Demo program for library named SD_PlayerSkeleton of SD card player skeleton. SD カードプレーヤのための骨組みとして使うためのライブラリ SD_PlayerSkeleton の使用例.このプログラムについては,CQ出版社インターフェース誌 2018年7月号で解説している.
Dependencies: F746_GUI F746_SAI_IO SD_PlayerSkeleton
spi_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SPI_API_H 00017 #define MBED_SPI_API_H 00018 00019 #include "device.h" 00020 #include "dma_api.h" 00021 #include "buffer.h" 00022 00023 #if DEVICE_SPI 00024 00025 #define SPI_EVENT_ERROR (1 << 1) 00026 #define SPI_EVENT_COMPLETE (1 << 2) 00027 #define SPI_EVENT_RX_OVERFLOW (1 << 3) 00028 #define SPI_EVENT_ALL (SPI_EVENT_ERROR | SPI_EVENT_COMPLETE | SPI_EVENT_RX_OVERFLOW) 00029 00030 #define SPI_EVENT_INTERNAL_TRANSFER_COMPLETE (1 << 30) // internal flag to report an event occurred 00031 00032 #define SPI_FILL_WORD (0xFFFF) 00033 00034 #if DEVICE_SPI_ASYNCH 00035 /** Asynch spi hal structure 00036 */ 00037 typedef struct { 00038 struct spi_s spi; /**< Target specific spi structure */ 00039 struct buffer_s tx_buff; /**< Tx buffer */ 00040 struct buffer_s rx_buff; /**< Rx buffer */ 00041 } spi_t; 00042 00043 #else 00044 /** Non-asynch spi hal structure 00045 */ 00046 typedef struct spi_s spi_t; 00047 00048 #endif 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 /** 00055 * \defgroup GeneralSPI SPI Configuration Functions 00056 * @{ 00057 */ 00058 00059 /** Initialize the SPI peripheral 00060 * 00061 * Configures the pins used by SPI, sets a default format and frequency, and enables the peripheral 00062 * @param[out] obj The SPI object to initialize 00063 * @param[in] mosi The pin to use for MOSI 00064 * @param[in] miso The pin to use for MISO 00065 * @param[in] sclk The pin to use for SCLK 00066 * @param[in] ssel The pin to use for SSEL 00067 */ 00068 void spi_init(spi_t *obj, PinName mosi, PinName miso, PinName sclk, PinName ssel); 00069 00070 /** Release a SPI object 00071 * 00072 * TODO: spi_free is currently unimplemented 00073 * This will require reference counting at the C++ level to be safe 00074 * 00075 * Return the pins owned by the SPI object to their reset state 00076 * Disable the SPI peripheral 00077 * Disable the SPI clock 00078 * @param[in] obj The SPI object to deinitialize 00079 */ 00080 void spi_free(spi_t *obj); 00081 00082 /** Configure the SPI format 00083 * 00084 * Set the number of bits per frame, configure clock polarity and phase, shift order and master/slave mode 00085 * @param[in,out] obj The SPI object to configure 00086 * @param[in] bits The number of bits per frame 00087 * @param[in] mode The SPI mode (clock polarity, phase, and shift direction) 00088 * @param[in] slave Zero for master mode or non-zero for slave mode 00089 */ 00090 void spi_format(spi_t *obj, int bits, int mode, int slave); 00091 00092 /** Set the SPI baud rate 00093 * 00094 * Actual frequency may differ from the desired frequency due to available dividers and bus clock 00095 * Configures the SPI peripheral's baud rate 00096 * @param[in,out] obj The SPI object to configure 00097 * @param[in] hz The baud rate in Hz 00098 */ 00099 void spi_frequency(spi_t *obj, int hz); 00100 00101 /**@}*/ 00102 /** 00103 * \defgroup SynchSPI Synchronous SPI Hardware Abstraction Layer 00104 * @{ 00105 */ 00106 00107 /** Write a byte out in master mode and receive a value 00108 * 00109 * @param[in] obj The SPI peripheral to use for sending 00110 * @param[in] value The value to send 00111 * @return Returns the value received during send 00112 */ 00113 int spi_master_write(spi_t *obj, int value); 00114 00115 /** Check if a value is available to read 00116 * 00117 * @param[in] obj The SPI peripheral to check 00118 * @return non-zero if a value