
player 1
Dependencies: 4DGL-uLCD-SE PinDetect SparkfunAnalogJoystick mbed-rtos mbed SDFileSystem
Fork of 4180FinalLab by
ball.h
00001 #include "uLCD_4DGL.h" 00002 00003 class Ball 00004 { 00005 public: 00006 Ball (uint8_t, uint8_t, uint8_t); 00007 // Set Functions 00008 void setVx(uint8_t); // This sets the velocity 00009 void setVxDir(bool); 00010 void setVy(uint8_t); // This sets the velocity 00011 void setVyDir(bool); 00012 // Get Functions 00013 uint8_t getSize(); 00014 uint8_t getX(); 00015 uint8_t getY(); 00016 uint8_t getFutureX(); // get estimate of where the ball will be in the next update() 00017 uint8_t getFutureY(); // get estimate of where the ball will be in the next update() 00018 // Member Functions 00019 void reverseXDirection(); // negate the sign for when a ball hits something 00020 void reverseYDirection(); // negate the sign for when a ball hits something 00021 void reset(uint8_t, uint8_t, int, int); // takes in a new location and new directions and draws the starting point for the ball 00022 void update(); // moves the ball on the screen one vx and vy 00023 00024 private: 00025 uint8_t vx; 00026 bool vxDir; //false is left (-x), true is right (+x) 00027 uint8_t vy; 00028 bool vyDir; //false is up (-y), true is down (+y) 00029 uint8_t x; 00030 uint8_t y; 00031 uint8_t diameter; 00032 };
Generated on Mon Jul 18 2022 23:07:48 by
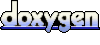