PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
Pokitto_settings.h
00001 /**************************************************************************/ 00002 /*! 00003 @file Pokitto_settings.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 00038 #ifndef POKITTO_SETTINGS_H 00039 #define POKITTO_SETTINGS_H 00040 00041 #include "My_settings.h" 00042 00043 #ifdef PROJ_BOARDREV 00044 #define POK_BOARDREV PROJ_BOARDREV // which revision of Pokitto board 00045 #else 00046 #define POK_BOARDREV 2 // default is boardrev 2 (the 4-layer board) 00047 #endif 00048 00049 /** LOGO */ 00050 #ifdef PROJ_STARTUPLOGO 00051 #define POK_DISPLAYLOGO PROJ_STARTUPLOGO// if enabled, show logo at start 00052 #else 00053 #define POK_DISPLAYLOGO 1 00054 #endif 00055 00056 #define POK_ENABLE_REFRESHWITHWAIT 0 // choose whether waiting in application refreshes display or not 00057 #define POK_ENABLE_FPSCOUNTER 0 // turn off to save some cpu 00058 #define POK_ENABLE_SD 1 // Define true to include SD library 00059 #define POK_LOADER_COUNTDOWN 3 //how many seconds waiting for C press for loader 00060 00061 #ifndef PROJ_ENABLE_SOUND 00062 #define POK_ENABLE_SOUND 1 00063 #else 00064 #define POK_ENABLE_SOUND PROJ_ENABLE_SOUND 00065 #endif 00066 00067 #ifndef PROJ_SOUND_BUFFERED 00068 #define POK_SOUND_BUFFERED 0 00069 #else 00070 #define POK_SOUND_BUFFERED PROJ_SOUND_BUFFERED 00071 #endif 00072 00073 #ifndef PROJ_GBSOUND 00074 #if POK_ENABLE_SOUND > 0 00075 #define POK_GBSOUND 0 00076 #endif 00077 #else 00078 #define POK_GBSOUND PROJ_GBSOUND 00079 //#define NUM_CHANNELS 2 00080 #endif 00081 00082 00083 #ifndef PROJ_STREAMING_MUSIC 00084 #define POK_STREAMING_MUSIC 0 // Define true to stream music from SD 00085 #else 00086 #define POK_STREAMING_MUSIC PROJ_STREAMING_MUSIC 00087 #endif // PROJ_STREAMING_MUSIC 00088 00089 #ifndef PROJ_ENABLE_SYNTH 00090 #define POK_ENABLE_SYNTH 0 00091 #else 00092 #define POK_ENABLE_SYNTH PROJ_ENABLE_SYNTH 00093 #endif // PROJ_ENABLE_SYNTH 00094 00095 #define HIGH_RAM_OFF 0 // SRAM1/SRAM2 are at the default setting 00096 #define HIGH_RAM_ON 1 // SRAM1/SRAM2 are enabled and free for use 00097 #define HIGH_RAM_MUSIC 2 // SRAM1/SRAM2 are enabled and used by music 00098 00099 #ifndef PROJ_HIGH_RAM 00100 #define POK_HIGH_RAM HIGH_RAM_OFF 00101 #else 00102 #define POK_HIGH_RAM PROJ_HIGH_RAM 00103 #endif 00104 00105 /** CONSOLE **/ 00106 #define POK_USE_CONSOLE 0 //if debugging console is available or not 00107 #define POK_CONSOLE_VISIBLE_AT_STARTUP 1 // whaddaya think ? 