A PPM driver for the K64F that uses the CMT module
Embed:
(wiki syntax)
Show/hide line numbers
PPMout.h
Go to the documentation of this file.
00001 /** 00002 * @file PPMout.h 00003 * @brief PPMout - PPM signal generator for K64F 00004 * @author Patrick Thomas 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2016 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef PPMOUT_H 00024 #define PPMOUT_H 00025 00026 #include "mbed.h" 00027 00028 #define PIN(n,min,max) ((n) > (max) ? max : ((n) < (min) ? (min) : (n))) 00029 00030 // System clock 00031 #define BUS_CLOCK 60000000 00032 #define CLOCK_DIVIDER 12 00033 #define CMT_PPS_VAL (CLOCK_DIVIDER - 1) 00034 00035 // Step array 00036 #define CHANNELS 8 00037 #define ARRAY_SIZE (CHANNELS + 1) 00038 00039 // Time parameters 00040 #define STEPS_PER_MS (((BUS_CLOCK/CLOCK_DIVIDER)/8)/1000) 00041 #define GAP_LENGTH_MS 0.3 00042 #define FRAME_LENGTH_MS 22.5 00043 00044 // PPM parameters 00045 #define PPM_RANGE_MS 1 00046 #define PPM_ZERO_MS 0.7 00047 00048 // Derived step parameters 00049 #define RANGE_STEPS (PPM_RANGE_MS*STEPS_PER_MS) 00050 #define ZERO_STEPS (PPM_ZERO_MS*STEPS_PER_MS) 00051 #define GAP_STEPS (int) (GAP_LENGTH_MS*STEPS_PER_MS) 00052 #define TOTAL_GAP_STEPS (GAP_STEPS*ARRAY_SIZE) 00053 #define FRAME_STEPS (FRAME_LENGTH_MS*STEPS_PER_MS) 00054 #define MAX_NON_GAP_STEPS (FRAME_STEPS - TOTAL_GAP_STEPS) 00055 00056 /** A library for producing PPM output on the K64F (pin PTD7) using the CMT (Carrier/Modulator Transmitter) module 00057 * 00058 * Example: 00059 * @code 00060 * #include "mbed.h" 00061 * #include "PPMout.h" 00062 * 00063 * PPMout myPPM; 00064 * Serial pc(USBTX, USBRX); 00065 * 00066 * int main() 00067 * { 00068 * while(1) { 00069 * 00070 * char buffer[128]; 00071 * float f; 00072 * 00073 * pc.gets(buffer, 5); 00074 * f = (float) atof(buffer); 00075 * 00076 * myPPM.update_channel(0, f); 00077 * } 00078 * } 00079 * @endcode 00080 */ 00081 00082 class PPMout { 00083 00084 private: 00085 00086 static int count_array[ARRAY_SIZE]; 00087 static int counter; 00088 00089 static void CMT_IRQHandler(); 00090 void init(); 00091 void set_sync_length(); 00092 00093 public: 00094 00095 /** Create PPMout instance 00096 */ 00097 PPMout(); 00098 00099 /** Update a PPM channel value 00100 * @param channel The channel to update (0-7) 00101 * @param value The new value for the channel (0-1) 00102 */ 00103 void update_channel(int channel, float value); 00104 }; 00105 00106 #endif
Generated on Wed Jul 27 2022 02:27:13 by
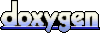