Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
pal_update.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include <stdlib.h> 00018 #include <stdio.h> 00019 #include <pal.h> 00020 #include "pal_plat_update.h" 00021 #include "pal_update.h" 00022 #include "pal_macros.h" 00023 00024 PAL_PRIVATE uint8_t palUpdateInitFlag = 0; 00025 00026 #define PAL_KILOBYTE 1024 00027 00028 #ifndef PAL_UPDATE_IMAGE_LOCATION 00029 #error "Please definee PAL_UPDATE_IMAGE_LOCATION to UPDATE_USE_FLASH (value 1) or UPDATE_USE_FS(2)" 00030 #endif 00031 00032 #if (PAL_UPDATE_IMAGE_LOCATION == PAL_UPDATE_USE_FS) 00033 #define SEEK_POS_INVALID 0xFFFFFFFF 00034 PAL_PRIVATE FirmwareHeader_t pal_pi_mbed_firmware_header; 00035 PAL_PRIVATE palImageSignalEvent_t g_palUpdateServiceCBfunc; 00036 PAL_PRIVATE palFileDescriptor_t image_file[IMAGE_COUNT_MAX]; 00037 PAL_PRIVATE bool last_read_nwrite[IMAGE_COUNT_MAX]; 00038 PAL_PRIVATE uint32_t last_seek_pos[IMAGE_COUNT_MAX]; 00039 PAL_PRIVATE bool valid_index(uint32_t index); 00040 PAL_PRIVATE size_t safe_read(uint32_t index, size_t offset, uint8_t *buffer, uint32_t size); 00041 PAL_PRIVATE size_t safe_write(uint32_t index, size_t offset, const uint8_t *buffer, uint32_t size); 00042 PAL_PRIVATE bool open_if_necessary(uint32_t index, bool read_nwrite); 00043 PAL_PRIVATE bool seek_if_necessary(uint32_t index, size_t offset, bool read_nwrite); 00044 PAL_PRIVATE bool close_if_necessary(uint32_t index); 00045 PAL_PRIVATE const char *image_path_alloc_from_index(uint32_t index); 00046 PAL_PRIVATE const char *header_path_alloc_from_index(uint32_t index); 00047 PAL_PRIVATE const char *path_join_and_alloc(const char * const * path_list); 00048 00049 PAL_PRIVATE palStatus_t pal_set_fw_header(palImageId_t index, FirmwareHeader_t *headerP); 00050 PAL_PRIVATE uint32_t internal_crc32(const uint8_t* buffer, uint32_t length); 00051 00052 00053 char* pal_imageGetFolder(void) 00054 { 00055 return PAL_UPDATE_FIRMWARE_DIR; 00056 } 00057 00058 00059 palStatus_t pal_imageInitAPI(palImageSignalEvent_t CBfunction) 00060 { 00061 palStatus_t status = PAL_SUCCESS; 00062 //printf("pal_imageInitAPI\r\n"); 00063 PAL_MODULE_INIT(palUpdateInitFlag); 00064 00065 // create absolute path. 00066 00067 00068 pal_fsMkDir(PAL_UPDATE_FIRMWARE_DIR); 00069 00070 g_palUpdateServiceCBfunc = CBfunction; 00071 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_INIT); 00072 return status; 00073 } 00074 00075 palStatus_t pal_imageDeInit(void) 00076 { 00077 //printf("pal_plat_imageDeInit\r\n"); 00078 PAL_MODULE_DEINIT(palUpdateInitFlag); 00079 00080 for (int i = 0; i < IMAGE_COUNT_MAX; i++) 00081 { 00082 close_if_necessary(i); 00083 } 00084 00085 return PAL_SUCCESS; 00086 } 00087 00088 palStatus_t pal_imagePrepare(palImageId_t imageId, palImageHeaderDeails_t *headerDetails) 00089 { 00090 //printf("pal_imagePrepare(imageId=%lu, size=%lu)\r\n", imageId, headerDetails->imageSize); 00091 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00092 palStatus_t ret; 00093 uint8_t *buffer; 00094 00095 // write the image header to file system 00096 memset(&pal_pi_mbed_firmware_header,0,sizeof(pal_pi_mbed_firmware_header)); 00097 pal_pi_mbed_firmware_header.totalSize = headerDetails->imageSize; 00098 pal_pi_mbed_firmware_header.magic = FIRMWARE_HEADER_MAGIC; 00099 pal_pi_mbed_firmware_header.version = FIRMWARE_HEADER_VERSION; 00100 pal_pi_mbed_firmware_header.firmwareVersion = headerDetails->version; 00101 memcpy(pal_pi_mbed_firmware_header.firmwareSHA256,headerDetails->hash.buffer,SIZEOF_SHA256); 