Humidity and Temperature Sensor - Sensirion SHT1x driver
Dependents: ProgrammePrincipal Wetterstation project1 4180_Project ... more
sht15.cpp
00001 /** 00002 * Copyright (c) 2010 Roy van Dam <roy@negative-black.org> 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions 00007 * are met: 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 00014 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS'' AND 00015 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00016 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00017 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS BE LIABLE 00018 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00019 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS 00020 * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00021 * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00022 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY 00023 * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00024 * SUCH DAMAGE. 00025 */ 00026 00027 #include "sht15.hpp" 00028 00029 namespace SHTx { 00030 SHT15::SHT15(PinName sda, PinName scl): i2c(sda, scl) { 00031 this->ready = true; 00032 wait_ms(11); 00033 } 00034 00035 float 00036 SHT15::getTemperature(void) { 00037 return this->convertTemperature( 00038 this->temperature, 00039 this->getFlag(flag_resolution), 00040 this->scale 00041 ); 00042 } 00043 00044 float 00045 SHT15::getHumidity(void) { 00046 return this->convertHumidity( 00047 this->humidity, 00048 this->temperature, 00049 this->getFlag(flag_resolution) 00050 ); 00051 } 00052 00053 bool 00054 SHT15::checkBattery(void) { 00055 this->readRegister(cmd_read_register); 00056 return this->getFlag(flag_battery); 00057 } 00058 00059 bool 00060 SHT15::setHeater(bool value) { 00061 this->setFlag(flag_heater, value); 00062 return this->writeRegister(); 00063 } 00064 00065 bool 00066 SHT15::setResolution(bool value) { 00067 this->setFlag(flag_resolution, value); 00068 return this->writeRegister(); 00069 } 00070 00071 bool 00072 SHT15::setOTPReload(bool value) { 00073 this->setFlag(flag_otp_reload, !value); 00074 return this->writeRegister(); 00075 } 00076 00077 void 00078 SHT15::setScale(bool value) { 00079 this->scale = value; 00080 } 00081 00082 bool 00083 SHT15::update(void) { 00084 if ((this->ready == false) || 00085 !this->readRegister(cmd_read_temperature) || 00086 !this->readRegister(cmd_read_humidity)) { 00087 return false; 00088 } 00089 00090 return true; 00091 } 00092 00093 bool 00094 SHT15::reset(void) { 00095 while (this->ready == false) { 00096 continue; 00097 } 00098 00099 this->ready = false; 00100 this->i2c.start(); 00101 bool ack = this->i2c.write(cmd_reset_device); 00102 this->i2c.stop(); 00103 this->ready = true; 00104 00105 if (ack) { 00106 this->status_register = 0; 00107 this->humidity = 0; 00108 this->temperature = 0; 00109 wait_ms(11); 00110 return true; 00111 } 00112 00113 return false; 00114 } 00115 00116 void 00117 SHT15::connectionReset(void) { 00118 this->i2c.reset(); 00119 } 00120 00121 float 00122 SHT15::convertTemperature(uint16_t sot, bool res, bool scale) { 00123 // Temperature conversion coefficients 00124 float d1 = this->coefficient.dv[scale]; 00125 float d2 = ((scale) ? this->coefficient.df[res] 00126 : this->coefficient.dc[res]); 00127 00128 // Temperature data conversion 00129 return d1 + (d2 * (float)(sot)); 00130 } 00131 00132 float 00133 SHT15::convertHumidity(uint16_t sohr, uint16_t sot, bool res) { 00134 // Humidity conversion coefficients 00135 float c1 = this->coefficient.c1[res]; 00136 float c2 = this->coefficient.c2[res]; 00137 float c3 = this->coefficient.c3[res]; 00138 00139 // Temperature compensation coefficients 00140 float t1 = this->coefficient.t1[res]; 00141 float t2 = this->coefficient.t2[res]; 00142 00143 // Temperature data conversion to celcius 00144 float temp = this->convertTemperature(sot, res, false); 00145 00146 // Humidity data conversion to relative humidity 00147 float humid = c1 + (c2 * (float)(sohr)) + (c3 * (float)(sohr * sohr)); 00148 00149 // Calculate temperature compensation 00150 return (temp - 25) + (t1 + (t2 * (float)(sohr)) + humid); 00151 } 00152 00153 bool 00154 SHT15::writeRegister(void) { 00155 while (this->ready == false) { 00156 continue; 00157 } 00158 00159 this->ready = false; 00160 this->i2c.start(); 00161 00162 if (this->i2c.write(cmd_write_register)) { 00163 this->i2c.write(this->status_register); 00164 } 00165 00166 this->i2c.stop(); 00167 this->ready = true; 00168 00169 return true; 00170 } 00171 00172 bool 00173 SHT15::readRegister(cmd_list command) { 00174 while (this->ready == false) { 00175 continue; 00176 } 00177 00178 this->ready = false; 00179 this->i2c.start(); 00180 00181 if (!this->i2c.write(command)) { 00182 this->i2c.stop(); 00183 return false; 00184 } 00185 00186 switch (command) { 00187 case cmd_read_temperature: { 00188 if (!this->i2c.wait()) { 00189 this->i2c.stop(); 00190 return false; 00191 } 00192 00193 this->temperature = this->i2c.read(1) << 8; 00194 this->temperature |= this->i2c.read(0); 00195 } break; 00196 00197 case cmd_read_humidity: { 00198 if (!this->i2c.wait()) { 00199 this->i2c.stop(); 00200 return false; 00201 } 00202 00203 this->humidity = this->i2c.read(1) << 8; 00204 this->humidity |= this->i2c.read(0); 00205 } break; 00206 00207 case cmd_read_register: { 00208 this->status_register = this->i2c.read(0); 00209 } break; 00210 } 00211 00212 this->i2c.stop(); 00213 this->ready = true; 00214 00215 return true; 00216 } 00217 00218 bool 00219 SHT15::getFlag(SHT15::flag_list flag) { 00220 return (this->status_register & flag) ? true : false; 00221 } 00222 00223 void 00224 SHT15::setFlag(SHT15::flag_list flag, bool value) { 00225 if (value) { 00226 this->status_register |= flag; 00227 } else { 00228 this->status_register &= ~flag; 00229 } 00230 } 00231 }
Generated on Wed Jul 13 2022 17:19:11 by
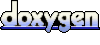