
Program to transmit strings as Morse code using the CC1200. Useful for amateur radio projects!
Dependencies: CC1200 SerialStream
main.cpp
00001 // 00002 // Test program for the CC1200 morse code example 00003 // 00004 00005 #include <mbed.h> 00006 #include <SerialStream.h> 00007 00008 #include <CC1200.h> 00009 #include <cinttypes> 00010 00011 #include "CC1200Morse.h" 00012 00013 #define PIN_SPI_MOSI P0_9 00014 #define PIN_SPI_MISO P0_8 00015 #define PIN_SPI_SCLK P0_7 00016 00017 #define PIN_CC1200_CS P0_6 00018 #define PIN_CC1200_RST P0_29 00019 00020 BufferedSerial serial(USBTX, USBRX, 115200); 00021 SerialStream<BufferedSerial> pc(serial); 00022 00023 CC1200 radio(PIN_SPI_MOSI, PIN_SPI_MISO, PIN_SPI_SCLK, PIN_CC1200_CS, PIN_CC1200_RST, &pc); 00024 00025 void testMorseCodeConversion() 00026 { 00027 radio.begin(); 00028 CC1200Morse morseConverter(radio); 00029 00030 const size_t bufferLen = 64; 00031 uint8_t outputBuffer[bufferLen]; 00032 00033 char const * testString = "eternal silence@54!"; 00034 00035 CC1200Morse::EncodedMorse morse = morseConverter.convertToMorse(testString, outputBuffer, bufferLen); 00036 00037 if(!morse.valid) 00038 { 00039 pc.printf("Morse is invalid\n"); 00040 } 00041 else 00042 { 00043 pc.printf("Output %zu bytes:", morse.totalLength); 00044 for(size_t index = 0; index < morse.totalLength; ++index) 00045 { 00046 pc.printf(" %" PRIx8, outputBuffer[index]); 00047 } 00048 pc.printf("\n"); 00049 } 00050 } 00051 00052 /** 00053 * Test sending some arbitrary bytes as OOK modulated data. 00054 */ 00055 void testMorseByteTransmission() 00056 { 00057 const float timeUnit = 0.1f; 00058 00059 radio.begin(); 00060 CC1200Morse morseConverter(radio); 00061 morseConverter.configure(CC1200::Band::BAND_820_960MHz, 915e6f, timeUnit, 14.5); 00062 00063 const char testString[] = "\xFF\x0E\xFF"; 00064 const size_t testStringLength = 3; 00065 00066 // manually create morse code data 00067 CC1200Morse::EncodedMorse morse; 00068 morse.valid = true; 00069 morse.buffer = reinterpret_cast<uint8_t const *>(testString); 00070 morse.byteLen = testStringLength; 00071 morse.bitLen = 0; 00072 morse.totalLength = testStringLength; 00073 00074 morseConverter.transmit(morse); 00075 00076 Timer messageTimer; 00077 messageTimer.start(); 00078 00079 radio.setOnTransmitState(CC1200::State::IDLE); 00080 radio.startTX(); 00081 00082 // wait until all bytes have been transmitted. 00083 // Note: the FIFO length is 0 when the last byte is being sent, so we can't just check the FIFO length 00084 while(radio.getTXFIFOLen() > 0 || radio.getState() != CC1200::State::IDLE) 00085 { 00086 pc.printf("TX FIFO size: %zu\n", radio.getTXFIFOLen()); 00087 ThisThread::sleep_for(std::chrono::milliseconds(static_cast<uint32_t>(timeUnit * 1000))); 00088 //ThisThread::sleep_for(1ms); 00089 } 00090 00091 float timeSeconds = std::chrono::duration_cast<std::chrono::duration<float>>(messageTimer.elapsed_time()).count(); 00092 size_t numBits = ((morse.byteLen * 8) + morse.bitLen); 00093 float effectiveBitrate = numBits / timeSeconds; 00094 00095 pc.printf("Sent %zu bits in %.03f s, effective bitrate = %.03f sps\n", numBits, timeSeconds, effectiveBitrate); 00096 00097 } 00098 00099 void testMorseCodeTransmission() 00100 { 00101 const float timeUnit = 0.1f; 00102 00103 radio.begin(); 00104 CC1200Morse morseConverter(radio); 00105 morseConverter.configure(CC1200::Band::BAND_820_960MHz, 915e6f, timeUnit, 0); 00106 00107 const size_t bufferLen = 64; 00108 uint8_t outputBuffer[bufferLen]; 00109 00110 char const * testString = "eternal silence@54!"; 00111 00112 CC1200Morse::EncodedMorse morse = morseConverter.convertToMorse(testString, outputBuffer, bufferLen); 00113 00114 if(!morse.valid) 00115 { 00116 pc.printf("Morse is invalid\n"); 00117 } 00118 00119 morseConverter.transmit(morse); 00120 00121 radio.setOnTransmitState(CC1200::State::IDLE); 00122 radio.startTX(); 00123 00124 // wait until all bytes have been transmitted. 00125 // Note: the FIFO length is 0 when the last byte is being sent, so we can't just check the FIFO length 00126 while(radio.getTXFIFOLen() > 0 || radio.getState() != CC1200::State::IDLE) 00127 { 00128 pc.printf("TX FIFO size: %zu\n", radio.getTXFIFOLen()); 00129 ThisThread::sleep_for(std::chrono::milliseconds(static_cast<uint32_t>(timeUnit * 1000))); 00130 //ThisThread::sleep_for(1ms); 00131 } 00132 } 00133 00134 int main() 00135 { 00136 pc.printf("\nCC1200 Morse Test Suite:\n"); 00137 00138 while(1){ 00139 int test=-1; 00140 //MENU. ADD AN OPTION FOR EACH TEST. 00141 pc.printf("Select a test: \n"); 00142 pc.printf("1. Test converting to Morse code\n"); 00143 pc.printf("2. Test transmitting bytes as Morse\n"); 00144 pc.printf("3. Test transmitting string of Morse\n"); 00145 00146 pc.scanf("%d", &test); 00147 printf("Running test %d:\n\n", test); 00148 //SWITCH. ADD A CASE FOR EACH TEST. 00149 switch(test) { 00150 case 1: testMorseCodeConversion(); break; 00151 case 2: testMorseByteTransmission(); break; 00152 case 3: testMorseCodeTransmission(); break; 00153 default: pc.printf("Invalid test number. Please run again.\n"); continue; 00154 } 00155 pc.printf("done.\r\n"); 00156 } 00157 00158 return 0; 00159 00160 }
Generated on Sun Jul 24 2022 06:31:11 by
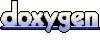