00102 00103 pal_pi_mbed_firmware_header.checksum = internal_crc32((uint8_t *) &pal_pi_mbed_firmware_header, 00104 sizeof(pal_pi_mbed_firmware_header)); 00105 00106 ret = pal_set_fw_header(imageId, &pal_pi_mbed_firmware_header); 00107 00108 /*Check that the size of the image is valid and reserve space for it*/ 00109 if (ret == PAL_SUCCESS) 00110 { 00111 buffer = malloc(PAL_KILOBYTE); 00112 if (NULL != buffer) 00113 { 00114 uint32_t writeCounter = 0; 00115 memset(buffer,0,PAL_KILOBYTE); 00116 while(writeCounter <= headerDetails->imageSize) 00117 { 00118 int written = safe_write(imageId,0,buffer,PAL_KILOBYTE); 00119 writeCounter+=PAL_KILOBYTE; 00120 if (PAL_KILOBYTE != written) 00121 { 00122 ret = PAL_ERR_UPDATE_ERROR ; 00123 } 00124 } 00125 if ((PAL_SUCCESS == ret) && (writeCounter < headerDetails->imageSize)) 00126 { 00127 //writing the last bytes 00128 int written = safe_write(imageId,0,buffer,(headerDetails->imageSize - writeCounter)); 00129 if ((headerDetails->imageSize - writeCounter) != written) 00130 { 00131 ret = PAL_ERR_UPDATE_ERROR ; 00132 } 00133 } 00134 free(buffer); 00135 if (PAL_SUCCESS == ret) 00136 { 00137 ret = pal_fsFseek(&(image_file[imageId]),0,PAL_FS_OFFSET_SEEKSET); 00138 } 00139 else 00140 { 00141 pal_fsUnlink(image_path_alloc_from_index(imageId)); 00142 } 00143 } 00144 else 00145 { 00146 ret = PAL_ERR_NO_MEMORY ; 00147 } 00148 } 00149 if (PAL_SUCCESS == ret) 00150 { 00151 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_PREPARE); 00152 } 00153 else 00154 { 00155 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00156 } 00157 00158 00159 return ret; 00160 } 00161 00162 palStatus_t pal_imageWrite(palImageId_t imageId, size_t offset, palConstBuffer_t *chunk) 00163 { 00164 //printf("pal_imageWrite(imageId=%lu, offset=%lu)\r\n", imageId, offset); 00165 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00166 palStatus_t ret = PAL_ERR_UPDATE_ERROR ; 00167 00168 int xfer_size_or_error = safe_write(imageId, offset, chunk->buffer, chunk->bufferLength); 00169 if ((xfer_size_or_error < 0) || ((uint32_t)xfer_size_or_error != chunk->bufferLength)) 00170 { 00171 //printf("Error writing to file\r\n"); 00172 } 00173 else 00174 { 00175 ret = PAL_SUCCESS; 00176 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_WRITE); 00177 } 00178 00179 return ret; 00180 } 00181 00182 palStatus_t pal_imageFinalize(palImageId_t imageId) 00183 { 00184 //printf("pal_imageFinalize(id=%i)\r\n", imageId); 00185 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00186 palStatus_t ret = PAL_ERR_UPDATE_ERROR ; 00187 00188 if (close_if_necessary(imageId)) 00189 { 00190 ret = PAL_SUCCESS; 00191 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_FINALIZE); 00192 } 00193 00194 return ret; 00195 } 00196 00197 palStatus_t pal_imageGetDirectMemoryAccess(palImageId_t imageId, void** imagePtr, size_t* imageSizeInBytes) 00198 { 00199 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00200 palStatus_t status = PAL_SUCCESS; 00201 status = pal_plat_imageGetDirectMemAccess (imageId, imagePtr, imageSizeInBytes); 00202 return status; 00203 } 00204 00205 palStatus_t pal_imageReadToBuffer(palImageId_t imageId, size_t offset, palBuffer_t *chunk) 00206 { 00207 //printf("pal_imageReadToBuffer(imageId=%lu, offset=%lu)\r\n", imageId, offset); 00208 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00209 palStatus_t ret = PAL_ERR_UPDATE_ERROR ; 00210 00211 int xfer_size_or_error = safe_read(imageId, offset, chunk->buffer, chunk->maxBufferLength); 