00108 #define POK_CONSOLE_INTERVAL 1000 // interval in ms how often console is drawn 00109 #if POK_USE_CONSOLE > 0 // this prevents trying to log messages if console is disabled 00110 #define POK_CONSOLE_LOG_BUTTONS 0 // if console logs keypresses 00111 #define POK_CONSOLE_LOG_COLLISIONS 1 // if console logs collisions 00112 #endif // POK_USE_CONSOLE 00113 #define CONSOLEBUFSIZE 20 00114 #define POK_SHOW_VOLUME 0 // volumebar drawn after console if enabled 00115 #define VOLUMEBAR_TIMEOUT 10 // frames before disappearing 00116 00117 /** PROJECT LIBRARY TYPE **/ 00118 // Tiled mode can NOT be buffered mode (fast mode, arduboy mode, gamebuino mode etc) 00119 #if PROJ_TILEDMODE > 0 00120 #define POK_TILEDMODE 1 00121 #ifdef PROJ_TILEWIDTH 00122 #define POK_TILE_W PROJ_TILEWIDTH 00123 #else 00124 #define POK_TILE_W 11 00125 #endif // PROJ_TILEWIDTH 00126 #if POK_TILE_W == 11 00127 #define POK_TILES_X 20 00128 #define LCDWIDTH 220 00129 #elif POK_TILE_W == 12 00130 #define POK_TILES_X 18 00131 #define LCDWIDTH 216 00132 #elif POK_TILE_W == 8 00133 #define POK_TILES_X 27 00134 #define LCDWIDTH 216 00135 #elif POK_TILE_W == 32 00136 #define POK_TILES_X 6 00137 #define LCDWIDTH 220 00138 #elif POK_TILE_W == 10 00139 #define POK_TILES_X 22 00140 #define LCDWIDTH 220 00141 #elif POK_TILE_W == 14 00142 #define POK_TILES_X 15 00143 #define LCDWIDTH 210 00144 #endif 00145 #ifdef PROJ_TILEHEIGHT 00146 #define POK_TILE_H PROJ_TILEHEIGHT 00147 #else 00148 #define POK_TILE_H 11 00149 #endif // PROJ_TILEHEIGHT 00150 #if POK_TILE_H == 11 00151 #define POK_TILES_Y 16 00152 #define LCDHEIGHT 176 00153 #elif POK_TILE_H == 12 00154 #define POK_TILES_Y 14 00155 #define LCDHEIGHT 168 00156 #elif POK_TILE_H == 8 00157 #define POK_TILES_Y 22 00158 #define LCDHEIGHT 176 00159 #elif POK_TILE_H == 32 00160 #define POK_TILES_Y 5 00161 #define LCDHEIGHT 176 00162 #elif POK_TILE_H == 10 00163 #define POK_TILES_Y 17 00164 #define LCDHEIGHT 170 00165 #elif POK_TILE_H == 14 00166 #define POK_TILES_Y 12 00167 #define LCDHEIGHT 168 00168 #endif 00169 #else 00170 #if PROJ_GAMEBUINO > 0 00171 #define POK_GAMEBUINO_SUPPORT PROJ_GAMEBUINO // Define true to support Gamebuino library calls 00172 #define PROJ_SCREENMODE MODE_GAMEBUINO_16COLOR 00173 #define POK_STRETCH 1 00174 #define PICOPALETTE 0 00175 #define POK_COLORDEPTH 4 00176 #else 00177 #if PROJ_ARDUBOY > 0 00178 #define POK_ARDUBOY_SUPPORT PROJ_ARDUBOY // Define true to support Arduboy library calls 00179 #define PROJ_SCREENMODE MODE_ARDUBOY_16COLOR 00180 #define POK_COLORDEPTH 1 00181 #define POK_STRETCH 1 00182 #define POK_FPS 20 00183 #define PICOPALETTE 0 00184 #else 00185 #if PROJ_RBOY > 0 00186 #define PROJ_SCREENMODE MODE_GAMEBUINO_16COLOR 00187 #define POK_COLORDEPTH 1 00188 #define POK_STRETCH 0 00189 #define POK_FPS 40 00190 #define PICOPALETTE 0 00191 #else 00192 #if PROJ_GAMEBOY > 0 00193 #define PROJ_SCREENMODE MODE_GAMEBOY 00194 #define POK_COLORDEPTH 2 00195 #define POK_STRETCH 0 00196 #define POK_FPS 6 00197 #define PICOPALETTE 0 00198 #else 00199 #define POK_GAMEBUINO_SUPPORT 0 00200 #define POK_GAMEBOY_SUPPORT 0 00201 #define POK_ARDUBOY_SUPPORT 0 00202 #define PICOPALETTE 0 00203 #define POK_COLORDEPTH 4 00204 #endif // PROJ_GAMEBOY 00205 #endif // PROJ_RBOY 00206 #endif // PROJ_ARDUBOY 00207 #endif // PROJ_GAMEBUINO 00208 #endif // PROJ_TILEDMODE 00209 00210 00211 /** SCREEN MODES TABLE -- DO NOT CHANGE THESE **/ 00212 00213 #define POK_LCD_W 220 //<- do not change !! 