
A simple program to use a Seeed ultrasound range finder.
Dependencies: RangeFinder mbed Pulse
main.cpp
00001 /* Copyright (c) 2012 Nick Ryder, University of Oxford 00002 * nick.ryder@physics.ox.ac.uk 00003 * 00004 * MIT License 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00007 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00008 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00009 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in all copies or 00013 * substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00016 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00017 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00018 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00020 */ 00021 00022 #include "mbed.h" 00023 00024 #include "RangeFinder.h" 00025 00026 // Seeed ultrasound range finder 00027 RangeFinder rf(p21, 10, 5800.0, 100000); 00028 DigitalOut led(LED1); 00029 00030 int main() { 00031 led = 1; 00032 float d; 00033 while (1) { 00034 d = rf.read_m(); 00035 if (d == -1.0) { 00036 printf("Timeout Error.\n"); 00037 } else if (d > 5.0) { 00038 // Seeed's sensor has a maximum range of 4m, it returns 00039 // something like 7m if the ultrasound pulse isn't reflected. 00040 printf("No object within detection range.\n"); 00041 } else { 00042 printf("Distance = %f m.\n", d); 00043 } 00044 wait(0.5); 00045 led = !led; 00046 } 00047 }
Generated on Tue Jul 12 2022 11:14:40 by
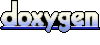