AccurateWaiter allows threaded applications to have threads wait for a precise amount of time, without sending the CPU into a permanent spinlock.
Dependents: AccurateWaiter-Test
AccurateWaiter.cpp
00001 // 00002 // Created by jamie on 11/12/2020. 00003 // 00004 00005 #include "AccurateWaiter.h" 00006 00007 AccurateWaiter::AccurateWaiter(): 00008 TimerEvent(get_us_ticker_data()) 00009 { 00010 00011 } 00012 00013 00014 void AccurateWaiter::handler() 00015 { 00016 // This signals the RTOS that the waiting thread is ready to wake up. 00017 flags.set(1); 00018 } 00019 00020 void AccurateWaiter::wait_for(std::chrono::microseconds duration) 00021 { 00022 // set up timer event to occur 00023 insert(duration); 00024 00025 // wait for event flag and then clear it 00026 flags.wait_all(1); 00027 } 00028 00029 void AccurateWaiter::wait_until(TickerDataClock::time_point timePoint) 00030 { 00031 // set up timer event to occur 00032 insert_absolute(timePoint); 00033 00034 // wait for event flag and then clear it 00035 flags.wait_all(1); 00036 }
Generated on Tue Jul 26 2022 01:26:16 by
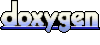