float _min ; 00067 float _max ; 00068 00069 } ; 00070 00071 #endif /* _METER_H_ */
Generated on Wed Jul 13 2022 19:37:46 by
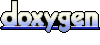