PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
lpc_defs.h
00001 #ifndef LPCDEFS_H 00002 #define LPCDEFS_H 00003 00004 #include <stdint.h> 00005 00006 #ifdef __cplusplus 00007 #define __I volatile /*!< Defines 'read only' permissions */ 00008 #else 00009 #define __I volatile const /*!< Defines 'read only' permissions */ 00010 #endif 00011 #define __O volatile /*!< Defines 'write only' permissions */ 00012 #define __IO volatile /*!< Defines 'read / write' permissions */ 00013 00014 00015 //#define LPC_PMU_BASE 0x40038000 00016 //#define LPC_IOCON_BASE 0x40044000 00017 #define LPC_SYSCTL_BASE 0x40048000 00018 //#define LPC_GPIO_PORT_BASE 0xA0000000 00019 #define LPC_GPIO_GROUP_INT0_BASE 0x4005C000 00020 #define LPC_GPIO_GROUP_INT1_BASE 0x40060000 00021 #define LPC_PIN_INT_BASE 0xA0004000 00022 //#define LPC_USART0_BASE 0x40008000 00023 //#define LPC_USART1_BASE 0x4006C000 00024 //#define LPC_USART2_BASE 0x40070000 00025 //#define LPC_USART3_BASE 0x40074000 00026 //#define LPC_USART4_BASE 0x4004C000 00027 //#define LPC_I2C0_BASE 0x40000000 00028 //#define LPC_I2C1_BASE 0x40020000 00029 //#define LPC_SSP0_BASE 0x40040000 00030 //#define LPC_SSP1_BASE 0x40058000 00031 #define LPC_USB0_BASE 0x40080000 00032 //#define LPC_ADC_BASE 0x4001C000 00033 //#define LPC_SCT0_BASE 0x5000C000 00034 //#define LPC_SCT1_BASE 0x5000E000 00035 #define LPC_TIMER16_0_BASE 0x4000C000 00036 #define LPC_TIMER16_1_BASE 0x40010000 00037 #define LPC_TIMER32_0_BASE 0x40014000 00038 #define LPC_TIMER32_1_BASE 0x40018000 00039 //#define LPC_RTC_BASE 0x40024000 00040 //#define LPC_WWDT_BASE 0x40004000 00041 //#define LPC_DMA_BASE 0x50004000 00042 //#define LPC_CRC_BASE 0x50000000 00043 #define LPC_FLASH_BASE 0x4003C000 00044 #define LPC_DMATRIGMUX_BASE 0x40028000UL 00045 00046 /** 00047 * @brief LPC11U6X System Control block structure 00048 */ 00049 typedef struct { /*!< SYSCTL Structure */ 00050 __IO uint32_t SYSMEMREMAP ; /*!< System Memory remap register */ 00051 __IO uint32_t PRESETCTRL ; /*!< Peripheral reset Control register */ 00052 __IO uint32_t SYSPLLCTRL ; /*!< System PLL control register */ 00053 __I uint32_t SYSPLLSTAT ; /*!< System PLL status register */ 00054 __IO uint32_t USBPLLCTRL ; /*!< USB PLL control register */ 00055 __I uint32_t USBPLLSTAT ; /*!< USB PLL status register */ 00056 __I uint32_t RESERVED1[1]; 00057 __IO uint32_t RTCOSCCTRL ; /*!< RTC Oscillator control register */ 00058 __IO uint32_t SYSOSCCTRL ; /*!< System Oscillator control register */ 00059 __IO uint32_t WDTOSCCTRL ; /*!< Watchdog Oscillator control register */ 00060 __I uint32_t RESERVED2[2]; 00061 __IO uint32_t SYSRSTSTAT ; /*!< System Reset Status register */ 00062 __I uint32_t RESERVED3[3]; 00063 __IO uint32_t SYSPLLCLKSEL ; /*!< System PLL clock source select register */ 00064 __IO uint32_t SYSPLLCLKUEN ; /*!< System PLL clock source update enable register*/ 00065 __IO uint32_t USBPLLCLKSEL ; /*!< USB PLL clock source select register */ 00066 __IO uint32_t USBPLLCLKUEN ; /*!< USB PLL clock source update enable register */ 00067 __I uint32_t RESERVED4[8]; 00068 __IO uint32_t MAINCLKSEL ; /*!< Main clock source select register */ 00069 __IO uint32_t MAINCLKUEN ; /*!< Main clock source update enable register */ 00070 __IO uint32_t SYSAHBCLKDIV ; /*!< System Clock divider register */ 00071 __I uint32_t RESERVED5; 00072 __IO uint32_t SYSAHBCLKCTRL ; /*!