AD9249 ADC
Fork of adc_ad9249 by
Embed:
(wiki syntax)
Show/hide line numbers
SWSPI_BI.cpp
00001 /* SWSPI, Software SPI library 00002 * Copyright (c) 2012-2014, David R. Van Wagner, http://techwithdave.blogspot.com 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 /* 00023 * modified the software for adapt to the AD9249 ADC ( bi directional ) with LVDS interface 00024 00025 00026 */ 00027 00028 #include <mbed.h> 00029 #include "SWSPI_BI.h" 00030 #include "S_SCTRL_SM1_hwfunct.h" 00031 00032 #define SWSPI_BI_SRC_VER "1.50" 00033 00034 /** 00035 PARAM 00036 @misopin pointer to the input / output pin for the data communication 00037 @rdir_pin pointer to the output pin that sets the remote LVDS buffer to read / write 00038 @ldir_pin pointer to the output pin that sets the local LVDS buffer to read / write 00039 @sclk_pin pointer to the output pin that act as the clock signal for the interface 00040 00041 */ 00042 SWSPI_BI::SWSPI_BI(DigitalInOut *msio_pin, DigitalOut *rdir_pin,DigitalOut *ldir_pin, DigitalOut *sclk_pin) 00043 :getVersion( SWSPI_BI_HDR_VER , SWSPI_BI_SRC_VER , __TIME__, __DATE__) 00044 { 00045 00046 msio = msio_pin; 00047 rdir = rdir_pin; 00048 ldir = ldir_pin; 00049 sclk = sclk_pin; 00050 set_bi_spi_mo(1, msio,ldir,rdir); 00051 00052 format(8); 00053 frequency(500000); 00054 } 00055 00056 SWSPI_BI::~SWSPI_BI() 00057 { 00058 } 00059 00060 void SWSPI_BI::format( int bitsin, int modein ){ 00061 bits = bitsin; 00062 mode = modein; 00063 polarity = !((modein >> 1) & 1); 00064 phase = modein & 1; 00065 sclk->write(polarity); 00066 } 00067 00068 void SWSPI_BI::frequency(int hz) 00069 { 00070 this->freq = hz; 00071 } 00072 00073 void SWSPI_BI::write(unsigned int value, DigitalOut * cs, bool lastdata, int cs_pol,bool nxtrd ){ 00074 00075 // write data to output 00076 // assumption is that the cs line was just set from high to low so that the SPI slave is in read mode 00077 value= ~value; 00078 set_bi_spi_mo(1,msio,ldir,rdir); 00079 cs->write(cs_pol); 00080 for (int bit = bits-1; bit >= 0; --bit) { 00081 msio->write(((value >> bit) & 0x01) != 0); 00082 if(phase) { sclk->write(!polarity); wait(1.0/freq/2); sclk->write(polarity); 00083 if(!bit and nxtrd) set_bi_spi_mo(0,msio,ldir,rdir); // set already to input mode 00084 wait(1.0/freq/2); } 00085 else { wait(1.0/freq/2); sclk->write(!polarity); wait(1.0/freq/2); sclk->write(polarity); } 00086 00087 00088 } 00089 if( lastdata) { 00090 set_bi_spi_mo(0,msio,ldir,rdir); 00091 cs->write(!cs_pol); 00092 } 00093 else { 00094 } 00095 } 00096 00097 unsigned int SWSPI_BI::read( DigitalOut * cs, bool lastdata, int cs_pol ){ 00098 unsigned int read = 0; 00099 set_bi_spi_mo(0,msio,ldir,rdir); 00100 cs->write(cs_pol); 00101 wait(1.0/freq/2); 00102 for (int bit = bits-1; bit >= 0; --bit) { 00103 if (phase == 0) { 00104 if (msio->read()) read |= (1 << bit); 00105 00106 } 00107 sclk->write(!polarity); 00108 wait(1.0/freq/2); 00109 if (phase == 1) { 00110 if (msio->read()) read |= (1 << bit); 00111 } 00112 00113 sclk->write(polarity); 00114 wait(1.0/freq/2); 00115 } 00116 if( lastdata) { 00117 cs->write(!cs_pol); 00118 } 00119 // else keep current io config 00120 00121 00122 return ~read; 00123 } 00124
Generated on Fri Jul 22 2022 16:13:35 by
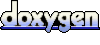