Embed:
(wiki syntax)
Show/hide line numbers
GetTickCount.h
00001 /* mbed GetTickCount Library 00002 * Copyright (c) 2010 Michael Wei 00003 */ 00004 00005 //shouldn't have to include, but fixes weird problems with defines 00006 #include "LPC1768/LPC17xx.h" 00007 00008 #ifndef MBED_TICKCOUNT_H 00009 #define MBED_TICKCOUNT_H 00010 extern volatile unsigned int TickCount; 00011 00012 inline void GetTickCount_Start(void) { 00013 //CMSIS SYSTICK Config 00014 SysTick_Config(SystemCoreClock / 100); /* Generate interrupt every 10 ms */ 00015 } 00016 00017 inline void GetTickCount_Stop(void) { 00018 SysTick->CTRL = (1 << SYSTICK_CLKSOURCE) | (0<<SYSTICK_ENABLE) | (0<<SYSTICK_TICKINT); /* Disable SysTick IRQ and SysTick Timer */ 00019 } 00020 00021 inline void GetTickCount_Reset(void) { 00022 SysTick->CTRL = (1 << SYSTICK_CLKSOURCE) | (0<<SYSTICK_ENABLE) | (0<<SYSTICK_TICKINT); /* Disable SysTick IRQ and SysTick Timer */ 00023 TickCount = 0; 00024 SysTick->CTRL = (1 << SYSTICK_CLKSOURCE) | (1<<SYSTICK_ENABLE) | (1<<SYSTICK_TICKINT); /* Enable SysTick IRQ and SysTick Timer */ 00025 } 00026 00027 inline unsigned int GetTickCount(void) 00028 { 00029 return TickCount; 00030 } 00031 #endif
Generated on Fri Jul 15 2022 14:31:34 by
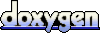