
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBRegs_STM32.h
00001 /** 00002 ****************************************************************************** 00003 * @file usb_regs.h 00004 * @author MCD Application Team 00005 * @version V2.1.0 00006 * @date 19-March-2012 00007 * @brief hardware registers 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT 2012 STMicroelectronics</center></h2> 00012 * 00013 * Licensed under MCD-ST Liberty SW License Agreement V2, (the "License"); 00014 * You may not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at: 00016 * 00017 * http://www.st.com/software_license_agreement_liberty_v2 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, 00021 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 ****************************************************************************** 00026 */ 00027 00028 #ifndef __USB_OTG_REGS_H__ 00029 #define __USB_OTG_REGS_H__ 00030 00031 typedef struct //000h 00032 { 00033 __IO uint32_t GOTGCTL; /* USB_OTG Control and Status Register 000h*/ 00034 __IO uint32_t GOTGINT; /* USB_OTG Interrupt Register 004h*/ 00035 __IO uint32_t GAHBCFG; /* Core AHB Configuration Register 008h*/ 00036 __IO uint32_t GUSBCFG; /* Core USB Configuration Register 00Ch*/ 00037 __IO uint32_t GRSTCTL; /* Core Reset Register 010h*/ 00038 __IO uint32_t GINTSTS; /* Core Interrupt Register 014h*/ 00039 __IO uint32_t GINTMSK; /* Core Interrupt Mask Register 018h*/ 00040 __IO uint32_t GRXSTSR; /* Receive Sts Q Read Register 01Ch*/ 00041 __IO uint32_t GRXSTSP; /* Receive Sts Q Read & POP Register 020h*/ 00042 __IO uint32_t GRXFSIZ; /* Receive FIFO Size Register 024h*/ 00043 __IO uint32_t DIEPTXF0_HNPTXFSIZ; /* EP0 / Non Periodic Tx FIFO Size Register 028h*/ 00044 __IO uint32_t HNPTXSTS; /* Non Periodic Tx FIFO/Queue Sts reg 02Ch*/ 00045 uint32_t Reserved30[2]; /* Reserved 030h*/ 00046 __IO uint32_t GCCFG; /* General Purpose IO Register 038h*/ 00047 __IO uint32_t CID; /* User ID Register 03Ch*/ 00048 uint32_t Reserved40[48]; /* Reserved 040h-0FFh*/ 00049 __IO uint32_t HPTXFSIZ; /* Host Periodic Tx FIFO Size Reg 100h*/ 00050 __IO uint32_t DIEPTXF[3];/* dev Periodic Transmit FIFO */ 00051 } 00052 USB_OTG_GREGS; 00053 00054 typedef struct // 800h 00055 { 00056 __IO uint32_t DCFG; /* dev Configuration Register 800h*/ 00057 __IO uint32_t DCTL; /* dev Control Register 804h*/ 00058 __IO uint32_t DSTS; /* dev Status Register (RO) 808h*/ 00059 uint32_t Reserved0C; /* Reserved 80Ch*/ 00060 __IO uint32_t DIEPMSK; /* dev IN Endpoint Mask 810h*/ 00061 __IO uint32_t DOEPMSK; /* dev OUT Endpoint Mask 814h*/ 00062 __IO uint32_t DAINT; /* dev All Endpoints Itr Reg 818h*/ 00063 __IO uint32_t DAINTMSK; /* dev All Endpoints Itr Mask 81Ch*/ 00064 uint32_t Reserved20; /* Reserved 820h*/ 00065 uint32_t Reserved9; /* Reserved 824h*/ 00066 __IO uint32_t DVBUSDIS; /* dev VBUS discharge Register 828h*/ 00067 __IO uint32_t DVBUSPULSE; /* dev VBUS Pulse Register 82Ch*/ 00068 __IO uint32_t