MCP3021
Embed:
(wiki syntax)
Show/hide line numbers
MCP3021.cpp
00001 #include "MCP3021.h" 00002 00003 //Create instance 00004 MCP3021::MCP3021(PinName sda, PinName scl, float supplyVoltage) : i2c(sda, scl), _supplyVoltage(supplyVoltage) { 00005 } 00006 00007 //destroy instance 00008 MCP3021::~MCP3021() { 00009 } 00010 00011 float MCP3021::read() { 00012 00013 //You cannot write to an MCP3021, it has no writable registers. 00014 //MCP3021 also requires an ACKnowledge between each byte sent, before it will send the next byte. So we need to be a bit manual with how we talk to it. 00015 //It also needs an (NOT) ACKnowledge after the second byte or it will keep sending bytes (continuous sampling) 00016 // 00017 //From the datasheet. 00018 // 00019 //I2C.START 00020 //Send 8 bit device/ part address to open conversation. (See .h file for part explanation) 00021 //read a byte (with ACK) 00022 //read a byte (with NAK) 00023 //I2C.STOP 00024 00025 00026 // char data[2]; 00027 00028 i2c.start(); 00029 int acknowledged = i2c.write(MCP3021_CONVERSE); //send a byte to start the conversation. It should be acknowledged. 00030 _data[0] = i2c.read(1); //read a byte. acknowledge when we have it. 00031 _data[1] = i2c.read(0); //read the second byte. (n)acknowledge when we have it to stop the flow. 00032 i2c.stop(); 00033 00034 //convert to 12 bit. 00035 short res; 00036 int _10_bit_var; // 2 bytes 00037 char _4_bit_MSnibble = _data[0]; // 1 byte, example 0000 1000 00038 char _6_bit_LSByte = _data[1]; // 1 byte, example 1111 0000 00039 00040 _10_bit_var = ((0x0F & _4_bit_MSnibble) << 6) | _6_bit_LSByte >> 2; //example 100011110000 00041 res = _10_bit_var; 00042 00043 return (_supplyVoltage / 1024) * res; 00044 00045 }
Generated on Thu Jul 21 2022 20:01:38 by
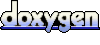