Biblioteca para uso do ultrassom HC-SR04
Dependents: Sonar-HC-SR04 CarrinhoLabirinto Nucleo_Us_ticker_20160803 ProjetoSO ... more
HCSR04.h
00001 /** 00002 * @author Nestor Pereira-Neto Team: SalvadorEngenharia 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * Biblioteca para uso do sonar HC-SR04, possui rotinas para permitir medições 00029 * de distências retornado valores em centímetros, polegadas ou o tempo 00030 * percorrido pelo som (em microsegundos). 00031 * Esta biblioteca pode ser usada com qualquer pino do microcontrolador. 00032 */ 00033 00034 00035 #ifndef HCSR04_H 00036 #define HCSR04_H 00037 00038 #include "mbed.h" 00039 00040 00041 00042 /** 00043 * Sonar HC-SR04 example. 00044 * @code 00045 * #include "mbed.h" 00046 * #include "HCSR04.h" 00047 * 00048 * DigitalOut myled(LED1); 00049 * Serial pc(USBTX,USBRX); 00050 * 00051 * HCSR04 sonar(PTD5, PTA13); 00052 * 00053 * int main() { 00054 * while(1) { 00055 * printf("Distancia detectada pelo sensor Frente %.2f cm \n", sonar.getCm()); 00056 * wait_ms(1000); 00057 * } 00058 * } 00059 * 00060 * @endcode 00061 */ 00062 00063 class HCSR04 { 00064 public: 00065 /** Constructor, create HCSR04 instance 00066 * 00067 * @param trigger TRIG pin 00068 * @param echo ECHO pin 00069 */ 00070 HCSR04(PinName trigger, PinName echo); 00071 00072 /** It make a reading of the sonar Faz uma leitura do sonar 00073 * 00074 * @returns Tempo do pulso echo em microsegundos*/ 00075 float readEcho(void); 00076 00077 /** It messures the distance in centimeter "cm" 00078 * 00079 *@returns Distance in centimeter 00080 */ 00081 float getCm(void); 00082 00083 /** Mede a distência em polegadas "in" 00084 * 00085 *@returns Distência em in*/ 00086 float getIn(void); 00087 00088 private: 00089 float tdist; //Leitura do tempo transcorrido 00090 float distcm; //Guarda o valor da distanciância em centímetros 00091 float distin; //Guarda o valor da distência em polegadas 00092 00093 DigitalOut _t; //Configuração do pino de Trigger 00094 DigitalIn _e; //Configuração do pino de Echo 00095 Timer _tempo; //Cria um objeto timer 00096 00097 }; 00098 00099 #endif
Generated on Tue Jul 12 2022 21:12:29 by
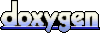