
Versie 0.2 Het versturen via de NRF werkt nog niet helemaal omdat er per 4 bytes verstuurd moet worden. Wordt gefixt d.m.v. dynamic stuff!
Fork of rtos_basic by
main.cpp
00001 /* *** INCLUDE **********************************************************************/ 00002 #include "mbed.h" 00003 #include "rtos.h" 00004 #include "nRF24L01P.h " 00005 00006 /* *** DEFINE ***********************************************************************/ 00007 #define sDestination_SIZE 20 00008 #define sMessage_SIZE 100 00009 00010 #define MF_RESET PC_13 00011 #define TRANSFER_SIZE_NRF 4 00012 #define TRANSFER_SIZE_UART 100 00013 #define nCS PA_15 00014 #define SCK PB_3 00015 #define MISO PB_4 00016 #define MOSI PB_5 00017 #define IRQ PB_6 00018 #define CE PB_7 00019 00020 /* *** THREADS **********************************************************************/ 00021 void LED_thread(void const *args); 00022 void MAIL_thread(void const *args); 00023 00024 /* *** FUNCTIONS ********************************************************************/ 00025 void initUART (void); 00026 void initWireLess(void); 00027 void UART_GetMessage(void); 00028 void WLESS_GetMessage(void); 00029 void flushCArray(char str[]); 00030 00031 /* *** GLOBAL DATA ******************************************************************/ 00032 // UART 00033 Serial raspPi(USBTX, USBRX); 00034 char getRaspPiData[TRANSFER_SIZE_UART], sendRaspPiData[TRANSFER_SIZE_UART]; 00035 int getRaspPiDataCnt, sendRaspPiDataCnt = 0; 00036 00037 // WIRELESS 00038 InterruptIn irqWLESS(IRQ); 00039 nRF24L01P NRF(MOSI, MISO, SCK, nCS, CE, IRQ); 00040 char getWLESSData[TRANSFER_SIZE_NRF], sendWLESSData[TRANSFER_SIZE_NRF]; 00041 int getWLESSDataCnt, sendWLESSDataCnt = 0; 00042 unsigned long long myAdress = 0x010203; 00043 unsigned long long otherAdress = 0x030201; 00044 00045 // MAIL 00046 typedef struct mail_t { 00047 char sDestination[sDestination_SIZE]; // Name of the Thread 00048 char sMessage[sMessage_SIZE]; // Type here you message 00049 }mail_t; 00050 00051 Mail<mail_t, 10> mailBox; 00052 mail_t *mail; 00053 00054 DigitalOut led2(LED2); 00055 //Use for debug!! 00056 DigitalOut check1(PB_15); 00057 DigitalOut check2(PB_14); 00058 DigitalOut check3(PB_13); 00059 // End debug 00060 00061 /* *** MAIN *************************************************************************/ 00062 int main() { 00063 00064 Thread threadLED(LED_thread); 00065 Thread threadMAIL(MAIL_thread); 00066 initUART(); 00067 initWireLess(); 00068 irqWLESS.fall(&WLESS_GetMessage); 00069 00070 while(1) { 00071 ; 00072 } 00073 } 00074 00075 /* *** LED THREAD *******************************************************************/ 00076 void LED_thread(void const *args) { 00077 while (true) { 00078 led2 = !led2; 00079 Thread::wait(500); 00080 } 00081 } 00082 00083 /* *** MAIL THREAD ******************************************************************/ 00084 void MAIL_thread(void const *args) { 00085 while(true) { 00086 osEvent evt = mailBox.get(); 00087 /* 00088 if (evt.status == osEventMail) { 00089 if (strcmp(mail->sDestination, "WLESS") == 0) { 00090 // functie aanroepen voor de NRF 00091 } 00092 else if (strcmp(mail->sDestination, "UART") == 0) { 00093 // functie aanroepen voor de UART 00094 } 00095 else if (strcmp(mail->sDestination, "LED") == 0) { 00096 // functie aanroepen voor de LED 00097 } 00098 mailBox.free(mail); 00099 } 00100 */ 00101 if (evt.status == osEventMail) { 00102 if (strcmp(mail->sDestination, "WLESS") == 0) { 00103 00104 raspPi.printf("Data from mail: %s ", mail->sMessage); 00105 00106 sendWLESSDataCnt = 0; 00107 // Deze functie werkt nog helemaal omdat er per 4 bytes verstuurd moeten worden... 00108 // Wanneer dit aangepast wordt doet hij het voor 100%! 