Library used to fetch counter(s) from Munin. Data later can be presented on LCD etc.
Dependents: munin-display-board
MuninClient Class Reference
MuninClient class, used to fetch counters from Munin. More...
#include <munincli.h>
Public Member Functions | |
void | dhcp_connection () |
Connect to DHCP. | |
int | socket_connection (const char *server, const int port) |
Create a dispBoB object defined on the I2C master bus. | |
void | socket_close () |
Closes socket conenction to Munin server. | |
int | socket_recv () |
Recv data from Munin to buffer 'buf'. | |
int | socket_recv_all () |
Recv data from Munin to buffer 'buf'. | |
int | socket_send (char *cmd, const int cmd_size) |
Send data using socket to Munin server. | |
int | get_param_value (std::string param_name) |
Explodes Munin command reply into individual counters divided by ' '. |
Detailed Description
MuninClient class, used to fetch counters from Munin.
Example:
#include "munincli.h" #include "dispBoB.h" namespace { const char* MUNIN_SERVER_ADDRESS = "172.28.22.45"; const int MUNIN_SERVER_PORT = 4949; } namespace { // Munin command(s): char CMD_FETCH_USERS[] = "fetch users\n"; } int main() { MuninClient munin_lcd; munin_lcd.dhcp_connection(); // DHCP connection dispBoB bob_display(p28, p27, p26); while(true) { while (munin_lcd.socket_connection(MUNIN_SERVER_ADDRESS, MUNIN_SERVER_PORT) < 0) { wait(1); } munin_lcd.socket_recv(); munin_lcd.socket_send(CMD_FETCH_USERS, sizeof(CMD_FETCH_USERS)); munin_lcd.socket_recv_all(); { const char *param = "usercount.value"; const int counter_value = munin_lcd.get_param_value(param); bob_display.cls(); bob_display.printf("% 6d\n", counter_value); } munin_lcd.socket_close(); wait(3); } }
Definition at line 68 of file munincli.h.
Member Function Documentation
void dhcp_connection | ( | ) |
Connect to DHCP.
Definition at line 73 of file munincli.h.
int get_param_value | ( | std::string | param_name ) |
Explodes Munin command reply into individual counters divided by '
'.
Function extract counter value from conunter wirh name param_name.
- Parameters:
-
param_name counter name
Definition at line 131 of file munincli.h.
void socket_close | ( | ) |
Closes socket conenction to Munin server.
Definition at line 94 of file munincli.h.
int socket_connection | ( | const char * | server, |
const int | port | ||
) |
Create a dispBoB object defined on the I2C master bus.
- Parameters:
-
server Munin server IP / name port Munin server port
Definition at line 85 of file munincli.h.
int socket_recv | ( | ) |
Recv data from Munin to buffer 'buf'.
Definition at line 100 of file munincli.h.
int socket_recv_all | ( | ) |
Recv data from Munin to buffer 'buf'.
Used to fetch reply for Munin command (given counter name)
Definition at line 110 of file munincli.h.
int socket_send | ( | char * | cmd, |
const int | cmd_size | ||
) |
Send data using socket to Munin server.
Used to send command to Munin server.
- Parameters:
-
cmd Munin command string, must end with
cmd_size Munin command buffer size
Definition at line 122 of file munincli.h.
Generated on Fri Jul 15 2022 08:08:27 by
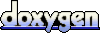