< System clock control register */ 00073 __I uint32_t RESERVED6[4]; 00074 __IO uint32_t SSP0CLKDIV ; /*!< SSP0 clock divider register */ 00075 __IO uint32_t USART0CLKDIV ; /*!< UART clock divider register */ 00076 __IO uint32_t SSP1CLKDIV ; /*!< SSP1 clock divider register */ 00077 __IO uint32_t FRGCLKDIV ; /*!< FRG clock divider (USARTS 1 - 4) register */ 00078 __I uint32_t RESERVED7[7]; 00079 __IO uint32_t USBCLKSEL ; /*!< USB clock source select register */ 00080 __IO uint32_t USBCLKUEN ; /*!< USB clock source update enable register */ 00081 __IO uint32_t USBCLKDIV ; /*!< USB clock source divider register */ 00082 __I uint32_t RESERVED8[5]; 00083 __IO uint32_t CLKOUTSEL ; /*!< Clock out source select register */ 00084 __IO uint32_t CLKOUTUEN ; /*!< Clock out source update enable register */ 00085 __IO uint32_t CLKOUTDIV ; /*!< Clock out divider register */ 00086 __I uint32_t RESERVED9; 00087 __IO uint32_t UARTFRGDIV ; /*!< USART fractional generator divider (USARTS 1 - 4) register */ 00088 __IO uint32_t UARTFRGMULT ; /*!< USART fractional generator multiplier (USARTS 1 - 4) register */ 00089 __I uint32_t RESERVED10; 00090 __IO uint32_t EXTTRACECMD ; /*!< External trace buffer command register */ 00091 __I uint32_t PIOPORCAP[3]; /*!< POR captured PIO status registers */ 00092 __I uint32_t RESERVED11[10]; 00093 __IO uint32_t IOCONCLKDIV[7]; /*!< IOCON block for programmable glitch filter divider registers */ 00094 __IO uint32_t BODCTRL ; /*!< Brown Out Detect register */ 00095 __IO uint32_t SYSTCKCAL ; /*!< System tick counter calibration register */ 00096 __I uint32_t RESERVED12[6]; 00097 __IO uint32_t IRQLATENCY ; /*!< IRQ delay register */ 00098 __IO uint32_t NMISRC ; /*!< NMI source control register */ 00099 __IO uint32_t PINTSEL[8]; /*!< GPIO pin interrupt select register 0-7 */ 00100 __IO uint32_t USBCLKCTRL ; /*!< USB clock control register */ 00101 __I uint32_t USBCLKST ; /*!< USB clock status register */ 00102 __I uint32_t RESERVED13[25]; 00103 __IO uint32_t STARTERP0 ; /*!< Start logic 0 interrupt wake-up enable register */ 00104 __I uint32_t RESERVED14[3]; 00105 __IO uint32_t STARTERP1 ; /*!< Start logic 1 interrupt wake-up enable register */ 00106 __I uint32_t RESERVED15[6]; 00107 __IO uint32_t PDSLEEPCFG ; /*!< Power down states in deep sleep mode register */ 00108 __IO uint32_t PDWAKECFG ; /*!< Power down states in wake up from deep sleep register */ 00109 __IO uint32_t PDRUNCFG ; /*!< Power configuration register*/ 00110 __I uint32_t RESERVED16[110]; 00111 __I uint32_t DEVICEID ; /*!< Device ID register */ 00112 } LPC_SYSCTL_T; 00113 00114 00115 #define LPC_SYSCTL_BASE 0x40048000 00116 00117 #define LPC_SYSCTL ((LPC_SYSCTL_T *) LPC_SYSCTL_BASE) 00118 00119 00120 00121 //#define LPC_DMA ((LPC_DMA_T *) LPC_DMA_BASE) 00122 00123 /* DMA channel source/address/next descriptor */ 00124 typedef struct { 00125 uint32_t xfercfg; /*!< Transfer configuration (only used in linked lists and ping-pong configs) */ 00126 uint32_t source; /*!< DMA transfer source end address */ 00127 uint32_t dest; /*!< DMA transfer desintation end address */ 00128 uint32_t next; /*!< Link to next DMA descriptor, must be 16 byte aligned */ 00129 } DMA_CHDESC_T; 00130 00131 00132 /* DMA channel mapping - each channel is mapped to an individual peripheral 00133 and direction or a DMA imput mux trigger */ 00134 typedef enum { 00135 SSP0_RX_DMA = 0, /*!< SSP0 receive DMA channel */ 00136 DMA_CH0 = SSP0_RX_DMA, 00137 DMAREQ_SSP0_TX, /*!