
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBDevice.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBDEVICE_H 00020 #define USBDEVICE_H 00021 00022 #include "mbed.h" 00023 #include "USBDevice_Types.h" 00024 #include "USBHAL.h" 00025 00026 class USBDevice: public USBHAL 00027 { 00028 public: 00029 USBDevice(uint16_t vendor_id, uint16_t product_id, uint16_t product_release); 00030 00031 /* 00032 * Check if the device is configured 00033 * 00034 * @returns true if configured, false otherwise 00035 */ 00036 bool configured(void); 00037 00038 /* 00039 * Connect a device 00040 * 00041 * @param blocking: block if not configured 00042 */ 00043 void connect(bool blocking = true); 00044 00045 /* 00046 * Disconnect a device 00047 */ 00048 void disconnect(void); 00049 00050 /* 00051 * Add an endpoint 00052 * 00053 * @param endpoint endpoint which will be added 00054 * @param maxPacket Maximum size of a packet which can be sent for this endpoint 00055 * @returns true if successful, false otherwise 00056 */ 00057 bool addEndpoint(uint8_t endpoint, uint32_t maxPacket); 00058 00059 /* 00060 * Start a reading on a certain endpoint. 00061 * You can access the result of the reading by USBDevice_read 00062 * 00063 * @param endpoint endpoint which will be read 00064 * @param maxSize the maximum length that can be read 00065 * @return true if successful 00066 */ 00067 bool readStart(uint8_t endpoint, uint32_t maxSize); 00068 00069 /* 00070 * Read a certain endpoint. Before calling this function, USBUSBDevice_readStart 00071 * must be called. 00072 * 00073 * Warning: blocking 00074 * 00075 * @param endpoint endpoint which will be read 00076 * @param buffer buffer will be filled with the data received 00077 * @param size the number of bytes read will be stored in *size 00078 * @param maxSize the maximum length that can be read 00079 * @returns true if successful 00080 */ 00081 bool readEP(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00082 00083 /* 00084 * Read a certain endpoint. 00085 * 00086 * Warning: non blocking 00087 * 00088 * @param endpoint endpoint which will be read 00089 * @param buffer buffer will be filled with the data received (if data are available) 00090 * @param size the number of bytes read will be stored in *size 00091 * @param maxSize the maximum length that can be read 00092 * @returns true if successful 00093 */ 00094 bool readEP_NB(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00095 00096 /* 00097 * Write a certain endpoint. 00098 * 00099 * Warning: blocking 00100 * 00101 * @param endpoint endpoint to write 00102 * @param buffer data contained in buffer will be write 00103 * @param size the number of bytes to write 00104 * @param maxSize the maximum length that can be written on this endpoint 00105 */ 00106 bool write(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00107 00108 00109 /* 00110 * Write a certain endpoint. 00111 * 00112 * Warning: non blocking 00113 * 00114 * @param endpoint endpoint to write 00115 * @param buffer data contained in buffer will be write 00116 * @param size the number of bytes to write 00117 * @param maxSize the maximum length that can be written on this endpoint 00118 */ 00119 bool writeNB(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00120 00121 00122 /* 00123 * Called by USBDevice layer on bus reset. Warning: Called in ISR context 00124 * 00125 * May be used to reset state 00126 */ 00127 virtual void USBCallback_busReset(void) {}; 00128 00129 /* 00130 * Called by USBDevice on Endpoint0 request. Warning: Called in ISR context 00131 * This is used to handle extensions to standard requests 00132 * and class specific requests 00133 * 00134 * @returns true if class handles this request 00135 */ 00136 virtual bool USBCallback_request() { return false; }; 00137 00138 /* 00139 * Called by USBDevice on Endpoint0 request completion 00140 * if the 'notify' flag has been set to true. Warning: Called in ISR context 00141 * 00142 * In this case it is used to indicate that a HID report has 00143 * been received from the host on endpoint 0 00144 * 00145 * @param buf buffer received on endpoint 0 00146 * @param length length of this buffer 00147 */ 00148 virtual void USBCallback_requestCompleted(uint8_t * buf, uint32_t length) {}; 00149 00150 /* 00151 * Called by USBDevice layer. Set configuration of the device. 