Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
unity_fixture_malloc_overrides.h
00001 //- Copyright (c) 2010 James Grenning and Contributed to Unity Project 00002 /* ========================================== 00003 Unity Project - A Test Framework for C 00004 Copyright (c) 2007 Mike Karlesky, Mark VanderVoord, Greg Williams 00005 [Released under MIT License. Please refer to license.txt for details] 00006 ========================================== */ 00007 00008 #ifndef UNITY_FIXTURE_MALLOC_OVERRIDES_H_ 00009 #define UNITY_FIXTURE_MALLOC_OVERRIDES_H_ 00010 00011 #include <stddef.h> 00012 00013 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00014 // Define this macro to remove the use of stdlib.h, malloc, and free. 00015 // Many embedded systems do not have a heap or malloc/free by default. 00016 // This internal unity_malloc() provides allocated memory deterministically from 00017 // the end of an array only, unity_free() only releases from end-of-array, 00018 // blocks are not coalesced, and memory not freed in LIFO order is stranded. 00019 #ifndef UNITY_INTERNAL_HEAP_SIZE_BYTES 00020 #define UNITY_INTERNAL_HEAP_SIZE_BYTES 256 00021 #endif 00022 #endif 00023 00024 // These functions are used by the Unity Fixture to allocate and release memory 00025 // on the heap and can be overridden with platform-specific implementations. 00026 // For example, when using FreeRTOS UNITY_FIXTURE_MALLOC becomes pvPortMalloc() 00027 // and UNITY_FIXTURE_FREE becomes vPortFree(). 00028 #if !defined(UNITY_FIXTURE_MALLOC) || !defined(UNITY_FIXTURE_FREE) 00029 #define UNITY_FIXTURE_MALLOC(size) malloc(size) 00030 #define UNITY_FIXTURE_FREE(ptr) free(ptr) 00031 #else 00032 extern void* UNITY_FIXTURE_MALLOC(size_t size); 00033 extern void UNITY_FIXTURE_FREE(void* ptr); 00034 #endif 00035 00036 #define malloc unity_malloc 00037 #define calloc unity_calloc 00038 #define realloc unity_realloc 00039 #define free unity_free 00040 00041 void* unity_malloc(size_t size); 00042 void* unity_calloc(size_t num, size_t size); 00043 void* unity_realloc(void * oldMem, size_t size); 00044 void unity_free(void * mem); 00045 00046 #endif /* UNITY_FIXTURE_MALLOC_OVERRIDES_H_ */
Generated on Tue Jul 12 2022 19:01:37 by
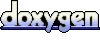