
player 1
Dependencies: 4DGL-uLCD-SE PinDetect SparkfunAnalogJoystick mbed-rtos mbed SDFileSystem
Fork of 4180FinalLab by
huzzah.cpp
00001 #include "huzzah.h" 00002 #include "mbed.h" 00003 #include "rtos.h" 00004 00005 Huzzah::Huzzah(char *enterssid, char *enterpassword, Serial *esp) { 00006 ssid = enterssid; // enter WiFi router ssid inside the quotes 00007 pwd = enterpassword; 00008 this->esp = esp; 00009 //ESP=esp; // enter WiFi router password inside the quotes 00010 } 00011 //set ESP baudrate if necessary 00012 void Huzzah::setbaudrate() 00013 { 00014 strcpy(snd, "AT+CIOBAUD=115200\r\n"); // change the numeric value to the required baudrate 00015 SendCMD(); 00016 } 00017 //Configurate ESP to Station mode and set username and password 00018 void Huzzah::config() 00019 { 00020 strcpy(snd,".\r\n.\r\n"); 00021 SendCMD(); 00022 Thread::wait(1000); 00023 00024 strcpy(snd,"node.restart()\r\n"); 00025 SendCMD(); 00026 Thread::wait(3000); 00027 00028 strcpy(snd, "wifi.setmode(wifi.STATION)\r\n"); 00029 SendCMD(); 00030 Thread::wait(2000); 00031 00032 strcpy(snd, "wifi.sta.config(\""); 00033 strcat(snd, ssid); 00034 strcat(snd, "\",\""); 00035 strcat(snd, pwd); 00036 strcat(snd, "\")\r\n"); 00037 SendCMD(); 00038 Thread::wait(2000); 00039 } 00040 00041 void Huzzah::SendCMD() 00042 { 00043 esp->printf("%s", snd); 00044 } 00045 00046 void Huzzah::sendwebpage(int _highscore1, int _highscore2) 00047 { 00048 this->_highscore1=_highscore1; 00049 this->_highscore2=_highscore2; 00050 00051 strcpy(snd, "srv=net.createServer(net.TCP)\r\n"); 00052 SendCMD(); 00053 Thread::wait(500); 00054 strcpy(snd, "srv:listen(80,function(conn)\r\n"); 00055 SendCMD(); 00056 Thread::wait(500); 00057 strcpy(snd, "conn:on(\"receive\",function(conn,payload)\r\n"); 00058 SendCMD(); 00059 Thread::wait(500); 00060 strcpy(snd, "print(payload)\r\n"); 00061 SendCMD(); 00062 Thread::wait(500); 00063 00064 strcpy(snd, "conn:send(\"<!DOCTYPE html>\")\r\n"); 00065 SendCMD(); 00066 Thread::wait(500); 00067 00068 strcpy(snd, "conn:send(\"<html>\")\r\n"); 00069 SendCMD(); 00070 Thread::wait(500); 00071 00072 strcpy(snd, "conn:send(\"<h1> PVP Wireless Pong High Scores</h1>\")\r\n"); 00073 SendCMD(); 00074 Thread::wait(500); 00075 00076 sprintf(snd, "conn:send(\"<h2> Player 1 high score: %i, Player 2 High Score: %i </h2>\")\r\n",_highscore1, _highscore2); 00077 SendCMD(); 00078 Thread::wait(500); 00079 00080 strcpy(snd, "conn:send(\"<h2> Great Job!</h2>\")\r\n"); 00081 SendCMD(); 00082 Thread::wait(500); 00083 00084 strcpy(snd, "conn:send(\"</html>\")\r\n"); 00085 SendCMD(); 00086 Thread::wait(500); 00087 00088 strcpy(snd, "end)\r\n"); 00089 SendCMD(); 00090 Thread::wait(500); 00091 00092 strcpy(snd, "conn:on(\"sent\",function(conn) conn:close() end)\r\n"); 00093 SendCMD(); 00094 Thread::wait(500); 00095 00096 strcpy(snd, "end)\r\n"); 00097 SendCMD(); 00098 Thread::wait(500); 00099 00100 }
Generated on Mon Jul 18 2022 23:07:48 by
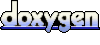