
Version using MEMS microphone and CODEC for the program "F746_RealtimeSpectrumAnalyzer". "F746_RealtimeSpectrumAnalyzer" の入力を MEMS のマイクと CODEC に変更.このプログラムは Tomona Nanase さんが作成し DISCO-F746NG_Oscilloscope の名前で登録しているプログラムで, CODEC を使って入力する部分を参考にして作成.このプログラムの説明は,CQ出版社のインターフェース誌,2016年4月号に掲載.
Dependencies: BSP_DISCO_F746NG BUTTON_GROUP LCD_DISCO_F746NG TS_DISCO_F746NG UIT_FFT_Real mbed
SpectrumDisplay.hpp
00001 //------------------------------------------------------- 00002 // Class for display spectrum (Header) 00003 // 00004 // 2016/01/07, Copyright (c) 2015 MIKAMI, Naoki 00005 //------------------------------------------------------- 00006 00007 #ifndef SPECTRUM_DISPLAY_HPP 00008 #define SPECTRUM_DISPLAY_HPP 00009 00010 #include "LCD_DISCO_F746NG.h" 00011 00012 namespace Mikami 00013 { 00014 class SpectrumDisplay 00015 { 00016 public: 00017 SpectrumDisplay(LCD_DISCO_F746NG &lcd, 00018 int nFft, int x0, int y0, float offset, 00019 float db1, int bin, float maxDb, int fs, 00020 uint32_t axisColor, uint32_t lineColor, 00021 uint32_t backColor); 00022 void Draw(float db[]); 00023 void Clear(); 00024 00025 private: 00026 00027 const int N_FFT_; // number of date for FFT 00028 const int X0_; // Origin for x axis 00029 const int Y0_; // Origin for y axis 00030 const float OFFSET_; // Offset for display 00031 const float DB1_; // Pixels for 1 dB 00032 const int BIN_; // Pixels per bin 00033 const float MAX_DB_; // Maximum dB 00034 const int FS_; // Sampling frequency: 10 kHz 00035 const uint32_t AXIS_COLOR_; 00036 const uint32_t LINE_COLOR_; 00037 const uint32_t BACK_COLOR_; 00038 00039 LCD_DISCO_F746NG& lcd_; 00040 00041 void AxisX(); // x-axis 00042 void AxisY(); // y-axis 00043 00044 void DrawString(uint16_t x, uint16_t y, char str[]) 00045 { lcd_.DisplayStringAt(x, y, (uint8_t *)str, LEFT_MODE); } 00046 00047 // disallow copy constructor and assignment operator 00048 SpectrumDisplay(const SpectrumDisplay& ); 00049 SpectrumDisplay& operator=(const SpectrumDisplay& ); 00050 }; 00051 } 00052 #endif // SPECTRUM_DISPLAY_HPP
Generated on Sun Jul 17 2022 12:37:24 by
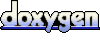