PCA9555
Dependents: Telliskivi2_2014 PowerManagementBoard_Rev_A_2017
PCA9555.h
00001 #ifndef MBED_PCA9555_H 00002 #define MBED_PCA9555_H 00003 00004 #include "mbed.h" 00005 00006 /** Interface to the PCA9555 I2C 16 Bit IO expander */ 00007 class PCA9555 { 00008 protected: 00009 InterruptIn _irqpin; 00010 FunctionPointer _callbackChange; 00011 public: 00012 /** Create an instance of the PCA9555 connected to specfied I2C pins, with the specified address. 00013 * 00014 * @param sda The I2C data pin 00015 * @param scl The I2C clock pin 00016 * @param interruptPin The pin connected to PCA9555 interrupt 00017 * @param address The I2C address for this PCA9555 00018 */ 00019 PCA9555(PinName sda, PinName scl, PinName interrupPin, int address); 00020 00021 /** Read the IO pin levels 00022 * 00023 * @return The two bytes read 00024 */ 00025 int read(); 00026 int getLastRead(); 00027 /** Write to the IO pins 00028 * 00029 * @param data The 16 bits to write to the IO port 00030 */ 00031 void write(int data); 00032 00033 /** Set one pin 00034 * 00035 * @param pinNumber The number of pin to set 00036 */ 00037 void setPin(unsigned int pinNumber); 00038 00039 /** Clear one pin 00040 * 00041 * @param pinNumber The number of pin to clear 00042 */ 00043 void clearPin(unsigned int pinNumber); 00044 00045 /** Toggle one pin 00046 * 00047 * @param pinNumber The number of pin to toggle 00048 */ 00049 void togglePin(unsigned int pinNumber); 00050 00051 /** Get state of pin 00052 * 00053 * @param pinNumber The number of pin 00054 */ 00055 bool getPin(unsigned int pinNumber); 00056 void writePins(); 00057 00058 /** Set direction of pin 00059 * 00060 * @param pinNumber The number of pin to set 00061 */ 00062 void setDirection(int data); 00063 00064 void change(void (*function)(void)); 00065 00066 template<typename T> 00067 void change(T *object, void (T::*member)(void)) { 00068 _callbackChange.attach(object, member); 00069 } 00070 00071 private: 00072 I2C _i2c; 00073 int _address; 00074 unsigned int currentWriteState; 00075 unsigned int lastReadState; 00076 DigitalOut led3; 00077 int retryCount; 00078 int currentRetryCount; 00079 void callChange(void); 00080 }; 00081 00082 #endif
Generated on Wed Jul 13 2022 02:33:02 by
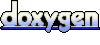