
player 1
Dependencies: 4DGL-uLCD-SE PinDetect SparkfunAnalogJoystick mbed-rtos mbed SDFileSystem
Fork of 4180FinalLab by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "PinDetect.h" 00004 #include "Speaker.h" 00005 #include "soundBuilder.h" 00006 #include "SparkfunAnalogJoystick.h" 00007 #include "paddle.h" 00008 #include "ball.h" 00009 #include "huzzah.h" 00010 #include "SDFileSystem.h" 00011 00012 // Pushbuttons 00013 SparkfunAnalogJoystick joystick(p16, p15, p14); 00014 //PinDetect select(p13); 00015 //Speaker 00016 Speaker mySpeaker(p25); 00017 Serial pc(USBTX, USBRX); 00018 Serial xbee(p9, p10); 00019 00020 //Wifi 00021 Serial esp(p28,p27); 00022 Huzzah huzzah("fese","12345678", &esp); 00023 DigitalOut reset(p26); 00024 int player1score,player2score; 00025 00026 //SD Card Storage 00027 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00028 00029 // State machine definitions 00030 enum gameStateType {START, WAIT, GAME_SETUP, GAME, WIN, LOSE}; 00031 /* State Definitions: 00032 * START -- Creates the start screen 00033 * WAIT -- After the start screen, goes into wait where mbed spins and does nothing 00034 * GAME_SETUP -- Sets up one time things (like boarders, initializes beginning velocity 00035 * GAME -- When the user actually gets to play 00036 * LOSE -- clears the screen, prints you lose, waits, then goes back to start 00037 */ 00038 00039 // Global state machine variable (So that the select can modify it) 00040 gameStateType gameState = START; 00041 bool ready = false; 00042 00043 Paddle botPaddle(0, 0, 0, 0); 00044 Paddle topPaddle(0, 0, 0, 0); 00045 Ball ball(0, 0, 0); 00046 00047 uint8_t gameLowX = 10, gameHighX = 190, gameLowY = 5, gameHighY = 245, gameX = 200, gameY = 250; 00048 uint8_t gameCenterX = gameX/2, gameCenterY = gameY/2; 00049 uint8_t ballSize=10; 00050 uint8_t paddleWidth = 5, paddleLength = 40; 00051 float botMove = joystick.yAxis(); 00052 uint8_t botScore = 0; 00053 uint8_t topScore = 0; 00054 int i = 0; 00055 00056 // thread that plays game sounds through the speaker 00057 void speaker_thread(void const *argument) { 00058 00059 Speaker *player = &mySpeaker; 00060 00061 // Start Song 00062 float sFreq[] = {550,750,550,750}; 00063 float sDur[] = {.3,.3,.3,.3}; 00064 float sVol[] = {.01,.01,.01,.01}; 00065 SoundBuilder startSong(sFreq, sDur, sVol, sizeof(sFreq)/sizeof(*sFreq), player); 00066 00067 // End Song 00068 float eFreq[] = {300,200,250,225,200,150,150,100}; 00069 float eDur[] = {.3,.3,.3,.3,.3,.3,.3,.3}; 00070 float eVol[] = {.01,.01,.01,.01,.01,.01,.01,.01}; 00071 SoundBuilder endSong(eFreq, eDur, eVol, sizeof(eFreq)/sizeof(*eFreq), player); 00072 00073 while (true) { 00074 switch (gameState) { 00075 case GAME: // if game is running and user dodges a pipe, play a note 00076 if (topPaddle.checkHit(ball.getFutureX(), ball.getFutureY(), ball.getSize()) 00077 || botPaddle.checkHit(ball.getFutureX(), ball.getFutureY(), ball.getSize()) 00078 || ball.getFutureX() <= 10 00079 || ball.getFutureX() + ball.getSize() >= 190) { 00080 mySpeaker.PlayNote(440, 0.1, 0.01); 00081 } 00082 00083 if (ball.getY() < 5){ 00084 mySpeaker.PlayNote(440, 0.1, 0.01); 00085 mySpeaker.PlayNote(880, 0.1, 0.01); 00086 } 00087 else if (ball.getY() + ball.getSize() > 245){ 00088 mySpeaker.PlayNote(880, 0.1, 0.01); 00089 mySpeaker.PlayNote(440, 0.1, 0.01); 00090 } 00091 00092 break; 00093 case START: // play a song at the start of the game 00094 startSong.playSong(); 00095 Thread::wait(5000); 00096 break; 00097 case WIN: // play a song when the player wins the game 00098 case LOSE: // play a song when the player loses the game 00099 endSong.playSong(); 00100 Thread::wait(5000); 00101 break; 00102 } 00103 } 00104 } 00105 00106 // thread that writes to the sd card and loads highscores to website 00107 void sd_card_thread(void const *argument) { 00108 00109 FILE *fp = NULL; 00110 fp = fopen("/sd/highscore.txt", "r"); 00111 fscanf(fp, "%i\n%i", &player1score, &player2score); 00112 fclose(fp); 00113 00114 huzzah.config(); 00115 huzzah.sendwebpage(player1score, player2score); 00116 00117 while (true) { 00118 00119 switch (gameState) { 00120 case WIN: 00121 // overwrite previous high score 00122 player1score++; 00123 fp = fopen("/sd/highscore.txt", "w"); 00124 fprintf(fp, "%i\n%i", player1score, player2score); 00125 fclose(fp); 00126 huzzah.config(); 00127 huzzah.sendwebpage(player1score, player2score); 00128 break; 00129 case LOSE: 00130 // overwrite previous high score 00131 player2score++; 00132 fp = fopen("/sd/highscore.txt", "w"); 00133 fprintf(fp, "%i\n%i", player1score, player2score); 00134 fclose(fp); 00135 huzzah.config(); 00136 huzzah.sendwebpage(player1score, player2score); 00137 break; 00138 } 00139 } 00140 } 00141 00142 void xbee_thread(void const *argument) { 00143 00144 while (true) { 00145 switch (gameState) { 00146 case WAIT: 00147 if (ready && xbee.writeable()) { 00148 xbee.putc(1); 00149 } 00150 if (ready && xbee.readable()) { 00151 char c = xbee.getc(); 00152 if (c == 1) { 00153 gameState = GAME_SETUP; 00154 } 00155 } 00156 break; 00157 case GAME: 00158 if (xbee.writeable()) { 00159 xbee.putc(botPaddle.getX()); 00160 } 00161 if (xbee.readable()) { 00162 char c = xbee.getc(); 00163 uint8_t top = 200 - paddleLength - ((uint8_t) c); 00164 topPaddle.setX(top); 00165 } 00166 break; 00167 } 00168 } 00169 } 00170 00171 int main() 00172 { 00173 //WifiServer Configuration and set-up 00174 reset=0; //hardware reset for 8266 00175 //Thread::wait(500); 00176 reset=1; 00177 esp.baud(9600); 00178 //huzzah.config(); 00179 //huzzah.sendwebpage(highscore1,highscore2); 00180 00181 // This is setting up the joystick select as a pushbutton 00182 //joystick.set_callback(&select_hit_callback); 00183 00184 pc.format(8, SerialBase::None, 1); 00185 pc.baud(115200); 00186 00187 botPaddle = Paddle(gameCenterX-(paddleLength/2), gameHighY, paddleLength, paddleWidth); 00188 botPaddle.setLimits(gameLowX, gameHighX); 00189 botPaddle.setMaxMove(3); 00190 topPaddle = Paddle(gameCenterX-(paddleLength/2), gameLowY, paddleLength, paddleWidth); 00191 topPaddle.setLimits(gameLowX, gameHighX); 00192 topPaddle.setMaxMove(3); 00193 ball = Ball(gameCenterX, gameCenterY, ballSize); 00194 00195 ball.setVxDir(true); 00196 ball.setVyDir(true); 00197 00198 while (!pc.readable()){ 00199 00200 } 00201 00202 Thread thread1(speaker_thread); 00203 Thread thread2(sd_card_thread); 00204 Thread thread3(xbee_thread); 00205 00206 while (1) 00207 { 00208 