DTHRCTL; /* dev thr 830h*/ 00069 __IO uint32_t DIEPEMPMSK; /* dev empty msk 834h*/ 00070 } 00071 USB_OTG_DREGS; 00072 00073 typedef struct 00074 { 00075 __IO uint32_t DIEPCTL; /* dev IN Endpoint Control Reg 900h + (ep_num * 20h) + 00h*/ 00076 uint32_t Reserved04; /* Reserved 900h + (ep_num * 20h) + 04h*/ 00077 __IO uint32_t DIEPINT; /* dev IN Endpoint Itr Reg 900h + (ep_num * 20h) + 08h*/ 00078 uint32_t Reserved0C; /* Reserved 900h + (ep_num * 20h) + 0Ch*/ 00079 __IO uint32_t DIEPTSIZ; /* IN Endpoint Txfer Size 900h + (ep_num * 20h) + 10h*/ 00080 uint32_t Reserved14; 00081 __IO uint32_t DTXFSTS;/*IN Endpoint Tx FIFO Status Reg 900h + (ep_num * 20h) + 18h*/ 00082 uint32_t Reserved1C; /* Reserved 900h+(ep_num*20h)+1Ch-900h+ (ep_num * 20h) + 1Ch*/ 00083 } 00084 USB_OTG_INEPREGS; 00085 00086 typedef struct 00087 { 00088 __IO uint32_t DOEPCTL; /* dev OUT Endpoint Control Reg B00h + (ep_num * 20h) + 00h*/ 00089 uint32_t Reserved04; /* Reserved B00h + (ep_num * 20h) + 04h*/ 00090 __IO uint32_t DOEPINT; /* dev OUT Endpoint Itr Reg B00h + (ep_num * 20h) + 08h*/ 00091 uint32_t Reserved0C; /* Reserved B00h + (ep_num * 20h) + 0Ch*/ 00092 __IO uint32_t DOEPTSIZ; /* dev OUT Endpoint Txfer Size B00h + (ep_num * 20h) + 10h*/ 00093 uint32_t Reserved14[3]; 00094 } 00095 USB_OTG_OUTEPREGS; 00096 00097 typedef struct 00098 { 00099 __IO uint32_t HCFG; /* Host Configuration Register 400h*/ 00100 __IO uint32_t HFIR; /* Host Frame Interval Register 404h*/ 00101 __IO uint32_t HFNUM; /* Host Frame Nbr/Frame Remaining 408h*/ 00102 uint32_t Reserved40C; /* Reserved 40Ch*/ 00103 __IO uint32_t HPTXSTS; /* Host Periodic Tx FIFO/ Queue Status 410h*/ 00104 __IO uint32_t HAINT; /* Host All Channels Interrupt Register 414h*/ 00105 __IO uint32_t HAINTMSK; /* Host All Channels Interrupt Mask 418h*/ 00106 } 00107 USB_OTG_HREGS; 00108 00109 typedef struct 00110 { 00111 __IO uint32_t HCCHAR; 00112 __IO uint32_t HCSPLT; 00113 __IO uint32_t HCINT; 00114 __IO uint32_t HCINTMSK; 00115 __IO uint32_t HCTSIZ; 00116 uint32_t Reserved[3]; 00117 } 00118 USB_OTG_HC_REGS; 00119 00120 typedef struct 00121 { 00122 USB_OTG_GREGS GREGS; 00123 uint32_t RESERVED0[188]; 00124 USB_OTG_HREGS HREGS; 00125 uint32_t RESERVED1[9]; 00126 __IO uint32_t HPRT; 00127 uint32_t RESERVED2[47]; 00128 USB_OTG_HC_REGS HC_REGS[8]; 00129 uint32_t RESERVED3[128]; 00130 USB_OTG_DREGS DREGS; 00131 uint32_t RESERVED4[50]; 00132 USB_OTG_INEPREGS INEP_REGS[4]; 00133 uint32_t RESERVED5[96]; 00134 USB_OTG_OUTEPREGS OUTEP_REGS[4]; 00135 uint32_t RESERVED6[160]; 00136 __IO uint32_t PCGCCTL; 00137 uint32_t RESERVED7[127]; 00138 __IO uint32_t FIFO[4][1024]; 00139 } 00140 USB_OTG_CORE_REGS; 00141 00142 00143 #define OTG_FS_BASE (AHB2PERIPH_BASE + 0x0000) 00144 #define OTG_FS ((USB_OTG_CORE_REGS *) OTG_FS_BASE) 00145 00146 #endif //__USB_OTG_REGS_H__ 00147 00148 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00149
Generated on Tue Jul 12 2022 16:20:44 by
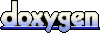