00214 #define POK_LCD_H 176 //<- do not change !! 00215 00216 #define MODE_NOBUFFER 0 //Size: 0 00217 #define BUFSIZE_NOBUFFER 0 00218 #define MODE_HI_4COLOR 1 //Size: 9680 00219 #define BUFSIZE_HI_4 9680 00220 #define MODE_FAST_16COLOR 2 //Size: 4840 00221 #define BUFSIZE_FAST_16 4840 00222 #define MODE_GAMEBUINO_16COLOR 4 //Size: 2016 00223 #define BUFSIZE_GAMEBUINO_16 2016 00224 #define MODE_ARDUBOY_16COLOR 5 //Size: 4096 00225 #define BUFSIZE_ARDUBOY_16 4096 00226 #define MODE_HI_MONOCHROME 6 //Size: 4840 00227 #define BUFSIZE_HI_MONO 4840 00228 #define MODE_HI_GRAYSCALE 7 //Size: 9680 00229 #define BUFSIZE_HI_GS 9680 00230 #define MODE_GAMEBOY 8 00231 #define BUFSIZE_GAMEBOY 5760 00232 #define MODE_UZEBOX 9 00233 #define MODE_TVOUT 10 00234 #define MODE_LAMENES 11 00235 #define BUFSIZE_LAMENES 7680 00236 #define MODE_256_COLOR 12 00237 #define BUFSIZE_MODE_12 4176 // 72 x 58 00238 #define MODE13 13 00239 #define BUFSIZE_MODE13 9680 // 110*88 00240 #define MIXMODE 32 00241 #define BUFSIZE_MIXMODE 9680 // 110*88 00242 #define MODE64 64 00243 #define BUFSIZE_MODE64 19360 // 110*176 00244 #define MODE14 14 00245 #define BUFSIZE_MODE14 14520 00246 #define MODE15 15 00247 #define BUFSIZE_MODE15 19360 00248 // Tiled modes 00249 #define MODE_TILED_1BIT 1001 00250 #define MODE_TILED_8BIT 1002 00251 00252 00253 #define R_MASK 0xF800 00254 #define G_MASK 0x7E0 00255 #define B_MASK 0x1F 00256 00257 /** SCREENMODE - USE THIS SELECTION FOR YOUR PROJECT **/ 00258 00259 #if POK_TILEDMODE > 0 00260 #ifndef PROJ_TILEBITDEPTH 00261 #define PROJ_TILEBITDEPTH 8 //default tiling mode is 256 color mode! 00262 #endif // PROJ_TILEBITDEPTH 00263 #if PROJ_TILEBITDEPTH == 1 00264 #define POK_SCREENMODE MODE_TILED_1BIT 00265 #define POK_COLORDEPTH 1 00266 #else 00267 #define POK_SCREENMODE MODE_TILED_8BIT 00268 #define POK_COLORDEPTH 8 00269 #endif // PROJ_TILEBITDEPTH 00270 #else 00271 #ifndef PROJ_SCREENMODE 00272 #undef POK_COLORDEPTH 00273 #ifdef PROJ_HIRES 00274 #if PROJ_HIRES > 0 00275 #define POK_SCREENMODE MODE_HI_4COLOR 00276 #undef POK_COLORDEPTH 00277 #define POK_COLORDEPTH 2 00278 #elif PROJ_HICOLOR > 0 00279 #define POK_SCREENMODE MODE_256_COLOR 00280 #undef POK_COLORDEPTH 00281 #define POK_COLORDEPTH 8 00282 #else 00283 #define POK_SCREENMODE MODE_FAST_16COLOR 00284 #undef POK_COLORDEPTH 00285 #define POK_COLORDEPTH 4 00286 #endif // PROJ_HIRES 00287 #else 00288 #define POK_SCREENMODE MODE_FAST_16COLOR 00289 #define POK_COLORDEPTH 4 00290 #endif // PROJ_HIRES 00291 #else 00292 #define POK_SCREENMODE PROJ_SCREENMODE 00293 #endif 00294 #endif // POK_TILEDMODE 00295 00296 #if PROJ_MODE13 > 0 || PROJ_SCREENMODE == 13 00297 #undef POK_SCREENMODE //get rid of warnings 