PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
PokittoConsole.h
00001 /**************************************************************************/ 00002 /*! 00003 @file PokittoConsole.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #ifndef POKITTO_CONSOLE_H 00038 #define POKITTO_CONSOLE_H 00039 00040 #include "Pokitto_settings.h " 00041 #include "PokittoButtons.h " 00042 #include <stdint.h> 00043 00044 struct consmsg { 00045 uint32_t val; 00046 uint8_t valtype; 00047 uint8_t msgsource; 00048 uint8_t msgtype; 00049 }; 00050 00051 /** VAL TYPES **/ 00052 #define V_UINT8 0 00053 #define V_INT8 1 00054 #define V_UINT16 2 00055 #define V_INT16 3 00056 #define V_UINT32 4 00057 #define V_INT32 5 00058 #define V_FLOAT 6 00059 #define V_STRING 7 00060 #define V_NONE 8 00061 00062 /** MESSAGE TYPE **/ 00063 #define MSG_NULL 0 00064 #define MSG_OK 1 00065 #define MSG_WARNING 2 00066 #define MSG_FATAL 255 00067 #define MSG_INIT_OK 3 00068 #define MSG_INIT_FAIL 4 00069 #define MSG_NOT_ENOUGH_MEM 5 00070 #define MSG_GFX_MODE_CHANGE 6 00071 #define MSG_GFX_MODE_INVALID 7 00072 #define MSG_UP 8 00073 #define MSG_DOWN 9 00074 #define MSG_PRINT 10 00075 #define MSG_BREAK 11 00076 #define MSG_YESNO 12 00077 #define MSG_YES 13 00078 #define MSG_NO 14 00079 #define MSG_OBJECT 15 00080 #define MSG_OBJECT2 16 00081 00082 /** MESSAGE SOURCE **/ 00083 #define MSOURCE_NULL 0 00084 #define MSOURCE_SD 1 00085 #define MSOURCE_LCD 2 00086 #define MSOURCE_SOUND 3 00087 #define MSOURCE_TIMER 4 00088 #define MSOURCE_BTNA 5 00089 #define MSOURCE_BTNB 6 00090 #define MSOURCE_BTNC 7 00091 #define MSOURCE_BTNU 8 00092 #define MSOURCE_BTND 9 00093 #define MSOURCE_BTNL 10 00094 #define MSOURCE_BTNR 11 00095 #define MSOURCE_BATT 12 00096 #define MSOURCE_APP 13 00097 #define MSOURCE_USER 14 00098 #define MSOURCE_COLLISION 15 00099 00100 /** CONSOLE MODES **/ 00101 #define CONS_OVERLAY 0x1 00102 #define CONS_PAUSE 0x2 00103 #define CONS_STEP 0x4 00104 #define CONS_VISIBLE 0x8 00105 00106 00107 /** CONSOLE DEBOUNCE **/ 00108 #define CONS_TIMEOUT 20 00109 extern uint16_t conscounter; 00110 00111 00112 00113 namespace Pokitto { 00114 00115 class Display; 00116 00117 class Console { 00118 public: 00119 /** Console class constructor */ 00120 Console(); 00121 00122 static uint8_t mode; 00123 static uint8_t visible; 00124 static unsigned char* font; 00125 static void Toggle(); 00126 static void AddMessage(uint8_t, uint8_t, uint8_t, uint32_t); 00127 static void AddMessage(uint8_t, uint8_t); 00128 static void AddMessage(uint8_t, char*); 00129 static void AddMessage(uint8_t, uint8_t, uint32_t); 00130 static void Last(); 00131 static void First(); 00132 static void RemoveLast(); 00133 static void Previous(); 00134 static void Next(); 00135 static consmsg GetMessage(); 00136 static void PrintMessage(); 00137 static void Purge(); 00138 static void Draw(); // pokConsole 00139 00140 private: 00141 /** Message buffer */ 00142 static consmsg msgbuf[CONSOLEBUFSIZE]; 00143 static uint8_t conslast; 00144 static uint8_t consfirst; 00145 static uint8_t conspointer; 00146 public: 00147 static uint16_t conscounter; 00148 static uint16_t color; 00149 static Display* _display; 00150 static Buttons* _buttons; 00151 }; 00152 00153 00154 } // namespace 00155 00156 // this is the console used by the core if POK_USE_CONSOLE is nonzero 00157 extern Pokitto::Console console; 00158 00159 #endif // POKITTO_CONSOLE_H 00160 00161
Generated on Tue Jul 12 2022 11:20:35 by
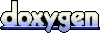