00212 if (xfer_size_or_error < 0) 00213 { 00214 //printf("Error reading from file\r\n"); 00215 } 00216 else 00217 { 00218 chunk->bufferLength = xfer_size_or_error; 00219 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_READTOBUFFER); 00220 ret = PAL_SUCCESS; 00221 } 00222 00223 return ret; 00224 } 00225 00226 palStatus_t pal_imageActivate(palImageId_t imageId) 00227 { 00228 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00229 palStatus_t status = PAL_SUCCESS; 00230 status = pal_plat_imageActivate (imageId); 00231 return status; 00232 } 00233 00234 palStatus_t pal_imageGetFirmwareHeaderData(palImageId_t imageId, palBuffer_t *headerData) 00235 { 00236 palStatus_t ret = PAL_SUCCESS; 00237 palFileDescriptor_t file = 0; 00238 size_t xfer_size; 00239 if (NULL == headerData) 00240 { 00241 return PAL_ERR_NULL_POINTER ; 00242 } 00243 if (headerData->maxBufferLength < sizeof(palFirmwareHeader_t)) 00244 { 00245 PAL_LOG(ERR, "Firmware header buffer size is too small(is %" PRIu32 " needs to be at least %zu)\r\n" 00246 ,headerData->maxBufferLength, sizeof(palFirmwareHeader_t)); 00247 return PAL_ERR_INVALID_ARGUMENT ; 00248 } 00249 00250 const char *file_path = header_path_alloc_from_index(imageId); 00251 if (file_path) 00252 { 00253 ret = pal_fsFopen(file_path, PAL_FS_FLAG_READONLY, &file); 00254 if (ret == PAL_SUCCESS) 00255 { 00256 ret = pal_fsFread(&file, headerData->buffer, sizeof(palFirmwareHeader_t), &xfer_size); 00257 if (PAL_SUCCESS == ret) 00258 { 00259 headerData->bufferLength = xfer_size; 00260 } 00261 pal_fsFclose(&file); 00262 } 00263 free((void*)file_path); 00264 } 00265 else 00266 { 00267 ret = PAL_ERR_NO_MEMORY ; 00268 } 00269 return ret; 00270 } 00271 00272 palStatus_t pal_imageGetActiveHash(palBuffer_t *hash) 00273 { 00274 //printf("pal_imageGetActiveHash\r\n"); 00275 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00276 palStatus_t ret; 00277 00278 if (hash->maxBufferLength < SIZEOF_SHA256) 00279 { 00280 ret = PAL_ERR_BUFFER_TOO_SMALL ; 00281 goto exit; 00282 } 00283 00284 hash->bufferLength = 0; 00285 memset(hash->buffer, 0, hash->maxBufferLength); 00286 00287 ret = pal_plat_imageGetActiveHash (hash); 00288 if (ret == PAL_SUCCESS) 00289 { 00290 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_GETACTIVEHASH); 00291 } 00292 00293 exit: 00294 return ret; 00295 } 00296 00297 palStatus_t pal_imageGetActiveVersion(palBuffer_t *version) 00298 { 00299 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00300 palStatus_t status = PAL_SUCCESS; 00301 status = pal_plat_imageGetActiveVersion (version); 00302 return status; 00303 } 00304 00305 palStatus_t pal_imageWriteDataToMemory(palImagePlatformData_t dataId, const palConstBuffer_t * const dataBuffer) 00306 { 00307 palStatus_t status = PAL_SUCCESS; 00308 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00309 // this switch is for further use when there will be more options 00310 switch(dataId) 00311 { 00312 case PAL_IMAGE_DATA_HASH: 00313 status = pal_plat_imageWriteHashToMemory (dataBuffer); 00314 break; 00315 default: 00316 { 00317 PAL_LOG(ERR, "Update image write to memory error"); 00318 status = PAL_ERR_GENERIC_FAILURE; 00319 } 00320 } 00321 return status; 00322 } 00323 00324 PAL_PRIVATE palStatus_t pal_set_fw_header(palImageId_t index, FirmwareHeader_t *headerP) 00325 { 00326 palStatus_t ret; 00327 palFileDescriptor_t file = 0; 00328 size_t xfer_size; 00329 00330 const char *file_path = header_path_alloc_from_index(index); 00331 ret = pal_fsFopen(file_path, PAL_FS_FLAG_READWRITETRUNC, &file); 00332 if (ret != PAL_SUCCESS) 00333 { 00334 //printf("pal_fsFopen returned 0x%x\r\n", ret); 00335 goto exit; 00336 } 00337 00338 ret = pal_fsFwrite(&file, headerP, sizeof(FirmwareHeader_t), &xfer_size); 00339 if (ret != PAL_SUCCESS) 00340 { 00341 //printf("pal_fsFread returned 0x%x\r\n", ret); 00342 goto exit; 00343 } 00344 else if (xfer_size != sizeof(FirmwareHeader_t)) 00345 { 00346 //printf("Size written %lu expected %lu\r\n", xfer_size, sizeof(FirmwareHeader_t)); 00347 goto exit; 00348 } 00349 00350 ret = PAL_SUCCESS; 00351 00352 exit: 00353 if (file != 0) 00354 { 00355 ret = pal_fsFclose(&file); 00356 if (ret != PAL_SUCCESS) 00357 { 00358 //printf("Error closing file %s\r\n", file_path); 00359 ret = PAL_ERR_UPDATE_ERROR ; 00360 } 00361 } 00362 free((void*)file_path); 00363 00364 return ret; 00365 } 00366 00367 /** 00368 * @brief Bitwise CRC32 calculation 00369 * @details Modified from ARM Keil code: 00370 * http://www.keil.com/appnotes/docs/apnt_277.asp 00371 * 00372 * @param buffer Input byte array. 00373 * @param length Number of bytes in array. 00374 * 00375 * @return CRC32 00376 */ 00377 PAL_PRIVATE uint32_t internal_crc32(const uint8_t* buffer, 00378 uint32_t length) 00379 { 00380 const uint8_t* current = buffer; 00381 uint32_t crc = 0xFFFFFFFF; 00382 00383 while (length--) 00384 { 00385 crc ^= *current++; 00386 00387 for (uint32_t counter = 0; counter < 8; counter++) 00388 { 00389 if (crc & 1) 00390 { 00391 crc = (crc >> 1) ^ 0xEDB88320; 00392 } 00393 else 00394 { 00395 crc = crc >> 1; 00396 } 00397 } 00398 } 00399 00400 return (crc ^ 0xFFFFFFFF); 00401 } 00402 00403 PAL_PRIVATE bool valid_index(uint32_t index) 00404 { 00405 return (index < IMAGE_COUNT_MAX); 00406 } 00407 00408 PAL_PRIVATE size_t safe_read(uint32_t index, size_t offset, uint8_t *buffer, uint32_t size) 00409 { 00410 const bool read_nwrite = true; 00411 size_t xfer_size = 0; 00412 palStatus_t status; 00413 00414 if ((!valid_index(index)) || (!open_if_necessary(index, read_nwrite)) || (!seek_if_necessary(index, offset, read_nwrite))) 00415 { 00416 return 0; 00417 } 00418 00419 status = pal_fsFread(&(image_file[index]), buffer, size, &xfer_size); 00420 if (status == PAL_SUCCESS) 00421 { 00422 last_read_nwrite[index] = read_nwrite; 00423 last_seek_pos[index] += xfer_size; 00424 } 00425 00426 return xfer_size; 00427 } 00428 00429 PAL_PRIVATE size_t safe_write(uint32_t index, size_t offset, const uint8_t *buffer, uint32_t size) 00430 { 00431 const bool read_nwrite = false; 00432 size_t xfer_size = 0; 00433 palStatus_t status; 00434 if ((!valid_index(index)) || (!open_if_necessary(index, read_nwrite)) || (!seek_if_necessary(index, offset, read_nwrite))) 00435 { 00436 return 0; 00437 } 00438 status = pal_fsFseek(&(image_file[index]), offset, PAL_FS_OFFSET_SEEKSET); 00439 if (status == PAL_SUCCESS) 00440 { 00441 status = pal_fsFwrite(&(image_file[index]), buffer, size, &xfer_size); 00442 if (status == PAL_SUCCESS) 00443 { 00444 last_read_nwrite[index] = read_nwrite; 00445 last_seek_pos[index] += xfer_size; 00446 00447 if (size != xfer_size) 00448 { 00449 //printf("WRONG SIZE expected %u got %lu\r\n", size, xfer_size); 00450 return 0; 00451 } 00452 00453 } 00454 } 00455 00456 return xfer_size; 00457 } 00458 00459 PAL_PRIVATE bool open_if_necessary(uint32_t index, bool read_nwrite) 00460 { 00461 if (!valid_index(index)) 00462 { 00463 return false; 00464 } 00465 if ( (unsigned int*)image_file[index] == NULL ) 00466 { 