00298 #undef POK_COLORDEPTH 00299 #undef POK_FPS 00300 #define POK_SCREENMODE MODE13 00301 #define POK_COLORDEPTH 8 00302 #define POK_STRETCH 0 00303 #define POK_FPS 30 00304 #endif 00305 00306 #if PROJ_MIXMODE > 0 00307 #undef POK_SCREENMODE //get rid of warnings 00308 #undef POK_COLORDEPTH 00309 #undef POK_FPS 00310 #define POK_SCREENMODE MIXMODE 00311 #define POK_COLORDEPTH 8 00312 #define POK_STRETCH 0 00313 #define POK_FPS 30 00314 #endif 00315 00316 00317 #if PROJ_MODE64 > 0 || PROJ_SCREENMODE == 64 00318 #undef POK_SCREENMODE //get rid of warnings 00319 #undef POK_COLORDEPTH 00320 #undef POK_FPS 00321 #define POK_SCREENMODE MODE64 00322 #define POK_COLORDEPTH 8 00323 #define POK_STRETCH 0 00324 #define POK_FPS 30 00325 #endif 00326 00327 #if PROJ_MODE14 > 0 || PROJ_SCREENMODE == 14 00328 #undef POK_SCREENMODE //get rid of warnings 00329 #undef POK_COLORDEPTH 00330 #undef POK_FPS 00331 #define POK_SCREENMODE MODE14 00332 #define POK_COLORDEPTH 3 00333 #define POK_STRETCH 0 00334 #define POK_FPS 30 00335 #endif 00336 #if PROJ_MODE15 > 0 || PROJ_SCREENMODE == 15 00337 #undef POK_SCREENMODE //get rid of warnings 00338 #undef POK_COLORDEPTH 00339 #undef POK_FPS 00340 #define POK_SCREENMODE MODE15 00341 #define POK_COLORDEPTH 4 00342 #define POK_STRETCH 0 00343 #define POK_FPS 30 00344 #define POK_BITFRAME 4840 00345 #endif 00346 /* DEFINE SCREENMODE AS THE MAXIMUM SCREEN SIZE NEEDED BY YOUR APP ... SEE SIZES LISTED ABOVE */ 00347 00348 /** AUTOMATIC COLOR DEPTH SETTING - DO NOT CHANGE **/ 00349 #ifndef POK_COLORDEPTH 00350 #define POK_COLORDEPTH 4 // 1...5 is valid 00351 #endif // POK_COLORDEPTH 00352 00353 /** AUTOMATIC SCREEN BUFFER SIZE CALCULATION - DO NOT CHANGE **/ 00354 #if POK_SCREENMODE == 0 00355 #define POK_SCREENBUFFERSIZE 0 00356 #define LCDWIDTH POK_LCD_W 00357 #define LCDHEIGHT POK_LCD_H 00358 #define POK_BITFRAME 0 00359 #elif POK_SCREENMODE == MODE_HI_MONOCHROME 00360 #define POK_SCREENBUFFERSIZE POK_LCD_W*POK_LCD_H*POK_COLORDEPTH/8 00361 #define LCDWIDTH POK_LCD_W 00362 #define LCDHEIGHT POK_LCD_H 00363 #define POK_BITFRAME 4840 00364 #elif POK_SCREENMODE == MODE_HI_4COLOR || POK_SCREENMODE == MODE_HI_GRAYSCALE 00365 #define POK_SCREENBUFFERSIZE (POK_LCD_W*POK_LCD_H*POK_COLORDEPTH/4) 00366 #define LCDWIDTH POK_LCD_W 00367 #define LCDHEIGHT POK_LCD_H 00368 #define POK_BITFRAME 4840 00369 #elif POK_SCREENMODE == MODE_FAST_16COLOR 00370 #define POK_SCREENBUFFERSIZE (POK_LCD_W/2)*(POK_LCD_H/2)*POK_COLORDEPTH/8 00371 #define XCENTER POK_LCD_W/4 00372 #define YCENTER POK_LCD_H/4 00373 #define LCDWIDTH 110 00374 #define LCDHEIGHT 88 00375 #define POK_BITFRAME 1210 00376 #elif POK_SCREENMODE == MODE_256_COLOR 00377 #define POK_SCREENBUFFERSIZE 72*58 00378 #define XCENTER 36 00379 #define YCENTER 29 00380 #define LCDWIDTH 72 00381 #define LCDHEIGHT 58 00382 #define POK_BITFRAME 72*58 00383 #elif POK_SCREENMODE == MODE_GAMEBUINO_16COLOR 