
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBHAL.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBBUSINTERFACE_H 00020 #define USBBUSINTERFACE_H 00021 00022 #include "mbed.h" 00023 #include "USBEndpoints.h" 00024 #include "toolchain.h" 00025 00026 //#ifdef __GNUC__ 00027 //#define __packed __attribute__ ((__packed__)) 00028 //#endif 00029 00030 class USBHAL { 00031 public: 00032 /* Configuration */ 00033 USBHAL(); 00034 ~USBHAL(); 00035 void connect(void); 00036 void disconnect(void); 00037 void configureDevice(void); 00038 void unconfigureDevice(void); 00039 void setAddress(uint8_t address); 00040 void remoteWakeup(void); 00041 00042 /* Endpoint 0 */ 00043 void EP0setup(uint8_t *buffer); 00044 void EP0read(void); 00045 void EP0readStage(void); 00046 uint32_t EP0getReadResult(uint8_t *buffer); 00047 void EP0write(uint8_t *buffer, uint32_t size); 00048 void EP0getWriteResult(void); 00049 void EP0stall(void); 00050 00051 /* Other endpoints */ 00052 EP_STATUS endpointRead(uint8_t endpoint, uint32_t maximumSize); 00053 EP_STATUS endpointReadResult(uint8_t endpoint, uint8_t *data, uint32_t *bytesRead); 00054 EP_STATUS endpointWrite(uint8_t endpoint, uint8_t *data, uint32_t size); 00055 EP_STATUS endpointWriteResult(uint8_t endpoint); 00056 void stallEndpoint(uint8_t endpoint); 00057 void unstallEndpoint(uint8_t endpoint); 00058 bool realiseEndpoint(uint8_t endpoint, uint32_t maxPacket, uint32_t options); 00059 bool getEndpointStallState(unsigned char endpoint); 00060 uint32_t endpointReadcore(uint8_t endpoint, uint8_t *buffer); 00061 00062 protected: 00063 virtual void busReset(void){}; 00064 virtual void EP0setupCallback(void){}; 00065 virtual void EP0out(void){}; 00066 virtual void EP0in(void){}; 00067 virtual void connectStateChanged(unsigned int connected){}; 00068 virtual void suspendStateChanged(unsigned int suspended){}; 00069 virtual void SOF(int frameNumber){}; 00070 00071 virtual bool EP1_OUT_callback(){return false;}; 00072 virtual bool EP1_IN_callback(){return false;}; 00073 virtual bool EP2_OUT_callback(){return false;}; 00074 virtual bool EP2_IN_callback(){return false;}; 00075 virtual bool EP3_OUT_callback(){return false;}; 00076 virtual bool EP3_IN_callback(){return false;}; 00077 #if !defined(TARGET_STM32F4) 00078 virtual bool EP4_OUT_callback(){return false;}; 00079 virtual bool EP4_IN_callback(){return false;}; 00080 #if !defined(TARGET_LPC11U24) 00081 virtual bool EP5_OUT_callback(){return false;}; 00082 virtual bool EP5_IN_callback(){return false;}; 00083 virtual bool EP6_OUT_callback(){return false;}; 00084 virtual bool EP6_IN_callback(){return false;}; 00085 virtual bool EP7_OUT_callback(){return false;}; 00086 virtual bool EP7_IN_callback(){return false;}; 00087 virtual bool EP8_OUT_callback(){return false;}; 00088 virtual bool EP8_IN_callback(){return false;}; 00089 virtual bool EP9_OUT_callback(){return false;}; 00090 virtual bool EP9_IN_callback(){return false;}; 00091 virtual bool EP10_OUT_callback(){return false;}; 00092 virtual bool EP10_IN_callback(){return false;}; 00093 virtual bool EP11_OUT_callback(){return false;}; 00094 virtual bool EP11_IN_callback(){return false;}; 00095 virtual bool EP12_OUT_callback(){return false;}; 00096 virtual bool EP12_IN_callback(){return false;}; 00097 virtual bool EP13_OUT_callback(){return false;}; 00098 virtual bool EP13_IN_callback(){return false;}; 00099 virtual bool EP14_OUT_callback(){return false;}; 00100 virtual bool EP14_IN_callback(){return false;}; 00101 virtual bool EP15_OUT_callback(){return false;}; 00102 virtual bool EP15_IN_callback(){return false;}; 00103 #endif 00104 #endif 00105 00106 private: 00107 void usbisr(void); 00108 static void _usbisr(void); 00109 static USBHAL * instance; 00110 00111 #if defined(TARGET_LPC11U24) 00112 bool (USBHAL::*epCallback[10 - 2])(void); 00113 #elif defined(TARGET_STM32F4XX) 00114 bool (USBHAL::*epCallback[8 - 2])(void); 00115 #else 00116 bool (USBHAL::*epCallback[32 - 2])(void); 00117 #endif 00118 00119 00120 }; 00121 #endif
Generated on Tue Jul 12 2022 16:20:43 by
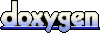