
Version using MEMS microphone and CODEC for the program "F746_RealtimeSpectrumAnalyzer". "F746_RealtimeSpectrumAnalyzer" の入力を MEMS のマイクと CODEC に変更.このプログラムは Tomona Nanase さんが作成し DISCO-F746NG_Oscilloscope の名前で登録しているプログラムで, CODEC を使って入力する部分を参考にして作成.このプログラムの説明は,CQ出版社のインターフェース誌,2016年4月号に掲載.
Dependencies: BSP_DISCO_F746NG BUTTON_GROUP LCD_DISCO_F746NG TS_DISCO_F746NG UIT_FFT_Real mbed
main.cpp
00001 //------------------------------------------------ 00002 // Realtime spectrum analyzer 00003 // Input: MEMS microphone or CN11 00004 // 00005 // 2016/04/19, Copyright (c) 2016 MIKAMI, Naoki 00006 //------------------------------------------------ 00007 00008 #include "main.h" 00009 00010 using namespace Mikami; 00011 00012 int16_t audio_in_buffer[BufferSize]; 00013 __IO bool audio_in_buffer_captured = false; 00014 __IO int32_t audio_in_buffer_offset = 0; 00015 __IO int32_t audio_in_buffer_length = 0; 00016 00017 // Switching input device 00018 void SwitchInputDevice(int sw) 00019 { 00020 uint16_t dev = (sw == 0) ? INPUT_DEVICE_DIGITAL_MICROPHONE_2 00021 : INPUT_DEVICE_INPUT_LINE_1; 00022 InitRunAudioIn(dev, I2S_AUDIOFREQ_16K); 00023 } 00024 00025 int main() 00026 { 00027 const int FS = 8000; // Sampling frequency: 8 kHz 00028 const int N_DATA = N_DATA_; // Number of data to be analyzed 00029 const int N_FFT = 512; // Number of date for FFT 00030 00031 const int X_WAV = 44; // Origin for x axis of waveform 00032 const int Y_WAV = 36; // Origin for y axis of waveform 00033 const int X0 = 40; // Origin for x axis of spectrum 00034 const int Y0 = 234; // Origin for y axis of spectrum 00035 const float DB1 = 2.4f; // Pixels for 1 dB 00036 const int BIN = 1; // Pixels per bin 00037 const int W_DB = 60; // Range in dB to be displayed 00038 const int X0_BTN = 340; // Holizontal position for left of buttons 00039 00040 const uint32_t BACK_COLOR = 0xFF006A6C; // Teal green 00041 const uint32_t INACTIVE_COLOR = BACK_COLOR & 0xD0FFFFFF; 00042 const uint32_t TOUCHED_COLOR = 0xFF7F7FFF; 00043 const uint32_t ORIGINAL_COLOR = 0xFF0068B7; 00044 const uint32_t INACTIVE_TEXT_COLOR = LCD_COLOR_GRAY; 00045 const uint32_t AXIS_COLOR = 0xFFCCFFFF; 00046 const uint32_t LINE_COLOR = LCD_COLOR_CYAN; 00047 00048 LCD_DISCO_F746NG lcd; // Object for LCD display 00049 TS_DISCO_F746NG ts; // Object for touch pannel 00050 00051 lcd.Clear(BACK_COLOR); 00052 00053 // Setting of button group 00054 const string RUN_STOP[2] = {"RUN", "STOP"}; 00055 ButtonGroup runStop(lcd, ts, X0_BTN, 10, 60, 40, 00056 ORIGINAL_COLOR, BACK_COLOR, 00057 2, RUN_STOP, 5, 0, 2); 00058 runStop.Draw(1, INACTIVE_COLOR, INACTIVE_TEXT_COLOR); 00059 00060 const string MIC_LINE[2] = {"MIC", "LINE"}; 00061 ButtonGroup micLine(lcd, ts, X0_BTN, 65, 60, 40, 00062 ORIGINAL_COLOR, BACK_COLOR, 00063 2, MIC_LINE, 5, 0, 2); 00064 micLine.DrawAll(INACTIVE_COLOR, INACTIVE_TEXT_COLOR); 00065 00066 const string METHOD[3] = {"FFT", "Linear Pred.", "Cepstrum"}; 00067 ButtonGroup method(lcd, ts, X0_BTN, 120, 125, 40, 00068 ORIGINAL_COLOR, BACK_COLOR, 00069 3, METHOD, 0, 5, 1); 00070 method.DrawAll(INACTIVE_COLOR, INACTIVE_TEXT_COLOR); 00071 // End of button group setting 00072 00073 WaveformDisplay waveDisp(lcd, X_WAV, Y_WAV, N_DATA, 9, 00074 AXIS_COLOR, LINE_COLOR, BACK_COLOR); 00075 00076 SpectrumDisplay disp(lcd, N_FFT, X0, Y0, -45, DB1, BIN, W_DB, FS, 00077 AXIS_COLOR, LINE_COLOR, BACK_COLOR); 00078 // Linear prediction: order = 10 00079 // Cepstral smoothing: highest quefrency = 40 00080 Selector analyzer(disp, N_DATA, N_FFT, 10, 40); 00081 00082 // Initialize the infinite loop procedure 00083 int select = -1; 00084 int sw = 0; // 0: mic, 1: line 00085 int stop = 0; 00086 int16_t sn[N_DATA]; 00087 00088 // Wait for "RUN" button touched 00089 while (!runStop.Touched(0, TOUCHED_COLOR)) {} 00090 00091 // Start of spectrum analyzing 00092 method.DrawAll(ORIGINAL_COLOR); 00093 00094 while (true) 00095 { 00096 if (runStop.Touched(0, TOUCHED_COLOR)) 00097 { 00098 SwitchInputDevice(sw); 00099 micLine.Draw(sw, TOUCHED_COLOR); 00100 micLine.Redraw(1-sw); 00101 } 00102 00103 runStop.GetTouchedNumber(stop, TOUCHED_COLOR); 00104 if (stop == 0) 00105 { 00106 if (micLine.GetTouchedNumber(sw, TOUCHED_COLOR)) 00107 SwitchInputDevice(sw); 00108 00109 if (audio_in_buffer_captured) 00110 { 00111 // Waveform display 00112 int32_t index = audio_in_buffer_offset; // right mic. or left line 00113 // int32_t index = audio_in_buffer_offset + 1; // left mic. or right line 00114 Decimate(N_DATA, index, audio_in_buffer, sn); 00115 waveDisp.Execute(sn); 00116 // Spectrum analysis and display 00117 analyzer.Execute(sn, select); 00118 audio_in_buffer_captured = false; 00119 } 00120 else 00121 if (method.GetTouchedNumber(select, TOUCHED_COLOR)) 00122 analyzer.Execute(sn, select); 00123 } 00124 else 00125 { 00126 if (runStop.Touched(1)) 00127 { 00128 StopAudioIn(); // Stop sampling 00129 micLine.DrawAll(INACTIVE_COLOR, INACTIVE_TEXT_COLOR); 00130 } 00131 if (method.GetTouchedNumber(select, TOUCHED_COLOR)) 00132 analyzer.Execute(sn, select); 00133 } 00134 } 00135 }
Generated on Sun Jul 17 2022 12:37:24 by
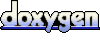