
SiPM basded cosmic ray detector data acquisition system.
Dependencies: DNSResolver EthernetNetIf FatFileSystem SDFileSystem mbed
SiPM.cpp
00001 #include "SiPM.h" 00002 00003 SiPM::SiPM(PinName pin, PinName led) : 00004 n(0), ncoincidence(0), ntrig(0), 00005 time(), 00006 checkcoincidence(false), running(false), 00007 interval(20), deadtime(50), 00008 other(NULL), 00009 iin(pin), ledpin(led) 00010 { 00011 iin.rise(this, &SiPM::trigger); 00012 ledpin = 1; 00013 } 00014 00015 void SiPM::addother(SiPM * sipm) { 00016 other = sipm; 00017 checkcoincidence = true; 00018 } 00019 00020 void SiPM::setinterval_us(int t) { 00021 interval = t; 00022 } 00023 00024 void SiPM::setdeadtime_us(int t) { 00025 deadtime = t; 00026 } 00027 00028 void SiPM::trigger() { 00029 ntrig++; 00030 if (running) { 00031 ledpin = !ledpin; 00032 if (time.read_us() > deadtime) { 00033 n++; 00034 time.reset(); 00035 if (checkcoincidence) { 00036 if (other->time.read_us() < interval) { 00037 ncoincidence++; 00038 } 00039 } 00040 } 00041 } 00042 } 00043 00044 void SiPM::reset() { 00045 n = 0; 00046 ncoincidence = 0; 00047 ntrig = 0; 00048 start(); 00049 } 00050 00051 void SiPM::start() { 00052 running = true; 00053 time.start(); 00054 printf("# Starting SiPM.\n"); 00055 } 00056 00057 void SiPM::stop() { 00058 running = false; 00059 printf("#Stopping SiPM.\n"); 00060 }
Generated on Tue Jul 19 2022 01:43:40 by
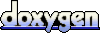