00152 * For instance, you can add all endpoints that you need on this function. 00153 * 00154 * @param configuration Number of the configuration 00155 */ 00156 virtual bool USBCallback_setConfiguration(uint8_t configuration) { return false; }; 00157 00158 /* 00159 * Called by USBDevice layer. Set interface/alternate of the device. 00160 * 00161 * @param interface Number of the interface to be configured 00162 * @param alternate Number of the alternate to be configured 00163 * @returns true if class handles this request 00164 */ 00165 virtual bool USBCallback_setInterface(uint16_t interface, uint8_t alternate) { return false; }; 00166 00167 /* 00168 * Get device descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00169 * 00170 * @returns pointer to the device descriptor 00171 */ 00172 virtual uint8_t * deviceDesc(); 00173 00174 /* 00175 * Get configuration descriptor 00176 * 00177 * @returns pointer to the configuration descriptor 00178 */ 00179 virtual uint8_t * configurationDesc(){return NULL;}; 00180 00181 /* 00182 * Get string lang id descriptor 00183 * 00184 * @return pointer to the string lang id descriptor 00185 */ 00186 virtual uint8_t * stringLangidDesc(); 00187 00188 /* 00189 * Get string manufacturer descriptor 00190 * 00191 * @returns pointer to the string manufacturer descriptor 00192 */ 00193 virtual uint8_t * stringImanufacturerDesc(); 00194 00195 /* 00196 * Get string product descriptor 00197 * 00198 * @returns pointer to the string product descriptor 00199 */ 00200 virtual uint8_t * stringIproductDesc(); 00201 00202 /* 00203 * Get string serial descriptor 00204 * 00205 * @returns pointer to the string serial descriptor 00206 */ 00207 virtual uint8_t * stringIserialDesc(); 00208 00209 /* 00210 * Get string configuration descriptor 00211 * 00212 * @returns pointer to the string configuration descriptor 00213 */ 00214 virtual uint8_t * stringIConfigurationDesc(); 00215 00216 /* 00217 * Get string interface descriptor 00218 * 00219 * @returns pointer to the string interface descriptor 00220 */ 00221 virtual uint8_t * stringIinterfaceDesc(); 00222 00223 /* 00224 * Get the length of the report descriptor 00225 * 00226 * @returns length of the report descriptor 00227 */ 00228 virtual uint16_t reportDescLength() { return 0; }; 00229 00230 00231 00232 protected: 00233 virtual void busReset(void); 00234 virtual void EP0setupCallback(void); 00235 virtual void EP0out(void); 00236 virtual void EP0in(void); 00237 virtual void connectStateChanged(unsigned int connected); 00238 virtual void suspendStateChanged(unsigned int suspended); 00239 uint8_t * findDescriptor(uint8_t descriptorType); 00240 CONTROL_TRANSFER * getTransferPtr(void); 00241 00242 uint16_t VENDOR_ID; 00243 uint16_t PRODUCT_ID; 00244 uint16_t PRODUCT_RELEASE; 00245 00246 private: 00247 bool addRateFeedbackEndpoint(uint8_t endpoint, uint32_t maxPacket); 00248 bool requestGetDescriptor(void); 00249 bool controlOut(void); 00250 bool controlIn(void); 00251 bool requestSetAddress(void); 00252 bool requestSetConfiguration(void); 00253 bool requestSetFeature(void); 00254 bool requestClearFeature(void); 00255 bool requestGetStatus(void); 00256 bool requestSetup(void); 00257 bool controlSetup(void); 00258 void decodeSetupPacket(uint8_t *data, SETUP_PACKET *packet); 00259 bool requestGetConfiguration(void); 00260 bool requestGetInterface(void); 00261 bool requestSetInterface(void); 00262 00263 CONTROL_TRANSFER transfer; 00264 USB_DEVICE device; 00265 00266 uint16_t currentInterface; 00267 uint8_t currentAlternate; 00268 }; 00269 00270 00271 #endif
Generated on Tue Jul 12 2022 16:20:43 by
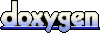