Library to provide a mechanism to make it easier to add RPC to custom code by using RPCFunction and RPCVariable objects. Also includes a class to receive and process RPC over serial.
Dependents: GSL_10-Pololu_A4983_STEPMOTORDRIVER Protodrive RPC_HTTP RPC_TestHack ... more
SerialRPCInterface.cpp
00001 /** 00002 * @section LICENSE 00003 *Copyright (c) 2010 ARM Ltd. 00004 * 00005 *Permission is hereby granted, free of charge, to any person obtaining a copy 00006 *of this software and associated documentation files (the "Software"), to deal 00007 *in the Software without restriction, including without limitation the rights 00008 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 *copies of the Software, and to permit persons to whom the Software is 00010 *furnished to do so, subject to the following conditions: 00011 * 00012 *The above copyright notice and this permission notice shall be included in 00013 *all copies or substantial portions of the Software. 00014 * 00015 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 *THE SOFTWARE. 00022 * 00023 * 00024 * @section DESCRIPTION 00025 * 00026 *This class sets up RPC communication. This allows objects on mbed to be controlled. Objects can be created or existing objects can be used 00027 * 00028 * Substantially updated Jan 2016 to work with the updated RPC system 00029 */ 00030 #include "SerialRPCInterface.h" 00031 00032 using namespace mbed; 00033 00034 //Requires multiple contstructors for each type, serial to set different pin numbers, TCP for port. 00035 SerialRPCInterface::SerialRPCInterface(PinName tx, PinName rx, int baud):pc(tx, rx) { 00036 _RegClasses(); 00037 _enabled = true; 00038 pc.attach(this, &SerialRPCInterface::_RPCSerial, Serial::RxIrq); 00039 if(baud != 9600)pc.baud(baud); 00040 } 00041 00042 void SerialRPCInterface::_RegClasses(void){ 00043 00044 //Register classes with base 00045 #if DEVICE_ANALOGIN 00046 //RPC::add_rpc_class<RpcAnalogIn>(); 00047 #endif 00048 RPC::add_rpc_class<RpcDigitalIn>(); 00049 RPC::add_rpc_class<RpcDigitalOut>(); 00050 RPC::add_rpc_class<RpcDigitalInOut>(); 00051 RPC::add_rpc_class<RpcPwmOut>(); 00052 RPC::add_rpc_class<RpcTimer>(); 00053 //RPC::add_rpc_class<RpcBusOut>(); 00054 //RPC::add_rpc_class<RpcBusIn>(); 00055 //RPC::add_rpc_class<RpcBusInOut>(); 00056 RPC::add_rpc_class<RpcSerial>(); 00057 00058 //AnalogOut not avaliable on mbed LPC11U24 so only compile for other devices 00059 #if DEVICE_ANALOGOUT 00060 //RPC::add_rpc_class<RpcAnalogOut>(); 00061 #endif 00062 00063 } 00064 00065 void SerialRPCInterface::Disable(void){ 00066 _enabled = false; 00067 } 00068 void SerialRPCInterface::Enable(void){ 00069 _enabled = true; 00070 } 00071 void SerialRPCInterface::_MsgProcess(void) { 00072 if(_enabled == true){ 00073 RPC::call(_command, _response); 00074 } 00075 } 00076 00077 void SerialRPCInterface::_RPCSerial() { 00078 _RPCflag = true; 00079 if(_enabled == true){ 00080 pc.gets(_command, 256); 00081 _MsgProcess(); 00082 pc.printf("%s\n", _response); 00083 } 00084 _RPCflag = false; 00085 }
Generated on Tue Jul 12 2022 13:34:52 by
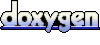