00384 #define POK_SCREENBUFFERSIZE (84/2)*(48/2)*POK_COLORDEPTH/8 00385 #define LCDWIDTH 84 00386 #define LCDHEIGHT 48 00387 #define POK_BITFRAME 504 00388 #elif POK_SCREENMODE == MODE_ARDUBOY_16COLOR 00389 #define POK_SCREENBUFFERSIZE (128/2)*(64/2)*POK_COLORDEPTH/8 00390 #define LCDWIDTH 128 00391 #define LCDHEIGHT 64 00392 #define POK_BITFRAME 1024 00393 #elif POK_SCREENMODE == MODE_LAMENES 00394 #define POK_SCREENBUFFERSIZE (128)*(120)*POK_COLORDEPTH/8 00395 #define LCDWIDTH 128 00396 #define LCDHEIGHT 120 00397 #define POK_BITFRAME 1210 00398 #elif POK_SCREENMODE == MODE_GAMEBOY 00399 #define POK_SCREENBUFFERSIZE (160)*(144)/4 00400 #define LCDWIDTH 160 00401 #define LCDHEIGHT 144 00402 #define POK_BITFRAME 2880 00403 #elif POK_SCREENMODE == MODE13 00404 #define POK_SCREENBUFFERSIZE 110*88 00405 #define LCDWIDTH 110 00406 #define LCDHEIGHT 88 00407 #define POK_BITFRAME 110*88 00408 #elif POK_SCREENMODE == MIXMODE 00409 #define POK_SCREENBUFFERSIZE 110*88 00410 #define LCDWIDTH 110 00411 #define LCDHEIGHT 88 00412 #define POK_BITFRAME 110*88 00413 #elif POK_SCREENMODE == MODE64 00414 #define POK_SCREENBUFFERSIZE 110*176 00415 #define LCDWIDTH 110 00416 #define LCDHEIGHT 176 00417 #define POK_BITFRAME 110*176 00418 #elif POK_SCREENMODE == MODE14 00419 #define POK_SCREENBUFFERSIZE 14520 00420 #define LCDWIDTH 220 00421 #define LCDHEIGHT 176 00422 #define POK_BITFRAME 4840 00423 #elif POK_SCREENMODE == MODE15 00424 #define POK_SCREENBUFFERSIZE 0x4BA0 00425 #define LCDWIDTH 220 00426 #define LCDHEIGHT 176 00427 00428 #else 00429 #define POK_SCREENBUFFERSIZE 0 00430 #endif // POK_SCREENMODE 00431 00432 #ifndef POK_STRETCH 00433 #define POK_STRETCH 1 // Stretch Gamebuino display 00434 #endif 00435 00436 #ifdef PROJ_FPS 00437 #define POK_FPS PROJ_FPS 00438 #endif 00439 #ifndef POK_FPS 00440 #define POK_FPS 20 00441 #endif 00442 #define POK_FRAMEDURATION 1000/POK_FPS 00443 00444 /** SCROLL TEXT VS. WRAP AROUND WHEN PRINTING **/ 00445 #if PROJ_NO_AUTO_SCROLL 00446 #define SCROLL_TEXT 0 00447 #else 00448 #define SCROLL_TEXT 1 00449 #endif 00450 00451 /** AUDIO **/ 00452 00453 #define POK_ALT_MIXING 1 // NEW! alternative more accurate mixing, uses more CPU 00454 00455 #define POK_AUD_PIN P2_19 00456 #define POK_AUD_PWM_US 15 //31 //Default value 31 00457 #ifndef PROJ_AUD_FREQ 00458 #define POK_AUD_FREQ 22050 //Valid values: 8000, 11025, 16000, 22050 // audio update frequency in Hz 00459 #else 00460 #define POK_AUD_FREQ PROJ_AUD_FREQ 00461 #endif 00462 00463 00464 #define POK_USE_EXT 0 // if extension port is in use or not 00465 00466 #define POK_STREAMFREQ_HALVE 0 // if true, stream update freq is half audio freq 00467 #define POK_STREAM_LOOP 1 //master switch 00468 00469 #ifndef PROJ_USE_DAC 00470 #define POK_USE_DAC 1 // is DAC in use in this project 00471 #else 00472 #define POK_USE_DAC PROJ_USE_DAC 00473 #endif 00474 #ifndef PROJ_USE_PWM 