
player 1
Dependencies: 4DGL-uLCD-SE PinDetect SparkfunAnalogJoystick mbed-rtos mbed SDFileSystem
Fork of 4180FinalLab by
ball.cpp
00001 #include "ball.h" 00002 00003 Ball::Ball(uint8_t initx, uint8_t inity, uint8_t size){ 00004 x = initx; 00005 y = inity; 00006 diameter = size; 00007 } 00008 00009 void Ball::setVx(uint8_t newvx){ 00010 vx = newvx; 00011 } 00012 00013 void Ball::setVxDir(bool dir){ 00014 vxDir = dir; 00015 } 00016 00017 void Ball::setVy(uint8_t newVy){ 00018 vy = newVy; 00019 } 00020 00021 void Ball::setVyDir(bool dir){ 00022 vyDir = dir; 00023 } 00024 00025 uint8_t Ball::getSize(){ 00026 return diameter; 00027 } 00028 00029 uint8_t Ball::getX(){ 00030 return (uint8_t)x; 00031 } 00032 00033 uint8_t Ball::getY(){ 00034 return (uint8_t)y; 00035 } 00036 00037 uint8_t Ball::getFutureX(){ 00038 if (vxDir) 00039 return x+vx; 00040 else 00041 return x-vx; 00042 } 00043 00044 uint8_t Ball::getFutureY(){ 00045 if (vyDir) 00046 return y+vy; 00047 else 00048 return y-vy; 00049 } 00050 00051 void Ball::reverseXDirection(){ 00052 vxDir = !vxDir; 00053 } 00054 00055 void Ball::reverseYDirection(){ 00056 vyDir = !vyDir; 00057 } 00058 00059 void Ball::reset(uint8_t newx, uint8_t newy, int newvx, int newvy){ 00060 x = newx; 00061 y = newy; 00062 vx = newvx; 00063 vy = newvy; 00064 } 00065 00066 void Ball::update(){ 00067 if (vxDir) 00068 x = x+vx; 00069 else 00070 x = x-vx; 00071 00072 if (vyDir) 00073 y = y+vy; 00074 else 00075 y = y-vy; 00076 }
Generated on Mon Jul 18 2022 23:07:48 by
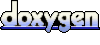