
Scalextric Lap Timer using ultrasonic sensor
Dependencies: HCSR04 mbed TextLCD
main.cpp
00001 // Scalextric Timer for Ada Lovelace Day 00002 // 00003 // Uses Nucleo board F072 with LCD Keypad shield 00004 // Connect D3 to Trig & D2 to Echo 00005 // Connect VCC to 5V (pin CN7 18) 00006 // Connect GND to 0V (pin CN7 19) 00007 00008 #include "mbed.h" 00009 #include "hcsr04.h" // Ultrasonic sensor 00010 #include "TextLCD.h" // LCD1602 00011 00012 // serial PC for debug 00013 //Serial pc(SERIAL_TX, SERIAL_RX); 00014 // Ultrasonic sensor 00015 HCSR04 usensor(D3,D2); // Trig, Echo - locks sometimes 00016 00017 // LCD Shield 00018 TextLCD lcd(D8, D9, D4, D5, D6, D7); // used to drive display 00019 //AnalogIn keys(A0); // used to sample buttons 00020 00021 Timer lap_timer; 00022 00023 // Subroutine to display text on LCD - called from below 00024 // call as follows: 00025 // textLCD(text, line) 00026 // where text = up to 16 characters of text 00027 // line = line 0 or 1 on the LCD; where 0 is the top line and 1 is the bottom line 00028 00029 void textLCD(char *text, int line) { 00030 00031 char tmpBuf[16]; 00032 for (int i = 0; i < 14; i++) { 00033 if (i < strlen(text)) { 00034 tmpBuf[i] = text[i]; 00035 } 00036 else 00037 { 00038 tmpBuf[i] = 0x20; 00039 } 00040 lcd.locate(i, line); 00041 lcd.putc(tmpBuf[i]); 00042 } 00043 } 00044 00045 // Subroutine to display Lap data on LCD - called from below 00046 // call as follows: 00047 // DisplayLap(lap_count, dist, lap_time, Show_Time); 00048 00049 void DisplayLap(int lcl_lap_count, int lcl_dist, float lcl_lap_time, bool Show_Time) { 00050 00051 char lap_string[16]; 00052 char lap_string2[16]; 00053 //textLCD("1 ", 0); // Clear top line on LCD 00054 //textLCD("2 ", 1); // Clear bottom line on LCD 00055 //lcd.cls(); 00056 00057 int lcl_lap_time_integerPart = (int)lcl_lap_time; 00058 int lcl_lap_time_decimalPart = ((int)(lcl_lap_time*1000)%1000); 00059 00060 sprintf(lap_string, "Lap : %d D : %d", (char*)lcl_lap_count, (char*)lcl_dist); // Format Lap count string 00061 // pc.printf("%s \n\r", lap_string); 00062 textLCD("1 ", 0); // Clear top line on LCD 00063 textLCD(lap_string, 0); // Display distance on LCD 00064 00065 if (Show_Time) { 00066 sprintf(lap_string2, "Time : %d.%d s, ", (char*)lcl_lap_time_integerPart, (char*)lcl_lap_time_decimalPart); // Format Lap time string 00067 // pc.printf("%s \n\r", lap_string); 00068 textLCD("1 ", 1); // Clear bottom line on LCD 00069 textLCD(lap_string2, 1); // Display Lap Time on LCD 00070 } 00071 } 00072 00073 /////////////////////////////////////////////////////////////////////////////// 00074 // Main Program starts here 00075 /////////////////////////////////////////////////////////////////////////////// 00076 00077 int main() 00078 { 00079 00080 //// Try changing these: 00081 // Variables to tune 00082 float min_laptime = 1.0; // Minimum laptime - time to wait after seeing car before measuring again in seconds 00083 int sample_time = 500; // Sample time - time between sampling values from sensor in milliseconds 00084 int trigger_distance = 5; // Trigger distance - distance below which the car registers as detected 00085 //// Careful with anything after this though 00086 00087 // Variables used within the program - set to their initial values 00088 int dist = 0; 00089 float lap_time = 0.0; 00090 int lap_count = 0; 00091 00092 00093 // Initialise the serial interface and set its data rate 00094 // pc.baud(9600); 00095 00096 // Intro Screen 00097 // pc.printf("ARM Sheffield Scalextric Challenge \n"); // Display to Serial Interface 00098 textLCD("ARM Scalextric ", 0); // Display on First Line of LCD 00099 wait_ms(2000); // Wait 2s 00100 textLCD(" ", 0); // Clear top line on LCD 00101 00102 // Main loop - runs forever 00103 while(1) { 00104 // Set up and read ultrasonic sensor after "sample_time" 00105 usensor.start(); 00106 wait_ms(sample_time); 00107 dist=usensor.get_dist_cm(); // read sesnsor distance measure 00108 //pc.printf("Lap %d Distance %d wait_time %d \n\r", lap_count, dist, wait_time); // Debug - display distance to Serial Interface 00109 if (dist < trigger_distance) { // Spotted something closer than trigger_distance from the sensor 00110 if (lap_count == 0) { // First Lap - start lap timer 00111 lap_timer.reset(); 00112 lap_timer.start(); 00113 //pc.printf("Timing... \r"); // Debug to Serial Interface 00114 textLCD("Timing..........", 1); // Message to LCD 00115 } else { // Completed a lap 00116 lap_time = lap_timer.read(); // Get time for lap 00117 lap_timer.reset(); 00118 DisplayLap(lap_count, dist, lap_time, 1); 00119 } 00120 lap_count++; // Increment lap counter by 1 00121 wait(min_laptime); // Wait for a time before starting to monitor sensor for next lap 00122 } // end of something spotted 00123 else // nothing spotted - output debug 00124 { 00125 DisplayLap(lap_count, dist, lap_time, 0); 00126 } 00127 } // end of main while loop 00128 00129 00130 } // end of main program 00131
Generated on Sun Jul 24 2022 14:58:03 by
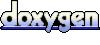