Slightly modified MD5 lib. Allows access to raw binary instead of hexadecimal ascii.
Embed:
(wiki syntax)
Show/hide line numbers
md5.h
00001 /* MD5 00002 converted to C++ class by Frank Thilo (thilo@unix-ag.org) 00003 for bzflag (http://www.bzflag.org) 00004 00005 based on: 00006 00007 md5.h and md5.c 00008 reference implementation of RFC 1321 00009 00010 Copyright (C) 1991-2, RSA Data Security, Inc. Created 1991. All 00011 rights reserved. 00012 00013 License to copy and use this software is granted provided that it 00014 is identified as the "RSA Data Security, Inc. MD5 Message-Digest 00015 Algorithm" in all material mentioning or referencing this software 00016 or this function. 00017 00018 License is also granted to make and use derivative works provided 00019 that such works are identified as "derived from the RSA Data 00020 Security, Inc. MD5 Message-Digest Algorithm" in all material 00021 mentioning or referencing the derived work. 00022 00023 RSA Data Security, Inc. makes no representations concerning either 00024 the merchantability of this software or the suitability of this 00025 software for any particular purpose. It is provided "as is" 00026 without express or implied warranty of any kind. 00027 00028 These notices must be retained in any copies of any part of this 00029 documentation and/or software. 00030 00031 */ 00032 00033 #ifndef BZF_MD5_H 00034 #define BZF_MD5_H 00035 00036 #include <string> 00037 00038 00039 /** a small class for calculating MD5 hashes of strings or byte arrays 00040 it is not meant to be fast or secure 00041 00042 usage: 1) feed it blocks of uchars with update() 00043 2) finalize() 00044 3) get hexdigest() string 00045 or 00046 MD5(std::string).hexdigest() 00047 00048 assumes that char is 8 bit and int is 32 bit 00049 */ 00050 class MD5 00051 { 00052 public: 00053 typedef unsigned int size_type; // must be 32bit 00054 00055 MD5(); 00056 /** 00057 take string, hash it and finalize 00058 @param text the string to hash 00059 */ 00060 MD5(const std::string& text); 00061 /** 00062 add text to hash 00063 @param buf the text to add to the hash 00064 @param text length 00065 */ 00066 void update(const unsigned char *buf, size_type length); 00067 /** 00068 add text to hash 00069 @param buf the text to add to the hash 00070 @param text length 00071 */ 00072 void update(const char *buf, size_type length); 00073 /** 00074 calculate the final hash value 00075 */ 00076 MD5& finalize(); 00077 /** 00078 @return the hash as hex string 00079 */ 00080 std::string hexdigest () const; 00081 00082 void init(); 00083 00084 /** 00085 @return the hash as binary data, an array of 16 bytes. 00086 */ 00087 const unsigned char* rawdigest () const { return digest; } 00088 00089 private: 00090 typedef unsigned char uint1; // 8bit 00091 typedef unsigned int uint4; // 32bit 00092 enum {blocksize = 64}; // VC6 won't eat a const static int here 00093 00094 void transform(const uint1 block[blocksize]); 00095 static void decode(uint4 output[], const uint1 input[], size_type len); 00096 static void encode(uint1 output[], const uint4 input[], size_type len); 00097 00098 bool finalized; 00099 uint1 buffer[blocksize]; // bytes that didn't fit in last 64 byte chunk 00100 uint4 count[2]; // 64bit counter for number of bits (lo, hi) 00101 uint4 state[4]; // digest so far 00102 uint1 digest[16]; // the result 00103 00104 // low level logic operations 00105 static inline uint4 F(uint4 x, uint4 y, uint4 z); 00106 static inline uint4 G(uint4 x, uint4 y, uint4 z); 00107 static inline uint4 H(uint4 x, uint4 y, uint4 z); 00108 static inline uint4 I(uint4 x, uint4 y, uint4 z); 00109 static inline uint4 rotate_left(uint4 x, int n); 00110 static inline void FF(uint4 &a, uint4 b, uint4 c, uint4 d, uint4 x, uint4 s, uint4 ac); 00111 static inline void GG(uint4 &a, uint4 b, uint4 c, uint4 d, uint4 x, uint4 s, uint4 ac); 00112 static inline void HH(uint4 &a, uint4 b, uint4 c, uint4 d, uint4 x, uint4 s, uint4 ac); 00113 static inline void II(uint4 &a, uint4 b, uint4 c, uint4 d, uint4 x, uint4 s, uint4 ac); 00114 }; 00115 00116 std::string md5(const std::string str); 00117 00118 #endif
Generated on Thu Sep 8 2022 01:08:55 by
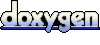