
firmware update from USB stick, does not work properly. If someone can get it work, please inform me.
Embed:
(wiki syntax)
Show/hide line numbers
FirmwareUpdater.cpp
00001 /** 00002 * ============================================================================= 00003 * Firmware updater (Version 0.0.1) 00004 * ============================================================================= 00005 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, including without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 * ============================================================================= 00025 */ 00026 00027 #include "FirmwareUpdater.h" 00028 00029 #include <stdio.h> 00030 #include <stdarg.h> 00031 00032 extern "C" void mbed_reset(); 00033 00034 const std::string FirmwareUpdater::EXT_BIN = ".bin"; 00035 const std::string FirmwareUpdater::EXT_BINTMP = ".b__"; 00036 const std::string FirmwareUpdater::EXT_TXT = ".txt"; 00037 const std::string FirmwareUpdater::EXT_TXTTMP = ".t__"; 00038 00039 MSCFileSystem fs ("fs"); 00040 LocalFileSystem local("local"); 00041 00042 00043 /** 00044 * Create. 00045 * 00046 * @param src_name: An application name on USB Stick. Do not include a extention. 00047 * @param dest_name An application name on MBED. Do not include a extention. 00048 * @param log True if logging. 00049 */ 00050 FirmwareUpdater::FirmwareUpdater(std::string src_name, std::string dest_name, bool log) 00051 : src_name(src_name), dest_name(dest_name), log(log), local("local") { 00052 00053 } 00054 00055 /* 00056 * Dispose. 00057 */ 00058 FirmwareUpdater::~FirmwareUpdater() { 00059 } 00060 00061 /** 00062 * Get the source_name. 00063 * 00064 * @return src_name. 00065 */ 00066 const std::string FirmwareUpdater:: get_src_name() const { 00067 return src_name; 00068 } 00069 00070 /** 00071 * Get the dest_name. 00072 * 00073 * @return _destname. 00074 */ 00075 const std::string FirmwareUpdater:: get_dest_name() const { 00076 return dest_name; 00077 } 00078 00079 /* 00080 * Checking a new firmware. 00081 * Compare versions of the software between local storage on mbed and on USB Stick. 00082 * 00083 * @return Return 0 if a new firmware exists. 00084 */ 00085 00086 int FirmwareUpdater::exist() { 00087 int ver_local, ver_USB; 00088 printf("Fetch the version from a local."); 00089 /* 00090 * Fetch the version from a local. 00091 */ 00092 std::string file_local = "/local/" + dest_name + EXT_TXT; 00093 FILE *fp = fopen(file_local.c_str(), "rb"); 00094 if (fp == NULL) { 00095 LOG("Local firmware version file '%s' open failed.\n\r", file_local.c_str()); 00096 return -1; 00097 } 00098 if (fscanf(fp, "%d", &ver_local) != 1) { 00099 LOG("Local firmware version file '%s' is invalid.\n\r", file_local.c_str()); 00100 return -2; 00101 } 00102 fclose(fp); 00103 LOG("Local firmware version is %d. (%s)\n\r", ver_local, file_local.c_str()); 00104 00105 /* 00106 * Fetch the version from USB Stick. 00107 */ 00108 printf("Fetch the version from USB Stick.\r"); 00109 std::string file_USB = "/fs/" + src_name + EXT_TXT; 00110 fp = fopen(file_USB.c_str(), "rb"); 00111 00112 if (fp == NULL) { 00113 LOG("Source firmware version file '%s' open failed.\n\r", file_USB.c_str()); 00114 return -1; 00115 } 00116 if (fscanf(fp, "%d", &ver_USB) != 1) { 00117 LOG("Source firmware version file '%s' is invalid.