QP is an event-driven, RTOS-like, active object framework for microcontrollers, such as mbed. The QP framework provides thread-safe execution of active objects (concurrent state machines) and support both manual and automatic coding of UML statecharts in readable, production-quality C or C++. Automatic code generation of QP code is supported by the free QM modeling tool.
Dependents: qp_hangman qp_dpp qp_blinky
qp_port.h
00001 ////////////////////////////////////////////////////////////////////////////// 00002 // Product: QP/C++ port to ARM Cortex, selectable Vanilla/QK, mbed compiler 00003 // Last Updated for Version: 4.5.02 00004 // Date of the Last Update: Sep 04, 2012 00005 // 00006 // Q u a n t u m L e a P s 00007 // --------------------------- 00008 // innovating embedded systems 00009 // 00010 // Copyright (C) 2002-2012 Quantum Leaps, LLC. All rights reserved. 00011 // 00012 // This program is open source software: you can redistribute it and/or 00013 // modify it under the terms of the GNU General Public License as published 00014 // by the Free Software Foundation, either version 2 of the License, or 00015 // (at your option) any later version. 00016 // 00017 // Alternatively, this program may be distributed and modified under the 00018 // terms of Quantum Leaps commercial licenses, which expressly supersede 00019 // the GNU General Public License and are specifically designed for 00020 // licensees interested in retaining the proprietary status of their code. 00021 // 00022 // This program is distributed in the hope that it will be useful, 00023 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00024 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00025 // GNU General Public License for more details. 00026 // 00027 // You should have received a copy of the GNU General Public License 00028 // along with this program. If not, see <http://www.gnu.org/licenses/>. 00029 // 00030 // Contact information: 00031 // Quantum Leaps Web sites: http://www.quantum-leaps.com 00032 // http://www.state-machine.com 00033 // e-mail: info@quantum-leaps.com 00034 ////////////////////////////////////////////////////////////////////////////// 00035 #ifndef qp_port_h 00036 #define qp_port_h 00037 00038 #include "qp_config.h" // QP configuration defined at the application level 00039 00040 //............................................................................ 00041 00042 // QF interrupt disable/enable 00043 #define QF_INT_DISABLE() __disable_irq() 00044 #define QF_INT_ENABLE() __enable_irq() 00045 00046 // QF critical section entry/exit 00047 // QF_CRIT_STAT_TYPE not defined: "unconditional interrupt unlocking" policy 00048 #define QF_CRIT_ENTRY(dummy) __disable_irq() 00049 #define QF_CRIT_EXIT(dummy) __enable_irq() 00050 00051 #ifdef QK_PREEMPTIVE 00052 // QK interrupt entry and exit 00053 #define QK_ISR_ENTRY() do { \ 00054 __disable_irq(); \ 00055 ++QK_intNest_; \ 00056 QF_QS_ISR_ENTRY(QK_intNest_, QK_currPrio_); \ 00057 __enable_irq(); \ 00058 } while (false) 00059 00060 #define QK_ISR_EXIT() do { \ 00061 __disable_irq(); \ 00062 QF_QS_ISR_EXIT(QK_intNest_, QK_currPrio_); \ 00063 --QK_intNest_; \ 00064 *Q_UINT2PTR_CAST(uint32_t, 0xE000ED04U) = \ 00065 static_cast<uint32_t>(0x10000000U); \ 00066 __enable_irq(); \ 00067 } while (false) 00068 00069 #else 00070 #define QK_ISR_ENTRY() ((void)0) 00071 #define QK_ISR_EXIT() ((void)0) 00072 #endif 00073 00074 #ifdef Q_SPY 00075 #define QS_TIME_SIZE 4 00076 #define QS_OBJ_PTR_SIZE 4 00077 #define QS_FUN_PTR_SIZE 4 00078 #endif 00079 00080 #include <stdint.h> // mbed compiler supports standard exact-width integers 00081 00082 #include "qp.h" // QP platform-independent interface 00083 00084 #endif // qp_port_h
Generated on Wed Jul 13 2022 11:50:18 by
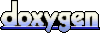