00109 for (int sndData = 0; mail->sMessage[sndData] != '!'; sndData++) { 00110 00111 00112 sendWLESSData[sendWLESSDataCnt++] = mail->sMessage[sndData]; 00113 00114 if (sendWLESSDataCnt == TRANSFER_SIZE_NRF) { 00115 00116 NRF.write( NRF24L01P_PIPE_P0, sendWLESSData, TRANSFER_SIZE_NRF); 00117 sendWLESSDataCnt = 0; 00118 } 00119 else if (mail->sMessage[sndData] == '!') { 00120 00121 for (int sndRestData = sendWLESSDataCnt; sndRestData <= TRANSFER_SIZE_NRF; sndRestData++) { 00122 sendWLESSData[sndRestData] = 0; 00123 } 00124 NRF.write( NRF24L01P_PIPE_P0, sendWLESSData, TRANSFER_SIZE_NRF ); 00125 } 00126 } 00127 00128 flushCArray(sendWLESSData); 00129 mailBox.free(mail); 00130 } 00131 } 00132 Thread::wait(100); 00133 } 00134 } 00135 00136 /* *** INIT UART ********************************************************************/ 00137 void initUART () { 00138 raspPi.baud(9600); 00139 raspPi.attach(&UART_GetMessage); 00140 flushCArray(getRaspPiData); 00141 flushCArray(sendRaspPiData); 00142 raspPi.printf("Hi, from NUCLEO! \r\n"); 00143 } 00144 00145 00146 /* *** INIT WIRELESS ****************************************************************/ 00147 void initWireLess() { 00148 flushCArray(getWLESSData); 00149 flushCArray(sendWLESSData); 00150 00151 NRF.powerUp(); 00152 NRF.setTransferSize( TRANSFER_SIZE_NRF ); 00153 NRF.setTxAddress(otherAdress, 3); 00154 NRF.setRxAddress(otherAdress, 3, NRF24L01P_PIPE_P0); 00155 NRF.enableAutoAcknowledge(NRF24L01P_PIPE_P0); 00156 NRF.enableAutoRetransmit(1000, 10); 00157 NRF.setAirDataRate(NRF24L01P_DATARATE_250_KBPS); 00158 NRF.setRfOutputPower(NRF24L01P_TX_PWR_ZERO_DB); 00159 NRF.setRxAddress(myAdress, 3, NRF24L01P_PIPE_P1); 00160 NRF.setRfFrequency(2500); 00161 00162 NRF.setReceiveMode(); 00163 NRF.enable(); 00164 00165 // USE FOR DEBUG 00166 raspPi.printf( "NRF+ Frequency : %d MHz\r\n", NRF.getRfFrequency() ); 00167 raspPi.printf( "NRF+ Output power : %d dBm\r\n", NRF.getRfOutputPower() ); 00168 raspPi.printf( "NRF+ Data Rate : %d kbps\r\n", NRF.getAirDataRate() ); 00169 raspPi.printf( "NRF+ TX Address : 0x%010llX\r\n", NRF.getTxAddress() ); 00170 raspPi.printf( "NRF+ RX0 Address : 0x%010llX\r\n", NRF.getRxAddress(0) ); 00171 raspPi.printf( "NRF+ RX1 Address : 0x%010llX\r\n", NRF.getRxAddress(1) ); 00172 raspPi.printf( "Type keys to test transfers:\r\n (transfers are grouped into %d characters)\r\n", TRANSFER_SIZE_NRF ); 00173 00174 } 00175 00176 /* *** UART GET MESSAGE *************************************************************/ 00177 void UART_GetMessage () { 00178 00179 if (getRaspPiDataCnt <= TRANSFER_SIZE_UART){ 00180 getRaspPiData[getRaspPiDataCnt] = raspPi.getc(); 00181 raspPi.putc(getRaspPiData[getRaspPiDataCnt]); 00182 getRaspPiDataCnt++; 00183 } 00184 00185 if(getRaspPiData[getRaspPiDataCnt-1] == '!'){ 00186 mail = mailBox.alloc(); 00187 //strncpy (mail->sDestination, "WLESS", strlen("WLESS")); 00188 //strncpy (mail->sMessage, getRaspPiData, getRaspPiDataCnt-1); 00189 strcpy (mail->sDestination, "WLESS"); 00190 strcpy (mail->sMessage, getRaspPiData); 00191 mailBox.put(mail); 00192 getRaspPiDataCnt = 0; 00193 flushCArray(getRaspPiData); 00194 } 00195 } 00196 00197 /* *** WIRELESS GET MESSAGE *********************************************************/ 00198 void WLESS_GetMessage () { 00199 // Deze functie nog niet getest! 00200 getWLESSDataCnt = NRF.read( NRF24L01P_PIPE_P0, getWLESSData, sizeof( getWLESSData ) ); 00201 00202 for ( int i = 0; getWLESSDataCnt > 0; getWLESSDataCnt--, i++ ) { 00203 raspPi.putc( getWLESSData[i] ); 00204 } 00205 } 00206 00207 /* *** FLUSH CHAR ARRAY *************************************************************/ 00208 void flushCArray(char str[]) { 00209 memset(str, '\n', strlen(str)); 00210 }
Generated on Sat Jul 16 2022 03:22:46 by
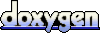