\n\r", file_USB.c_str()); 00118 return -2; 00119 } 00120 fclose(fp); 00121 LOG("Source firmware version is %d. (%s)\n\r", ver_USB, file_USB.c_str()); 00122 00123 00124 00125 return (ver_local < ver_USB) ? 0 : 1; 00126 } 00127 00128 /** 00129 * Execute update. 00130 * 00131 * @return Return 0 if it succeed. 00132 */ 00133 int FirmwareUpdater::execute() { 00134 /* 00135 * Fetch the files. 00136 */ 00137 printf("Start execute\r"); 00138 std::string USB_txt = "/fs/" + src_name + EXT_TXT; 00139 std::string USB_bin = "/fs/" + src_name + EXT_BIN; 00140 00141 /* 00142 std::string file_txttmp = "/local/" + name + EXT_TXTTMP; 00143 std::string file_bintmp = "/local/" + name + EXT_BINTMP; 00144 if (fetch(serv_txt, file_txttmp) != 0) { 00145 return -1; 00146 } 00147 if (fetch(serv_bin, file_bintmp) != 0) { 00148 return -2; 00149 }*/ 00150 /* 00151 * Copy it. 00152 */ 00153 00154 std::string local_txt = "/local/" + dest_name + EXT_TXT; 00155 std::string local_bin = "/local/" + dest_name + EXT_BIN; 00156 if (copy(USB_txt, local_txt) != 0) { 00157 printf("-3"); 00158 return -3; 00159 } 00160 if (copy(USB_bin, local_bin) != 0) { 00161 printf("-4"); 00162 return -4; 00163 } 00164 /* 00165 * Delete the temporary files. 00166 */ 00167 //remove(file_txttmp.c_str()); 00168 //remove(file_bintmp.c_str()); 00169 return 0; 00170 } 00171 00172 00173 /** 00174 * Reset system. 00175 */ 00176 void FirmwareUpdater::reset() { 00177 mbed_reset(); 00178 } 00179 00180 /** 00181 * Fetch a file. 00182 * 00183 * @param src_url URL of a source file. 00184 * @param local_file Local file name. 00185 * 00186 * @return Return 0 if it succeed. 00187 */ 00188 int FirmwareUpdater::fetch(std::string src_name, std::string local_file) { 00189 return (0); 00190 } 00191 00192 00193 /** 00194 * Copy a file. 00195 * 00196 * @param local_file1 Source file. 00197 * @param local_file2 Destination file. 00198 * 00199 * @return Return 0 if it succeed. 00200 */ 00201 int FirmwareUpdater::copy(std::string local_file1, std::string local_file2) { 00202 00203 LOG("File copying... (%s->%s)\n\r", local_file1.c_str(), local_file2.c_str()); 00204 00205 FILE *rp = fopen(local_file1.c_str(), "rb"); 00206 if (rp == NULL) { 00207 LOG("File '%s' open failed.\n", local_file1.c_str()); 00208 return -1; 00209 } 00210 remove(local_file2.c_str()); 00211 FILE *wp = fopen(local_file2.c_str(), "wb"); 00212 if (wp == NULL) { 00213 LOG("File '%s' open failed.\n\r", local_file2.c_str()); 00214 fclose(rp); 00215 return -2; 00216 } 00217 int c; 00218 while ((c = fgetc(rp)) != EOF) { 00219 fputc(c, wp); 00220 } 00221 fclose(rp); 00222 fclose(wp); 00223 LOG("File copied. (%s->%s)\n\r", local_file1.c_str(), local_file2.c_str()); 00224 return 0; 00225 } 00226 00227 00228 /** 00229 * Output a message to a log file. 00230 * 00231 * @param format ... 00232 */ 00233 void FirmwareUpdater::LOG(const char* format, ...) { 00234 if (log) { 00235 FILE *fplog = fopen("/local/update.log", "a"); 00236 if (fplog != NULL) { 00237 char buf[BUFSIZ]; 00238 va_list p; 00239 va_start(p, format); 00240 vsnprintf(buf, sizeof(buf) - 1, format, p); 00241 fprintf(fplog, "%s", buf); 00242 va_end(p); 00243 fclose(fplog); 00244 } 00245 } 00246 00247 00248 }
Generated on Wed Jul 13 2022 17:07:14 by
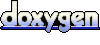