00467 const char *file_path = image_path_alloc_from_index(index); 00468 pal_fsFileMode_t mode = read_nwrite ? PAL_FS_FLAG_READWRITE : PAL_FS_FLAG_READWRITETRUNC; 00469 00470 palStatus_t ret = pal_fsFopen(file_path, mode, &(image_file[index])); 00471 free((void*)file_path); 00472 last_seek_pos[index] = 0; 00473 if (ret != PAL_SUCCESS) 00474 { 00475 return false; 00476 } 00477 } 00478 00479 return true; 00480 } 00481 00482 PAL_PRIVATE bool seek_if_necessary(uint32_t index, size_t offset, bool read_nwrite) 00483 { 00484 if (!valid_index(index)) 00485 { 00486 return false; 00487 } 00488 00489 if ((read_nwrite != last_read_nwrite[index]) || 00490 (offset != last_seek_pos[index])) 00491 { 00492 palStatus_t ret = pal_fsFseek(&(image_file[index]), offset, PAL_FS_OFFSET_SEEKSET); 00493 if (ret != PAL_SUCCESS) 00494 { 00495 last_seek_pos[index] = SEEK_POS_INVALID; 00496 return false; 00497 } 00498 } 00499 00500 last_read_nwrite[index] = read_nwrite; 00501 last_seek_pos[index] = offset; 00502 00503 return true; 00504 } 00505 00506 PAL_PRIVATE bool close_if_necessary(uint32_t index) 00507 { 00508 if (!valid_index(index)) 00509 { 00510 return false; 00511 } 00512 00513 palFileDescriptor_t file = image_file[index]; 00514 image_file[index] = 0; 00515 last_seek_pos[index] = SEEK_POS_INVALID; 00516 00517 if (file != 0) 00518 { 00519 palStatus_t ret = pal_fsFclose(&file); 00520 if (ret != 0) 00521 { 00522 return false; 00523 } 00524 } 00525 00526 return true; 00527 } 00528 00529 PAL_PRIVATE const char *image_path_alloc_from_index(uint32_t index) 00530 { 00531 char file_name[32] = {0}; 00532 snprintf(file_name, sizeof(file_name)-1, "image_%" PRIu32 ".bin", index); 00533 file_name[sizeof(file_name) - 1] = 0; 00534 const char * const path_list[] = { 00535 (char*)PAL_UPDATE_FIRMWARE_DIR, 00536 file_name, 00537 NULL 00538 }; 00539 00540 return path_join_and_alloc(path_list); 00541 } 00542 00543 PAL_PRIVATE const char *header_path_alloc_from_index(uint32_t index) 00544 { 00545 char file_name[32] = {0}; 00546 00547 if (ACTIVE_IMAGE_INDEX == index) 00548 { 00549 snprintf(file_name, sizeof(file_name)-1, "header_active.bin"); 00550 } 00551 else 00552 { 00553 snprintf(file_name, sizeof(file_name)-1, "header_%" PRIu32 ".bin", index); 00554 } 00555 00556 const char * const path_list[] = { 00557 (char*)PAL_UPDATE_FIRMWARE_DIR, 00558 file_name, 00559 NULL 00560 }; 00561 00562 return path_join_and_alloc(path_list); 00563 } 00564 00565 00566 PAL_PRIVATE const char *path_join_and_alloc(const char * const * path_list) 00567 { 00568 uint32_t string_size = 1; 00569 uint32_t pos = 0; 00570 00571 // Determine size of string to return 00572 while (path_list[pos] != NULL) 00573 { 00574 // Size of string and space for separator 00575 string_size += strlen(path_list[pos]) + 1; 00576 pos++; 00577 } 00578 00579 // Allocate and initialize memory 00580 char *path = (char*)malloc(string_size); 00581 if (NULL != path) 00582 { 00583 memset(path, 0, string_size); 00584 // Write joined path 00585 pos = 0; 00586 while (path_list[pos] != NULL) 00587 { 00588 bool has_slash = '/' == path_list[pos][strlen(path_list[pos]) - 1]; 00589 bool is_last = NULL == path_list[pos + 1]; 00590 strncat(path, path_list[pos], string_size - strlen(path) - 1); 00591 if (!has_slash && !is_last) 00592 { 00593 strncat(path, "/", string_size - strlen(path) - 1); 00594 } 00595 pos++; 00596 } 00597 } 00598 return path; 00599 } 00600 00601 00602 00603 00604 #elif (PAL_UPDATE_IMAGE_LOCATION == PAL_UPDATE_USE_FLASH) 