is available 00119 */ 00120 int spi_slave_receive(spi_t *obj); 00121 00122 /** Get a received value out of the SPI receive buffer in slave mode 00123 * 00124 * Blocks until a value is available 00125 * @param[in] obj The SPI peripheral to read 00126 * @return The value received 00127 */ 00128 int spi_slave_read(spi_t *obj); 00129 00130 /** Write a value to the SPI peripheral in slave mode 00131 * 00132 * Blocks until the SPI peripheral can be written to 00133 * @param[in] obj The SPI peripheral to write 00134 * @param[in] value The value to write 00135 */ 00136 void spi_slave_write(spi_t *obj, int value); 00137 00138 /** Checks if the specified SPI peripheral is in use 00139 * 00140 * @param[in] obj The SPI peripheral to check 00141 * @return non-zero if the peripheral is currently transmitting 00142 */ 00143 int spi_busy(spi_t *obj); 00144 00145 /** Get the module number 00146 * 00147 * @param[in] obj The SPI peripheral to check 00148 * @return The module number 00149 */ 00150 uint8_t spi_get_module(spi_t *obj); 00151 00152 /**@}*/ 00153 00154 #if DEVICE_SPI_ASYNCH 00155 /** 00156 * \defgroup AsynchSPI Asynchronous SPI Hardware Abstraction Layer 00157 * @{ 00158 */ 00159 00160 /** Begin the SPI transfer. Buffer pointers and lengths are specified in tx_buff and rx_buff 00161 * 00162 * @param[in] obj The SPI object which holds the transfer information 00163 * @param[in] tx The buffer to send 00164 * @param[in] tx_length The number of words to transmit 00165 * @param[in] rx The buffer to receive 00166 * @param[in] rx_length The number of words to receive 00167 * @param[in] bit_width The bit width of buffer words 00168 * @param[in] event The logical OR of events to be registered 00169 * @param[in] handler SPI interrupt handler 00170 * @param[in] hint A suggestion for how to use DMA with this transfer 00171 */ 00172 void spi_master_transfer(spi_t *obj, const void *tx, size_t tx_length, void *rx, size_t rx_length, uint8_t bit_width, uint32_t handler, uint32_t event, DMAUsage hint); 00173 00174 /** The asynchronous IRQ handler 00175 * 00176 * Reads the received values out of the RX FIFO, writes values into the TX FIFO and checks for transfer termination 00177 * conditions, such as buffer overflows or transfer complete. 00178 * @param[in] obj The SPI object which holds the transfer information 00179 * @return event flags if a transfer termination condition was met or 0 otherwise. 00180 */ 00181 uint32_t spi_irq_handler_asynch(spi_t *obj); 00182 00183 /** Attempts to determine if the SPI peripheral is already in use. 00184 * 00185 * If a temporary DMA channel has been allocated, peripheral is in use. 00186 * If a permanent DMA channel has been allocated, check if the DMA channel is in use. If not, proceed as though no DMA 00187 * channel were allocated. 00188 * If no DMA channel is allocated, check whether tx and rx buffers have been assigned. For each assigned buffer, check 00189 * if the corresponding buffer position is less than the buffer length. If buffers do not indicate activity, check if 00190 * there are any bytes in the FIFOs. 00191 * @param[in] obj The SPI object to check for activity 00192 * @return non-zero if the SPI port is active or zero if it is not. 00193 */ 00194 uint8_t spi_active(spi_t *obj); 00195 00196 /** Abort an SPI transfer 00197 * 00198 * @param obj The SPI peripheral to stop 00199 */ 00200 void spi_abort_asynch(spi_t *obj); 00201 00202 00203 #endif 00204 00205 /**@}*/ 00206 00207 #ifdef __cplusplus 00208 } 00209 #endif // __cplusplus 00210 00211 #endif // SPI_DEVICE 00212 00213 #endif // MBED_SPI_API_H
Generated on Tue Jul 12 2022 21:30:19 by
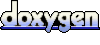