
Version using MEMS microphone and CODEC for the program "F746_RealtimeSpectrumAnalyzer". "F746_RealtimeSpectrumAnalyzer" の入力を MEMS のマイクと CODEC に変更.このプログラムは Tomona Nanase さんが作成し DISCO-F746NG_Oscilloscope の名前で登録しているプログラムで, CODEC を使って入力する部分を参考にして作成.このプログラムの説明は,CQ出版社のインターフェース誌,2016年4月号に掲載.
Dependencies: BSP_DISCO_F746NG BUTTON_GROUP LCD_DISCO_F746NG TS_DISCO_F746NG UIT_FFT_Real mbed
WaveformDisplay.hpp
00001 //----------------------------------------------------------- 00002 // Class for waveform display 00003 // 00004 // 2015/12/15, Copyright (c) 2015 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #ifndef F746_WAVEFORM_DISPLAY_HPP 00008 #define F746_WAVEFORM_DISPLAY_HPP 00009 00010 #include "mbed.h" 00011 00012 namespace Mikami 00013 { 00014 class WaveformDisplay 00015 { 00016 public: 00017 WaveformDisplay(LCD_DISCO_F746NG &lcd, 00018 uint16_t x0, uint16_t y0, int nData, 00019 uint16_t rShift, 00020 uint32_t axisColor, uint32_t lineColor, 00021 uint32_t backColor) 00022 : X0_(x0), Y0_(y0), N_DATA_(nData), R_SHIFT_(rShift), 00023 AXIS_COLOR_(axisColor), LINE_COLOR_(lineColor), 00024 BACK_COLOR_(backColor), lcd_(lcd) { Axis(); } 00025 00026 void Execute(const int16_t xn[]) 00027 { 00028 static const uint16_t LIMIT1 = Y0_ + LIMIT2_; 00029 static const uint16_t LIMIT2 = Y0_ - LIMIT2_; 00030 Axis(); 00031 lcd_.SetTextColor(LINE_COLOR_); 00032 uint16_t x1 = X0_; 00033 uint16_t y1 = Clip(xn[0]); 00034 for (int n=1; n<N_DATA_; n++) 00035 { 00036 uint16_t x2 = X0_ + n; 00037 uint16_t y2 = Clip(xn[n]); 00038 if ( ((y2 == LIMIT1) && (y1 == LIMIT1)) || 00039 ((y2 == LIMIT2) && (y1 == LIMIT2)) ) 00040 { // Out of displaying boundaries 00041 lcd_.SetTextColor(LCD_COLOR_RED); 00042 lcd_.DrawHLine(x1, y1, 1); 00043 lcd_.SetTextColor(LINE_COLOR_); 00044 } 00045 else 00046 lcd_.DrawLine(x1, y1, x2, y2); 00047 if ((y1 == LIMIT1) || (y1 == LIMIT2)) 00048 lcd_.DrawPixel(x1, y1, LCD_COLOR_RED); 00049 x1 = x2; 00050 y1 = y2; 00051 } 00052 lcd_.SetTextColor(BACK_COLOR_); 00053 } 00054 00055 private: 00056 const uint16_t X0_; 00057 const uint16_t Y0_; 00058 const int N_DATA_; 00059 const uint16_t R_SHIFT_; 00060 const uint32_t AXIS_COLOR_; 00061 const uint32_t LINE_COLOR_; 00062 const uint32_t BACK_COLOR_; 00063 static const int LIMIT_ = 32; 00064 static const int LIMIT2_ = LIMIT_ + 1; 00065 00066 LCD_DISCO_F746NG& lcd_; 00067 00068 // Clipping 00069 uint16_t Clip(int16_t xn) 00070 { 00071 int16_t x = xn >> R_SHIFT_; 00072 if (x > LIMIT_ ) x = LIMIT2_; 00073 if (x < -LIMIT_ ) x = -LIMIT2_ ; 00074 return Y0_ - x; 00075 } 00076 00077 void Axis() 00078 { 00079 lcd_.SetTextColor(BACK_COLOR_); 00080 lcd_.FillRect(X0_, Y0_-LIMIT2_, N_DATA_, LIMIT2_*2+1); 00081 00082 lcd_.SetTextColor(AXIS_COLOR_); 00083 lcd_.DrawLine(X0_-5, Y0_, X0_+N_DATA_+5, Y0_); 00084 } 00085 00086 // disallow copy constructor and assignment operator 00087 WaveformDisplay(const WaveformDisplay& ); 00088 WaveformDisplay& operator=(const WaveformDisplay& ); 00089 }; 00090 } 00091 #endif // F746_WAVEFORM_DISPLAY_HPP
Generated on Sun Jul 17 2022 12:37:24 by
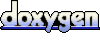