Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
sotp_no_sotp.c
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 00019 // ----------------------------------------------------------- Includes ----------------------------------------------------------- 00020 00021 #include "sotp.h" 00022 00023 #if (SYS_CONF_SOTP==SYS_CONF_SOTP_LIMITED) 00024 00025 #include "esfs.h" 00026 #include "pal.h" 00027 #include <string.h> 00028 #include <stdio.h> 00029 #include "sotp_int.h" 00030 00031 #define FILE_NAME_BASE "sotp_type_" 00032 #define FILE_NAME_SIZE (sizeof(FILE_NAME_BASE)+4) 00033 00034 STATIC bool init_done = false; 00035 00036 static const sotp_type_e otp_types[] = {SOTP_TYPE_TRUSTED_TIME_SRV_ID}; 00037 00038 // --------------------------------------------------------- Definitions ---------------------------------------------------------- 00039 00040 00041 // -------------------------------------------------- Local Functions Declaration ---------------------------------------------------- 00042 00043 // -------------------------------------------------- Functions Implementation ---------------------------------------------------- 00044 00045 // Start of API functions 00046 00047 bool sotp_is_otp_type(uint32_t type) 00048 { 00049 unsigned int i; 00050 for (i = 0; i < sizeof(otp_types) / sizeof(sotp_type_e); i++) { 00051 if (otp_types[i] == type) { 00052 return true; 00053 } 00054 } 00055 return false; 00056 } 00057 00058 sotp_result_e sotp_get(uint32_t type, uint16_t buf_len_bytes, uint32_t *buf, uint16_t *actual_len_bytes) 00059 { 00060 esfs_file_t handle; 00061 uint16_t mode; 00062 char file_name[FILE_NAME_SIZE]; 00063 esfs_result_e esfs_ret; 00064 size_t act_size; 00065 sotp_result_e ret; 00066 00067 if (!init_done) { 00068 ret = sotp_init(); 00069 if (ret != SOTP_SUCCESS) 00070 return ret; 00071 } 00072 00073 if (type > SOTP_MAX_TYPES) { 00074 return SOTP_BAD_VALUE; 00075 } 00076 00077 memset(&handle, 0, sizeof(handle)); 00078 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00079 00080 esfs_ret = esfs_open((uint8_t *)file_name, strlen(file_name), &mode, &handle); 00081 if (esfs_ret == ESFS_NOT_EXISTS) { 00082 return SOTP_NOT_FOUND; 00083 } 00084 else if (esfs_ret != ESFS_SUCCESS) { 00085 return SOTP_OS_ERROR; 00086 } 00087 00088 if (!buf) { 00089 buf_len_bytes = 0; 00090 } 00091 00092 esfs_ret = esfs_file_size(&handle, &act_size); 00093 *actual_len_bytes = (uint16_t) act_size; 00094 if (esfs_ret != ESFS_SUCCESS) { 00095 esfs_close(&handle); 00096 return SOTP_READ_ERROR; 00097 } 00098 00099 if (*actual_len_bytes > buf_len_bytes) { 00100 esfs_close(&handle); 00101 return SOTP_BUFF_TOO_SMALL; 00102 } 00103 00104 if (*actual_len_bytes) { 00105 esfs_ret = esfs_read(&handle, buf, buf_len_bytes, &act_size); 00106 if (esfs_ret != ESFS_SUCCESS) { 00107 esfs_close(&handle); 00108 return SOTP_READ_ERROR; 00109 } 00110 } 00111 00112 esfs_ret = esfs_close(&handle); 00113 if (esfs_ret != ESFS_SUCCESS) { 00114 return SOTP_OS_ERROR; 00115 } 00116 00117 return SOTP_SUCCESS; 00118 } 00119 00120 sotp_result_e sotp_get_item_size(uint32_t type, uint16_t *actual_len_bytes) 00121 { 00122 esfs_file_t handle; 00123 uint16_t mode; 00124 char file_name[FILE_NAME_SIZE]; 00125 esfs_result_e esfs_ret; 00126 size_t size_bytes; 00127 sotp_result_e ret; 00128 00129 if (!init_done) { 00130 ret = sotp_init(); 00131 if (ret != SOTP_SUCCESS) 00132 return ret; 00133 } 00134 00135 if (type > SOTP_MAX_TYPES) { 00136 return SOTP_BAD_VALUE; 00137 } 00138 00139 memset(&handle, 0, sizeof(handle)); 00140 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00141 00142 esfs_ret = esfs_open((uint8_t *)file_name, strlen(file_name), &mode, &handle); 00143 if (esfs_ret == ESFS_NOT_EXISTS) { 00144 return SOTP_NOT_FOUND; 00145 } 00146 if (esfs_ret != ESFS_SUCCESS) { 00147 return SOTP_OS_ERROR; 00148 } 00149 00150 esfs_ret = esfs_file_size(&handle, &size_bytes); 00151 if (esfs_ret != ESFS_SUCCESS) { 00152 esfs_close(&handle); 00153 return SOTP_READ_ERROR; 00154 } 00155 00156 esfs_ret = esfs_close(&handle); 00157 if (esfs_ret != ESFS_SUCCESS) { 00158 return SOTP_OS_ERROR; 00159 } 00160 00161 *actual_len_bytes = (uint16_t) size_bytes; 00162 return SOTP_SUCCESS; 00163 } 00164 00165 sotp_result_e sotp_set(uint32_t type, uint16_t buf_len_bytes, const uint32_t *buf) 00166 { 00167 esfs_file_t handle; 00168 uint16_t mode; 00169 char file_name[FILE_NAME_SIZE]; 00170 esfs_result_e esfs_ret; 00171 sotp_result_e ret; 00172 00173 if (!init_done) { 00174 ret = sotp_init(); 00175 if (ret != SOTP_SUCCESS) 00176 return ret; 00177 } 00178 00179 if (type > SOTP_MAX_TYPES) { 00180 return SOTP_BAD_VALUE; 00181 } 00182 00183 // Only perform actual setting of values on OTP types. Return success for the rest without 00184 // doing anything. 00185 if (!sotp_is_otp_type(type)) { 00186 return SOTP_SUCCESS; 00187 } 00188 00189 memset(&handle, 0, sizeof(handle)); 00190 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00191 00192 esfs_ret = esfs_open((uint8_t *)file_name, strlen(file_name), &mode, &handle); 00193 if (esfs_ret == ESFS_SUCCESS) { 00194 esfs_close(&handle); 00195 return SOTP_ALREADY_EXISTS; 00196 } 00197 if (esfs_ret != ESFS_NOT_EXISTS) { 00198 return SOTP_OS_ERROR; 00199 } 00200 00201 esfs_ret = esfs_create((uint8_t *)file_name, strlen(file_name), NULL, 0, ESFS_FACTORY_VAL, &handle); 00202 if (esfs_ret != ESFS_SUCCESS) { 00203 return SOTP_OS_ERROR; 00204 } 00205 00206 if (buf && buf_len_bytes) { 00207 esfs_ret = esfs_write(&handle, buf, buf_len_bytes); 00208 if (esfs_ret != ESFS_SUCCESS) { 00209 esfs_close(&handle); 00210 return SOTP_WRITE_ERROR; 00211 } 00212 } 00213 00214 esfs_ret = esfs_close(&handle); 00215 if (esfs_ret != ESFS_SUCCESS) { 00216 return SOTP_OS_ERROR; 00217 } 00218 00219 return SOTP_SUCCESS; 00220 } 00221 00222 #ifdef SOTP_TESTING 00223 00224 sotp_result_e sotp_set_for_testing(uint32_t type, uint16_t buf_len_bytes, const uint32_t *buf) 00225 { 00226 char file_name[FILE_NAME_SIZE]; 00227 esfs_result_e esfs_ret; 00228 sotp_result_e ret; 00229 00230 if (!init_done) { 00231 ret = sotp_init(); 00232 if (ret != SOTP_SUCCESS) 00233 return ret; 00234 } 00235 00236 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00237 esfs_ret = esfs_delete((uint8_t *)file_name, strlen(file_name)); 00238 if ((esfs_ret != ESFS_NOT_EXISTS) && (esfs_ret != ESFS_SUCCESS)) { 00239 return SOTP_OS_ERROR; 00240 } 00241 return sotp_set(type, buf_len_bytes, buf); 00242 } 00243 00244 sotp_result_e sotp_delete(uint32_t type) 00245 { 00246 char file_name[FILE_NAME_SIZE]; 00247 esfs_result_e esfs_ret; 00248 sotp_result_e ret; 00249 00250 if (!init_done) { 00251 ret = sotp_init(); 00252 if (ret != SOTP_SUCCESS) 00253 return ret; 00254 } 00255 00256 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00257 esfs_ret = esfs_delete((uint8_t *)file_name, strlen(file_name)); 00258 if (esfs_ret == ESFS_SUCCESS) 00259 return SOTP_SUCCESS; 00260 00261 if (esfs_ret == ESFS_NOT_EXISTS) 00262 return SOTP_NOT_FOUND; 00263 00264 return SOTP_OS_ERROR; 00265 } 00266 00267 #endif 00268 00269 sotp_result_e sotp_init(void) 00270 { 00271 esfs_result_e esfs_ret; 00272 00273 if (init_done) 00274 return SOTP_SUCCESS; 00275 00276 esfs_ret = esfs_init(); 00277 if (esfs_ret != ESFS_SUCCESS) { 00278 return SOTP_OS_ERROR; 00279 } 00280 00281 return SOTP_SUCCESS; 00282 } 00283 00284 sotp_result_e sotp_deinit(void) 00285 { 00286 return SOTP_SUCCESS; 00287 } 00288 00289 sotp_result_e sotp_reset(void) 00290 { 00291 char file_name[FILE_NAME_SIZE]; 00292 esfs_result_e esfs_ret; 00293 uint32_t type; 00294 sotp_result_e ret; 00295 00296 if (!init_done) { 00297 ret = sotp_init(); 00298 if (ret != SOTP_SUCCESS) 00299 return ret; 00300 } 00301 00302 for (type = 0; type < SOTP_MAX_TYPES; type++) { 00303 sprintf(file_name, "%s%ld", FILE_NAME_BASE, type); 00304 00305 esfs_ret = esfs_delete((uint8_t *)file_name, strlen(file_name)); 00306 if ((esfs_ret != ESFS_NOT_EXISTS) && (esfs_ret != ESFS_SUCCESS)) { 00307 return SOTP_OS_ERROR; 00308 } 00309 } 00310 00311 return SOTP_SUCCESS; 00312 } 00313 00314 #ifdef SOTP_TESTING 00315 00316 sotp_result_e sotp_force_garbage_collection(void) 00317 { 00318 return SOTP_SUCCESS; 00319 } 00320 00321 #endif 00322 00323 #endif
Generated on Tue Jul 12 2022 19:01:37 by
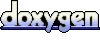