switch (gameState) 00209 { 00210 case START: 00211 if (pc.writeable()){ 00212 pc.printf("%c%c%c%c%c", 0, 0, 0, 0, 0); 00213 ball.setVxDir(true); 00214 ball.setVyDir(true); 00215 gameState = WAIT; 00216 } 00217 break; 00218 case GAME_SETUP: 00219 ball.reset(gameCenterX-(ballSize/2), gameCenterY-(ballSize/2), 0, 6); 00220 botPaddle.reset(gameCenterX-(paddleLength/2), gameHighY); 00221 topPaddle.reset(gameCenterX-(paddleLength/2), gameLowY); 00222 if (pc.writeable()){ 00223 pc.printf("%c%c%c%c%c", 3, botScore, topScore, 0, 0); 00224 ready = false; 00225 srand(i); 00226 gameState = GAME; 00227 Thread::wait(2000); 00228 } 00229 break; 00230 case GAME: 00231 if (pc.writeable()) { 00232 pc.printf("%c%c%c%c%c", 4, botPaddle.getX(), topPaddle.getX(), ball.getX(), ball.getY()); 00233 00234 uint8_t size = ball.getSize(); //stored in a temp variable because used a lot 00235 00236 if (ball.getFutureX() <= gameLowX){ 00237 ball.reverseXDirection(); 00238 } 00239 else if (ball.getFutureX() + size >= gameHighX){ 00240 ball.reverseXDirection(); 00241 } 00242 00243 if (topPaddle.checkHit(ball.getFutureX(), ball.getFutureY(), size)) { 00244 ball.reverseYDirection(); 00245 uint8_t fx = ball.getFutureX(); 00246 uint8_t newVx = topPaddle.returnAngle(fx, size); 00247 uint8_t newVy = 6 - newVx; 00248 ball.setVx(newVx); 00249 ball.setVy(newVy); 00250 ball.setVxDir(topPaddle.returnDir(fx, size)); 00251 } 00252 else if (botPaddle.checkHit(ball.getFutureX(), ball.getFutureY(), size)) { 00253 ball.reverseYDirection(); 00254 uint8_t fx = ball.getFutureX(); 00255 uint8_t newVx = botPaddle.returnAngle(fx, size); 00256 uint8_t newVy = 6 - newVx; 00257 ball.setVx(newVx); 00258 ball.setVy(newVy); 00259 ball.setVxDir(botPaddle.returnDir(fx, size)); 00260 } 00261 00262 if (ball.getY() < gameLowY){ 00263 botScore++; 00264 ball.setVyDir(false); 00265 gameState = GAME_SETUP; 00266 } 00267 else if (ball.getY() + size > gameHighY){ 00268 topScore++; 00269 ball.setVyDir(true); 00270 gameState = GAME_SETUP; 00271 } 00272 00273 if (botScore >= 5) { 00274 gameState = WIN; 00275 } 00276 else if (topScore >= 5) { 00277 gameState = LOSE; 00278 } 00279 00280 ball.update(); 00281 botMove = -joystick.yAxis(); 00282 if ((botMove < -0.1 || botMove > 0.1) && ball.getY() < gameHighY - 50) { 00283 botPaddle.move(botMove); 00284 } 00285 } 00286 break; 00287 case LOSE: 00288 if (pc.writeable()){ 00289 pc.printf("%c%c%c%c%c", 5, 0, 0, 0, 0); 00290 botScore = 0; 00291 topScore = 0; 00292 Thread::wait(5000); 00293 gameState = START; 00294 } 00295 break; 00296 case WIN: 00297 if (pc.writeable()){ 00298 pc.printf("%c%c%c%c%c", 6, 0, 0, 0, 0); 00299 botScore = 0; 00300 topScore = 0; 00301 Thread::wait(5000); 00302 gameState = START; 00303 } 00304 break; 00305 case WAIT: 00306 if (pc.writeable()){ 00307 // Used to seed the rand() function so the ball has a random starting direction 00308 i++; 00309 if (joystick.button()) 00310 ready = true; 00311 if (ready){ 00312 pc.printf("%c%c%c%c%c", 2, 0, 0, 0, 0); 00313 } 00314 else { 00315 pc.printf("%c%c%c%c%c", 1, 0, 0, 0, 0); 00316 } 00317 } 00318 break; 00319 } 00320 Thread::wait(20); 00321 } 00322 }
Generated on Mon Jul 18 2022 23:07:48 by
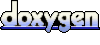