00605 00606 palStatus_t pal_imageInitAPI(palImageSignalEvent_t CBfunction) 00607 { 00608 PAL_MODULE_INIT(palUpdateInitFlag); 00609 palStatus_t status = PAL_SUCCESS; 00610 status = pal_plat_imageInitAPI (CBfunction); 00611 return status; 00612 } 00613 00614 palStatus_t pal_imageDeInit(void) 00615 { 00616 PAL_MODULE_DEINIT(palUpdateInitFlag); 00617 palStatus_t status = PAL_SUCCESS; 00618 status = pal_plat_imageDeInit (); 00619 return status; 00620 } 00621 00622 00623 00624 palStatus_t pal_imagePrepare(palImageId_t imageId, palImageHeaderDeails_t *headerDetails) 00625 { 00626 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00627 palStatus_t status = PAL_SUCCESS; 00628 pal_plat_imageSetHeader (imageId,headerDetails); 00629 status = pal_plat_imageReserveSpace (imageId, headerDetails->imageSize); 00630 00631 return status; 00632 } 00633 00634 palStatus_t pal_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t *chunk) 00635 { 00636 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00637 palStatus_t status = PAL_SUCCESS; 00638 status = pal_plat_imageWrite (imageId, offset, chunk); 00639 return status; 00640 } 00641 00642 palStatus_t pal_imageFinalize(palImageId_t imageId) 00643 { 00644 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00645 palStatus_t status = PAL_SUCCESS; 00646 status = pal_plat_imageFlush (imageId); 00647 return status; 00648 } 00649 00650 palStatus_t pal_imageGetDirectMemoryAccess(palImageId_t imageId, void** imagePtr, size_t* imageSizeInBytes) 00651 { 00652 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00653 palStatus_t status = PAL_SUCCESS; 00654 status = pal_plat_imageGetDirectMemAccess (imageId, imagePtr, imageSizeInBytes); 00655 return status; 00656 } 00657 00658 palStatus_t pal_imageReadToBuffer(palImageId_t imageId, size_t offset, palBuffer_t *chunk) 00659 { 00660 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00661 palStatus_t status = PAL_SUCCESS; 00662 00663 status = pal_plat_imageReadToBuffer (imageId,offset,chunk); 00664 return status; 00665 } 00666 00667 palStatus_t pal_imageActivate(palImageId_t imageId) 00668 { 00669 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00670 palStatus_t status = PAL_SUCCESS; 00671 status = pal_plat_imageActivate (imageId); 00672 return status; 00673 } 00674 00675 palStatus_t pal_imageGetActiveHash(palBuffer_t *hash) 00676 { 00677 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00678 palStatus_t status = PAL_SUCCESS; 00679 status = pal_plat_imageGetActiveHash (hash); 00680 return status; 00681 } 00682 00683 palStatus_t pal_imageGetActiveVersion(palBuffer_t *version) 00684 { 00685 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00686 palStatus_t status = PAL_SUCCESS; 00687 status = pal_plat_imageGetActiveVersion (version); 00688 return status; 00689 } 00690 00691 palStatus_t pal_imageWriteDataToMemory(palImagePlatformData_t dataId, const palConstBuffer_t * const dataBuffer) 00692 { 00693 palStatus_t status = PAL_SUCCESS; 00694 PAL_MODULE_IS_INIT(palUpdateInitFlag); 00695 // this switch is for further use when there will be more options 00696 switch(dataId) 00697 { 00698 case PAL_IMAGE_DATA_HASH: 00699 status = pal_plat_imageWriteHashToMemory (dataBuffer); 00700 break; 00701 default: 00702 { 00703 PAL_LOG(ERR, "Update write data to mem status %d", (int)dataId); 00704 status = PAL_ERR_GENERIC_FAILURE; 00705 } 00706 } 00707 return status; 00708 } 00709 00710 #endif
Generated on Tue Jul 12 2022 19:01:36 by
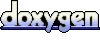