< SSP0 transmit DMA channel */ 00138 DMA_CH1 = DMAREQ_SSP0_TX, 00139 DMAREQ_SSP1_RX, /*!< SSP1 receive DMA channel */ 00140 DMA_CH2 = DMAREQ_SSP1_RX, 00141 DMAREQ_SSP1_TX, /*!< SSP1 transmit DMA channel */ 00142 DMA_CH3 = DMAREQ_SSP1_TX, 00143 DMAREQ_USART0_RX, /*!< USART0 receive DMA channel */ 00144 DMA_CH4 = DMAREQ_USART0_RX, 00145 DMAREQ_USART0_TX, /*!< USART0 transmit DMA channel */ 00146 DMA_CH5 = DMAREQ_USART0_TX, 00147 DMAREQ_USART1_RX, /*!< USART1 transmit DMA channel */ 00148 DMA_CH6 = DMAREQ_USART1_RX, 00149 DMAREQ_USART1_TX, /*!< USART1 transmit DMA channel */ 00150 DMA_CH7 = DMAREQ_USART1_TX, 00151 DMAREQ_USART2_RX, /*!< USART2 transmit DMA channel */ 00152 DMA_CH8 = DMAREQ_USART2_RX, 00153 DMAREQ_USART2_TX, /*!< USART2 transmit DMA channel */ 00154 DMA_CH9 = DMAREQ_USART2_TX, 00155 DMAREQ_USART3_RX, /*!< USART3 transmit DMA channel */ 00156 DMA_CH10 = DMAREQ_USART3_RX, 00157 DMAREQ_USART3_TX, /*!< USART3 transmit DMA channel */ 00158 DMA_CH11 = DMAREQ_USART3_TX, 00159 DMAREQ_USART4_RX, /*!< USART4 transmit DMA channel */ 00160 DMA_CH12 = DMAREQ_USART4_RX, 00161 DMAREQ_USART4_TX, /*!< USART4 transmit DMA channel */ 00162 DMA_CH13 = DMAREQ_USART4_TX, 00163 DMAREQ_RESERVED_14, 00164 DMA_CH14 = DMAREQ_RESERVED_14, 00165 DMAREQ_RESERVED_15, 00166 DMA_CH15 = DMAREQ_RESERVED_15 00167 } DMA_CHID_T; 00168 00169 /* On LPC412x, Max DMA channel is 22 */ 00170 #define MAX_DMA_CHANNEL (DMA_CH15 + 1) 00171 00172 #undef _BIT 00173 /* Set bit macro */ 00174 #define _BIT(n) (1 << (n)) 00175 00176 /* _SBF(f,v) sets the bit field starting at position "f" to value "v". 00177 * _SBF(f,v) is intended to be used in "OR" and "AND" expressions: 00178 * e.g., "((_SBF(5,7) | _SBF(12,0xF)) & 0xFFFF)" 00179 */ 00180 #undef _SBF 00181 /* Set bit field macro */ 00182 #define _SBF(f, v) ((v) << (f)) 00183 00184 /* _BITMASK constructs a symbol with 'field_width' least significant 00185 * bits set. 00186 * e.g., _BITMASK(5) constructs '0x1F', _BITMASK(16) == 0xFFFF 00187 * The symbol is intended to be used to limit the bit field width 00188 * thusly: 00189 * <a_register> = (any_expression) & _BITMASK(x), where 0 < x <= 32. 00190 * If "any_expression" results in a value that is larger than can be 00191 * contained in 'x' bits, the bits above 'x - 1' are masked off. When 00192 * used with the _SBF example above, the example would be written: 00193 * a_reg = ((_SBF(5,7) | _SBF(12,0xF)) & _BITMASK(16)) 00194 * This ensures that the value written to a_reg is no wider than 00195 * 16 bits, and makes the code easier to read and understand. 00196 */ 00197 #undef _BITMASK 00198 /* Bitmask creation macro */ 00199 #define _BITMASK(field_width) ( _BIT(field_width) - 1) 00200 00201 /* NULL pointer */ 00202 #ifndef NULL 00203 #define NULL ((void *) 0) 00204 #endif 00205 00206 /* Number of elements in an array */ 00207 #define NELEMENTS(array) (sizeof(array) / sizeof(array[0])) 00208 00209 //#define LPC_SCT0 ((LPC_SCT_T *) LPC_SCT0_BASE) 00210 //#define LPC_SCT1 ((LPC_SCT_T *) LPC_SCT1_BASE) 00211 #define LPC_TIMER16_0 ((LPC_TIMER_T *) LPC_TIMER16_0_BASE) 00212 #define LPC_TIMER16_1 ((LPC_TIMER_T *) LPC_TIMER16_1_BASE) 00213 #define LPC_TIMER32_0 ((LPC_TIMER_T *) LPC_TIMER32_0_BASE) 00214 #define LPC_TIMER32_1 ((LPC_TIMER_T *) LPC_TIMER32_1_BASE) 00215 00216 #endif 00217
Generated on Tue Jul 12 2022 11:20:33 by
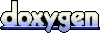