00475 #define POK_USE_PWM 1 // is PWM for audio used in this project 00476 #else 00477 #define POK_USE_PWM PROJ_USE_PWM 00478 #endif 00479 00480 #ifndef PROJ_STREAM_TO_DAC 00481 #define POK_STREAM_TO_DAC 1 // 1 = stream from SD to DAC, synthesizer to PWM, 0 = opposite 00482 #else 00483 #define POK_STREAM_TO_DAC PROJ_STREAM_TO_DAC 00484 #endif 00485 00486 00487 #define POK_BACKLIGHT_PIN P2_2 00488 #define POK_BACKLIGHT_INITIALVALUE 0.3f 00489 00490 #define POK_BATTERY_PIN1 P0_22 // read battery level through these pins 00491 #define POK_BATTERY_PIN2 P0_23 00492 00493 #define POK_BTN_A_PIN P1_9 00494 #define POK_BTN_B_PIN P1_4 00495 #define POK_BTN_C_PIN P1_10 00496 #define POK_BTN_UP_PIN P1_13 00497 #define POK_BTN_DOWN_PIN P1_3 00498 #define POK_BTN_LEFT_PIN P1_25 00499 #define POK_BTN_RIGHT_PIN P1_7 00500 00501 #define UPBIT 0 00502 #define DOWNBIT 1 00503 #define LEFTBIT 2 00504 #define RIGHTBIT 3 00505 #define ABIT 4 00506 #define BBIT 5 00507 #define CBIT 6 00508 00509 /** LOADER UPDATE MECHANISM **/ 00510 #define POK_ENABLE_LOADER_UPDATES 1 //1=check for new loader versions on SD and update if new found 00511 00512 #ifndef SPRITE_COUNT 00513 #define SPRITE_COUNT 4 // The default max sprite count 00514 #endif 00515 00516 /** SYSTEM SETTINGS ADDRESSES IN EEPROM **/ 00517 #define EESETTINGS_FILENAME 3980 // 0xF8C 20bytes last filename requested 00518 #define EESETTINGS_VOL 4000 // 0xFA0 Volume 00519 #define EESETTINGS_DEFAULTVOL 4001 // 0xFA1 Default volume 00520 #define EESETTINGS_LOADERWAIT 4002 // 0xFA2 Loader wait in sec 00521 #define EESETTINGS_VOLWAIT 4003 // 0xFA3 Volume screen wait in sec 00522 #define EESETTINGS_TIMEFORMAT 4004 // 0xFA4 Time format (0=24 hrs, 1 = 12 hrs) 00523 #define EESETTINGS_LASTHOURSSET 4005 // 0xFA5 Last time set in hours 00524 #define EESETTINGS_LASTMINUTESSET 4006 // 0xFA6 Last time set in minutes 00525 #define EESETTINGS_DATEFORMAT 4007 // 0xFA7 Date format (0=D/M/Y, 1 = M/D/Y) 00526 #define EESETTINGS_LASTDAYSET 4008 // 0xFA8 Last Day set 00527 #define EESETTINGS_LASTMONTHSET 4009 // 0xFA9 Last Month set 00528 #define EESETTINGS_LASTYEARSET 4010 // 0xFAA Last Year set (counting from 2000) 00529 #define EESETTINGS_RTCALARMMODE 4011 // 0xFAB RTC alarm mode (0=disabled, 1=enabled, 3 = enabled with sound) 00530 #define EESETTINGS_RESERVED 4012 // 0xFAC 4bytes reserved (additional sleep configuration) 00531 #define EESETTINGS_WAKEUPTIME 4016 // 0xFB0 Wake-up time as 32bit value for 1Hz RTC clock 00532 #define EESETTINGS_SKIPINTRO 4020 // 0xFB4 Show Logo (0-Yes, 1-No, 2-Yes then switch to 0, 3-No, then switch to 1) 00533 00534 /** USB SERIAL PORT **/ 00535 00536 #ifndef PROJ_VENDOR_ID 00537 #define POK_VENDOR_ID 0x04D8 00538 #define POK_PRODUCT_ID 0x000A 00539 #endif 00540 00541 00542 #endif // POKITTO_SETTINGS_H 00543 00544
Generated on Tue Jul 12 2022 11:20:34 by
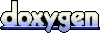